


Using jQuery to asynchronously load JavaScript script solutions_jquery
JavaScript loaders are very powerful and useful tools in web development. Several popular loaders, such as curljs, LABjs and RequireJS, are widely used. They are powerful, but some situations can have simpler solutions.
If you are using jQuery, there is a built-in method for loading scripts. You can use this method if you want to lazy load plugins or any other type of script. Here's how to use it.
Implementation method
jQuery has a built-in getScript method to load a script, and there are several ways to handle the returned results. The most basic usage of jQuery.getScript looks like this:
jQuery.getScript("/path/to/myscript.js", function(data, status, jqxhr) {
/*
After the script has been loaded and executed, you can do some processing
*/
});
getScript method returns a jqXHR object, so it can be used like this:
jQuery.getScript("/path/to/myscript.js")
.done(function() {
/* Processing after successful execution*/
})
.fail(function() {
/* Processing after failed execution*/
});
The most common scenario for using jQuery.getScript is to lazy load a plugin and call it after loading:
jQuery.getScript("jquery.cookie.js")
.done(function() {
jQuery.cookie("cookie_name", "value", { expires : 7 });
});
If you need to do more advanced things like loading multiple scripts and different types of files (text files, images, CSS files, etc.), I recommend you switch to a more powerful JavaScript loader. getScript is perfect if you just want to load the plugin lazily, rather than simply on every page load!
Caching issues
It should be noted that when using jQuery.getScript, a timestamp will be automatically added to the end of the script URL to prevent the script from being cached. Therefore you need to set up the cache script for all requests:
jQuery.ajaxSetup({
cache: true
});
If you don't want to overwrite all the cache with your AJAX request, it's better to use jQuery.ajax method and put dataType Set to script, for example:
jQuery.ajax({
url: "jquery.cookie.js",
dataType: "script",
cache: true
}).done(function() {
jQuery.cookie("cookie_name" , "value", { expires: 7 });
});
You need to pay special attention to caching issues when loading scripts!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
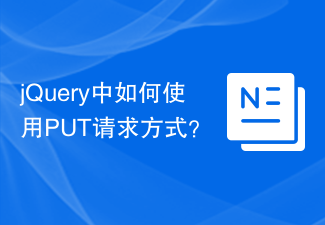
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
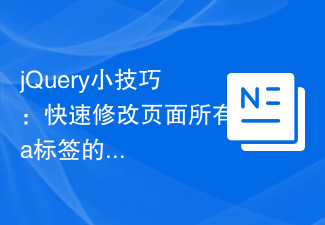
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
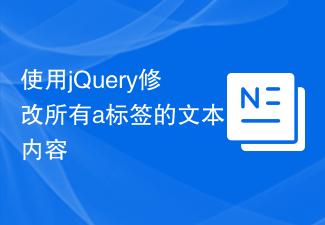
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
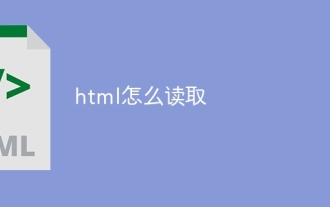
Although HTML itself cannot read files, file reading can be achieved through the following methods: using JavaScript (XMLHttpRequest, fetch()); using server-side languages (PHP, Node.js); using third-party libraries (jQuery.get() , axios, fs-extra).
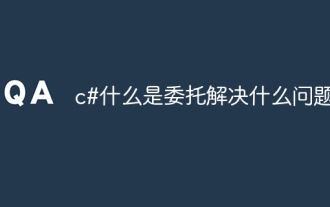
Delegation is a type-safe reference type used to pass method pointers between objects to solve asynchronous programming and event handling problems: Asynchronous programming: Delegation allows methods to be executed in different threads or processes, improving application responsiveness. Event handling: Delegates simplify event handling, allowing events such as clicks or mouse movements to be created and handled.
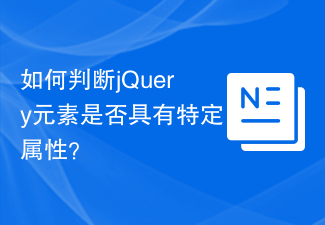
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
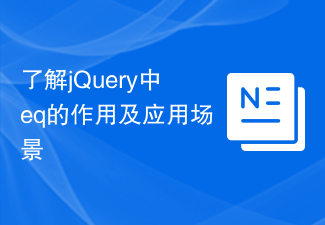
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
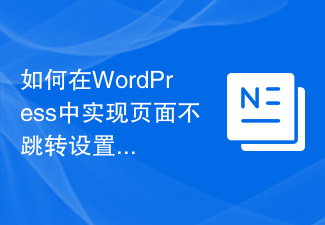
How to prevent page redirection in WordPress? In website development, sometimes we want to implement a page non-jump setting in WordPress, that is, during certain operations, the page content can be updated without refreshing the entire page. This improves user experience and makes the website smoother. Next, we will share how to implement the page non-jump setting in WordPress and provide specific code examples. First, we can use Ajax to prevent the page from jumping. Ajax
