Summary of javascript if condition judgment method_javascript skills
May 16, 2016 pm 04:48 PMConditional statements are used to perform different actions based on different conditions.
Conditional statement
Usually when writing code, you always need to perform different actions for different decisions. You can use conditional statements in your code to accomplish this task.
In JavaScript, we can use the following conditional statements:
•if statement - Use this statement to execute code only when the specified condition is true
•if...else statement - Execute code when the condition is true, and execute other code when the condition is false
•if...else if....else statement - Use this statement to select one of multiple blocks of code to execute
•switch statement - Use this statement to select one of multiple code blocks to execute
If statement
This statement will execute the code only if the specified condition is true.
Grammar
if (condition)
{
only Code executed when the condition is true
}
Note: Please use lowercase if. Using uppercase letters (IF) will generate a JavaScript error!
Example
When the time is less than 20:00, generate a "Good day" greeting:
if (time<20)
{
x="Good day";
}
x is:
Good day
Try it for yourself
Please note that in this syntax, there is no ..else.. . You've told the browser to only execute the code if the specified condition is true.
If...else statement
Please use if....else statement to execute code when the condition is true and other code when the condition is false.
Grammar
if (condition)
{
when Code executed when the condition is true
}
else
{
Code executed when the condition is not true
}
Example
When the time is less than 20:00, you will get the greeting "Good day", otherwise you will get the greeting "Good evening".
if (time<20)
{
x="Good day";
}
else
{
x="Good evening";
}
x is:
Good day
Try it for yourself
If...else if...else statement
Use the if....else if...else statement to select one of multiple blocks of code to execute.
Grammar
if (Condition 1)
{
Code executed when condition 1 is true
}
else if (condition 2)
{
Code executed when condition 2 is true
}
else
{
Code executed when neither condition 1 nor condition 2 is true
}
Instance
If the time is less than 10:00, the greeting "Good morning" will be sent, Otherwise if the time is less than 20:00, send the greeting "Good day", otherwise send the greeting "Good evening":
if (time<10)
{
x="Good morning";
}
else if (time<20)
{
x="Good day";
}
else
{
x="Good evening";
}
x is:
Good morning
In JavaScript, what values can be used as the conditions of if
1. Boolean variable true/false
2. Number is not 0, not NaN/ (0 or NaN)
See the example below. Don’t think that a negative number means the if statement is false.
var i = -1;
if(i ){
alert('here');
}else{
alert('test is ok!');
}
3. The object is not null/(null or undefined)
4. The string is not empty string ("")/empty string ("")
To sum up, for strings, there is no need to write a lot of if (str!=null && str!=undefined && str !=''), just use one sentence
if(!str){
//do something
}
That’s it.
For the non-empty judgment of numbers, you should consider using the isNaN() function. NaN is not equal to any type of data, including itself, and can only be judged with isNaN(). For numeric types, if(a) is false when a in the if(a) statement is 0, and if(a) is true when it is not 0
var b;
var a = 0;
a = a b;
if(a){
alert('1');
}else{
alert('2');
}
if(isNaN (a)){
alert('a is NaN');
}
Javascript tutorial: Methods for optimizing if statements if abbreviation
UglifyJS is a tool for compressing and beautifying JavaScript. In its documentation, I saw several methods for optimizing if statements. Although I haven't used it to do some tentative tests, you can see from here that it does beautify js. Some people may think that the if statement is that simple, to what extent can it be optimized? But take a look at the following ways and you might change your mind.
1. Use common ternary operators
if (foo) bar(); else baz(); ==> foo?bar():baz();
if (!foo) bar(); else baz(); ==> ; foo?baz():bar();
if (foo) return bar(); else return baz(); ==> return foo?bar():baz();
You will definitely be familiar with the above use of ternary operator to optimize if statements, maybe you use it often.
Example given by Script House:
<script>
var i=9
var ii=(i>8)?100:9;
alert(ii);
</script>
Output result:
100
2. Use the and(&&) and or(||) operators
if (foo) bar(); ==> foo&&bar();
if (!foo) bar(); ==> foo||bar();
To be honest, I have never written code like this. I saw this way of writing when I was studying "Brother Niao's Linux Private Cooking", but I didn't expect to implement it in js.
3. Omit the braces {}
if (foo) return bar(); else something(); ==> {if(foo)return bar();something()}
You and I are familiar with this writing method, but I recommend doing this when optimizing the code, or letting UglifyJS help you solve it. After all, if there is one missing curly bracket, the readability of the code is not high.
Writing this, I thought of a method for obtaining HTML element attributes in "Mastering JavaScript" by the father of jQuery.
function getAttr(el, attrName){
var attr = {'for':'htmlFor', 'class':'className'}[attrName] || attrName;
};
If we don’t write it this way, we may need to use two if statements to process it. The above code is not only concise and effective, but also highly readable.
If you think about it carefully, many times we can find effective ways to solve problems, but the key lies in whether we are diligent in finding a better way.
[javascript skills] if(x==null) abbreviation
if(x==null) or if (typeof (x) == 'undefined') can be abbreviated as if(!x), which is not verified.
On the contrary if(x) means x is not empty
Determine whether the object exists
if(document.form1.softurl9){
//Determine whether softurl9 exists to prevent js errors
}
if(document.getElementById("softurl9")){
//Determine whether softurl9 exists to prevent js errors
}

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
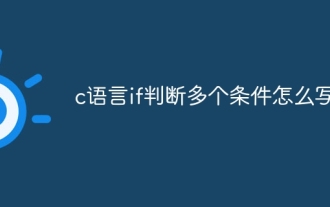
How to write if in c language to judge multiple conditions
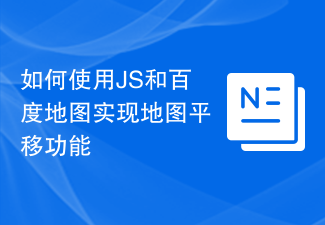
How to use JS and Baidu Maps to implement map pan function
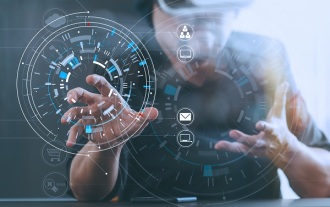
Recommended: Excellent JS open source face detection and recognition project
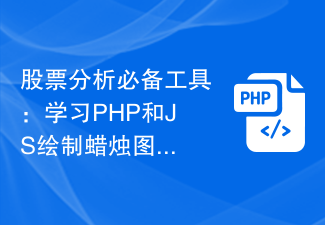
Essential tools for stock analysis: Learn the steps to draw candle charts with PHP and JS
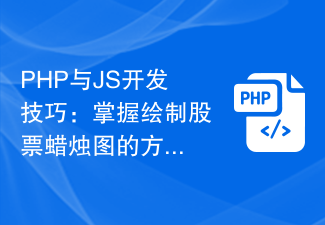
PHP and JS Development Tips: Master the Method of Drawing Stock Candle Charts
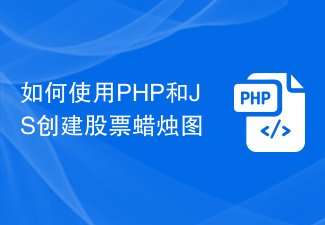
How to create a stock candlestick chart using PHP and JS
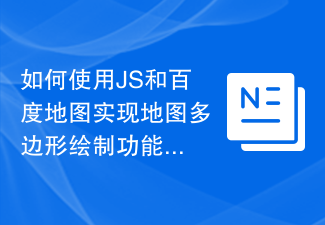
How to use JS and Baidu Maps to implement map polygon drawing function
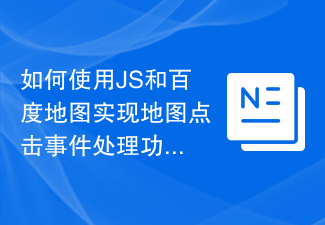
How to use JS and Baidu Map to implement map click event processing function
