


If the title is too long, use javascript to intercept the string by bytes_javascript tips
As a front-end developer, I often encounter in web page display that the title is too long and strings need to be intercepted. If implemented using CSS, there are various compatibility issues and various pitfalls.
Asking the background program to cut it, and making various excuses to ask the background to cut it by bytes is the same as killing the background. In the end, it may only be cut according to the character length, and the final result will not look good. If it’s not correct, it’s better to go back and adjust the CSS and adjust the compatibility;
Front-end students who have the above impressions can silently like it.
I recently came across a project where the backend only provided an interface (json), and the data rendering and data binding of all pages were left to the front end. Finally, regardless of SEO, all the initiative on the page was in my hands, and I inadvertently encountered the old problem of byte interception.
There is a Javascript method to simply obtain the byte length circulating on the Internet:
String.prototype.Blength = function(){//Return the string length in bytes
return this.replace(/([^x00-xFF])/g, "aa"). length;
};
It’s really simple. Characters larger than the ASCII code are counted as two bytes. Although strictly speaking it’s not correct, we use it to assist the display effect. It’s not good if it’s really strict.
But I always feel that it’s just a little opportunistic, and it’s not good to use regular expressions, which are time-consuming. In fact, it only saves two lines of code, so I decided to use the normal method. Calculation:
function getBlength(str){
for (var i=str.length;i--;){
n = str.charCodeAt(i) > 255 ? 2 : 1;
}
return n;
}
I did not extend the method to the prototype of the String object, or because of efficiency issues, the following is the test code:
//Expand to the prototype of String
String.prototype.Blength = function () {
var str = this,
n = 0;
for (var i = str.length; i--; ) {
n = str.charCodeAt(i) > 255 ? 2 : 1;
}
return n ;
}
//Add a method to the String object
String.getBlength = function (str) {
for (var i = str.length, n = 0; i--; ) {
n = str.charCodeAt(i) > 255 ? 2 : 1;
}
return n;
}
//Construct a long mixed Chinese and English string first
var str = "javascript efficient interception of strings by bytes method getBlengthjavascript efficient interception of strings by bytes method getBlength";
str = str.replace(/./g, str).replace(/./g , str);
console.log("The length of the created string is: ",str.length)
console.log("-------------Test begins-- ------------")
console.log("str.Blength() >> ",str.Blength())
console.log("String.getBlength (str) >> ",String.getBlength(str))
console.log("--Efficiency test starts--")
var time1 = new Date()
for (var i=0;i<100;i ){
str.Blength()
}
console.log("Blength takes time: ",new Date() - time1);
var time2 = new Date()
for(var i=0;i<100;i ){
String.getBlength(str)
}
console.log("getBlength consumes When: ",new Date() - time2);
The result is not a little bit inefficient. As for the reason, it may be that time is spent on retrieving the prototype chain. I have not gone into it. I know it. Leave a message and tell me:
The length of the created string is: 314432
-------------Test begins-------------
str.Blength() >> 425408
String.getBlength(str) >> 425408
--Efficiency test starts--
Blength takes time: 1774
getBlength takes time: 95
Now to intercept The basic function of string is now available, because in this case the maximum length of bytes occupied by a character is 2, so it is best to use the binary method to find the appropriate interception position.
Give me an interception function that should be quite efficient:
//Simple calculation of byte length
String.getBlength = function (str) {
for (var i = str.length, n = 0; i--; ) {
n = str.charCodeAt(i) > 255 ? 2 : 1;
}
return n;
}
//Truncate the string according to the specified bytes
String.cutByte = function (str,len,endstr){
var len = len
,endstr = typeof(endstr) == 'undefined' ? "..." : endstr.toString();
function n2(a ){ var n = a / 2 | 0; return (n > 0 ? n : 1)} //Used for binary search
if(!(str "").length || !len || len< ;=0){return "";}
if(this.getBlength(str) <= len){return str;} //The most time-consuming judgment in the entire function, welcome to optimize
var lenS = len - this.getBlength(endstr)
,_lenS = 0
, _strl = 0
while (_strl <= lenS){
var _lenS1 = n2(lenS -_strl)
_strl = this.getBlength(str.substr(_lenS,_lenS1))
_lenS = _lenS1
}
return str.substr(0,_lenS-1) endstr
}
Test the string above. The longer it is, the more time-consuming it is to load. Try cutting it to a length of 20W:
console.log("The length of the created string is: ",str.length," The byte length is: ",String.getBlength(str) )
console.log("-------------Test starts-------------")
console.log("String. cutByte('1 starts with 1',6,'...') >> ",String.cutByte('1 starts with 1',6,'...'))
console.log("String .cutByte(str,12,'...') >> ",String.cutByte(str,12,'...'))
console.log("String.cutByte(str,13, '..') >> ",String.cutByte(str,13,'..'))
console.log("String.cutByte(str,14,'.') >> " ,String.cutByte(str,14,'.'))
console.log("String.cutByte(str,15,'') >> ",String.cutByte(str,15,'') )
console.log("--Efficiency test starts--")
var time1 = new Date()
for(var i=0;i<100;i ){
String. cutByte(str,200000,'...')
}
console.log("Time consuming:",new Date() - time1);
Output result:
The length of the created string is: 314432 The byte length is: 425408
-------------Test begins------------- -
String.cutByte('1 starts with 1',6 ,'...') >> 1 starts 1
String.cutByte(str,12,'...') >> javascrip...
String.cutByte(str,13, '..') >> javascript ..
String.cutByte(str,14,'.') >> javascript high.
String.cutByte(str,15,'') > > javascript high
--Efficiency test starts--
Time consuming: 155
In fact, changing the intercepted character length to 30W and 40W will not be much different, In the face of the binary method, this is all on the same level
Compared with the previous time-consuming calculation of the byte length, using the binary method to search and intercept only consumes less than two byte length calculations.
Finally, students, come and challenge your efficiency!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


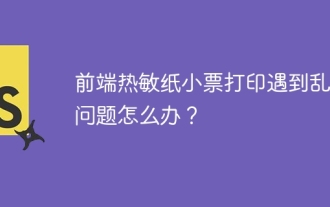
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
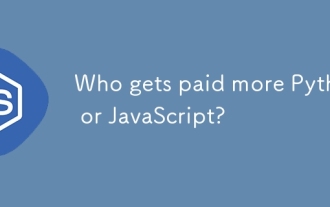
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
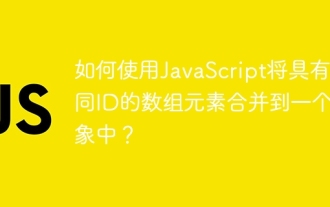
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
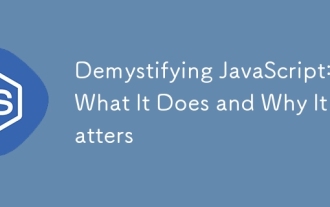
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
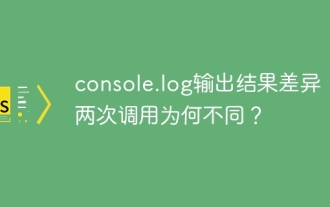
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
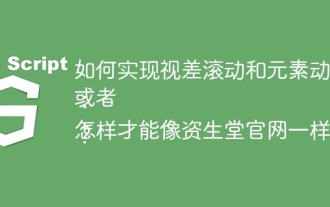
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
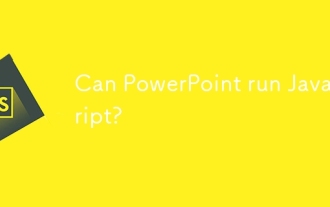
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
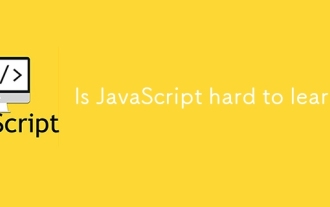
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
