19 guidelines for writing efficient jquery code_jquery
First of all, keep in mind that jQuery is javascript. This means we should adopt the same coding conventions, style guides and best practices.
First of all, if you are a JavaScript novice, I recommend you read "24 Best Practices for JavaScript Beginners". This is a high-quality JavaScript tutorial. It is best to read it before contacting jQuery.
When you are ready to use jQuery, I strongly recommend that you follow these guidelines:
1. Caching variables
DOM traversal is expensive, so try to cache reused elements.
// Oops
h = $('#element').height();
$('#element').css('height',h-20);
// Suggestions
$element = $('#element');
h = $element.height();
$element.css('height',h-20);
2 .Avoid Global Variables
With jQuery and JavaScript, in general, it’s best to make sure your variables are within function scope.
// Oops
$element = $('#element');
h = $element.height();
$element.css('height',h-20);
// Suggestions
var $element = $('#element');
var h = $element.height();
$element.css('height',h-20);
3. Use the Hungarian nomenclature
to prefix the variable with $ to easily identify the jQuery object.
// Oops
var first = $('#first');
var second = $('#second');
var value = $first.val();
// Suggestion - Prefix the jQuery object with $
var $first = $('#first');
var $second = $('#second'),
var value = $first.val();
4 .Use Var chain (single Var mode)
to combine multiple var statements into one statement. I recommend putting unassigned variables at the end.
var
$first = $('#first '),
$second = $('#second'),
value = $first.val(),
k = 3,
cookiestring = 'SOMECOOKIESPLEASE',
i,
j,
myArray = {};
5. Please use 'On'
In the new version of jQuery, the shorter on("click") is used to replace similar click() Such a function. In previous versions on() was bind(). Since jQuery version 1.7, on() is the preferred method of attaching event handlers. However, for consistency's sake, you can simply use the on() method all together.
// Oops
$first.click(function(){
$first.css('border','1px solid red');
$first.css('color','blue');
});
$first.hover(function(){
$first.css('border','1px solid red');
})
// Recommendation
$first.on('click',function(){
$first.css('border','1px solid red');
$first.css(' color','blue');
})
$first.on('hover',function(){
$first.css('border','1px solid red');
})
6. Simplify javascript
In general, it’s best to combine functions whenever possible.
// Oops
$first.click(function(){
$first.css('border','1px solid red');
$first.css('color','blue');
});
// Suggestions
$first.on('click',function(){
$first.css({
'border':'1px solid red',
'color':'blue'
});
});
7. Chain operation
It is very easy for jQuery to implement chain operation of methods. Take advantage of this below.
// Oops
$second.html(value);
$second.on('click',function(){
alert('hello everybody');
});
$second. fadeIn('slow');
$second.animate({height:'120px'},500);
// Suggestions
$second.html(value);
$second.on('click',function(){
alert('hello everybody');
}).fadeIn('slow') .animate({height:'120px'},500);
8. Maintain the readability of the code
Along with streamlining the code and using chaining, it may make the code difficult to read. Adding pinches and line breaks can work wonders.
// Oops
$second.html(value);
$second.on('click',function(){
alert('hello everybody');
}).fadeIn('slow') .animate({height:'120px'},500);
// Suggestions
$second.html(value);
$second
.on('click',function(){ alert('hello everybody');})
.fadeIn('slow')
.animate({height:'120px'},500);
9. Select short-circuit evaluation
Short-circuit evaluation is an expression that is evaluated from left to right, using && ( Logical AND) or || (Logical OR) operator.
// Oops
function initVar($myVar) {
if(!$myVar) {
$myVar = $('#selector');
}
}
// Suggestions
function initVar($myVar) {
$myVar = $myVar || $('#selector');
}
10. Choose one of the shortcuts
to simplify the code One way is to take advantage of coding shortcuts.
// Oops
if(collection.length > 0){..}
// Suggestions
if(collection.length){..}
11. Separate elements during heavy operations
If you plan to do a lot of operations on DOM elements (setting multiple attributes or css styles in succession), It is recommended to detach elements first and then add them.
// Oops
var
$container = $("#container"),
$containerLi = $("#container li"),
$element = null;
$element = $containerLi.first();
//... Many complex operations
// better
var
$container = $("#container"),
$containerLi = $container.find("li"),
$element = null;
$element = $containerLi.first().detach();
//... Many complex operations
$container.append($element);
12. Memorize skills
You may lack experience in using methods in jQuery, be sure to check the documentation, there may be a better or Faster way to use it.
// Oops
$('#id').data(key,value);
// Suggestions (efficient)
$.data('#id',key,value);
13. Cache parent elements using subqueries
As mentioned earlier, DOM traversal is an expensive operation . A typical approach is to cache parent elements and reuse these cached elements when selecting child elements.
// Oops
var
$container = $('#container'),
$containerLi = $('#container li'),
$containerLiSpan = $('#container li span');
// Suggestions (efficient)
var
$container = $('#container '),
$containerLi = $container.find('li'),
$containerLiSpan= $containerLi.find('span');
14. Avoid universal selectors
Putting universal selectors into descendant selectors has very poor performance.
// Oops
$('.container > *');
// Suggestions
$('.container').children();
Avoid implicit universal selectors
Universal selectors are sometimes implicit and difficult to find.
// Oops
$('.someclass :radio');
// Suggestions
$('.someclass input:radio');
Optimize selectors
For example, the Id selector should be unique, so there is no need to add additional selectors.
// Oops
$('div#myid');
$('div#footer a.myLink');
// Recommendation
$('#myid');
$('#footer .myLink');
15. Avoid multiple ID selectors
emphasize here , the ID selector should be unique, there is no need to add additional selectors, and there is no need for multiple descendant ID selectors.
// Oops
$('#outer #inner');
// Suggestions
$('#inner');
16. Stick to the latest version
Newer versions are usually better: more lightweight and more efficient. Obviously, you need to consider the compatibility of the code you want to support. For example, version 2.0 does not support IE 6/7/8.
Abandon deprecated methods
It is very important to pay attention to deprecated methods with each new version and try to avoid using these methods.
// Oops - live has been abandoned
$('#stuff').live('click', function() {
console.log('hooray');
});
// Suggestion
$('#stuff').on('click', function() {
console.log('hooray');
});
// Note: This may be inappropriate. Live can achieve real-time binding, and delegate may be more appropriate
17. Use CDN
Google's CND can ensure that the cache closest to the user is selected and responds quickly. (Please search the address yourself when using Google CND. The address here cannot be used. We recommend the CDN provided by jquery official website).
18. Combine jQuery and javascript native code when necessary
As mentioned above, jQuery is javascript, which means that anything you can do with jQuery can also be done with native code. Native code (or vanilla) may not be as readable and maintainable as jQuery, and the code is longer. But it also means more efficient (usually the closer to the underlying code the less readable the higher the performance, for example: assembly, which of course requires more powerful people). Remember that no framework can be smaller, lighter, and more efficient than native code
Given the performance differences between vanilla and jQuery, I strongly recommend absorbing the best of both worlds and using (if possible) native code equivalent to jQuery.
19. Final advice
Finally, the purpose of recording this article is to improve the performance of jQuery and some other good suggestions. If you want to delve deeper into this topic you will find a lot of fun. Remember, jQuery is not required, just an option. Think about why you want to use it. DOM manipulation? ajax? stencil? css animation? Or a selector engine? Perhaps a javascript microframework or a customized version of jQuery would be a better choice.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
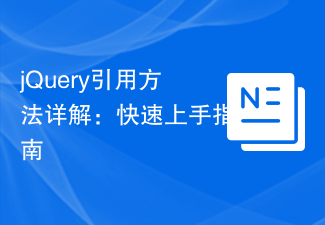
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
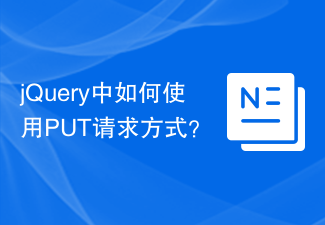
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
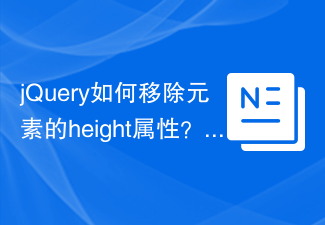
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
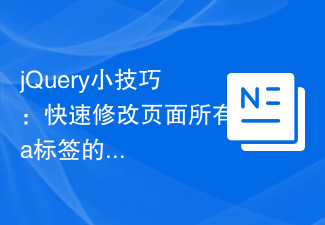
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
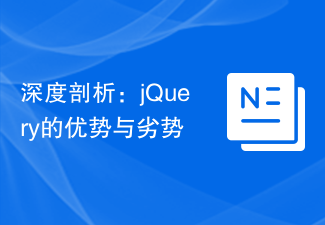
jQuery is a fast, small, feature-rich JavaScript library widely used in front-end development. Since its release in 2006, jQuery has become one of the tools of choice for many developers, but in practical applications, it also has some advantages and disadvantages. This article will deeply analyze the advantages and disadvantages of jQuery and illustrate it with specific code examples. Advantages: 1. Concise syntax jQuery's syntax design is concise and clear, which can greatly improve the readability and writing efficiency of the code. for example,
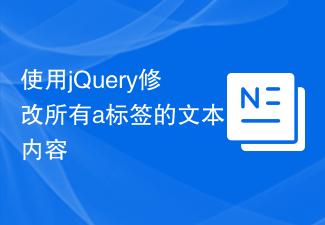
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
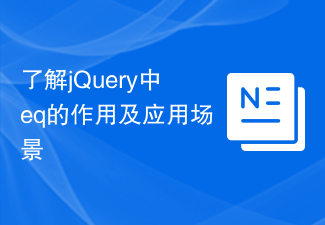
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
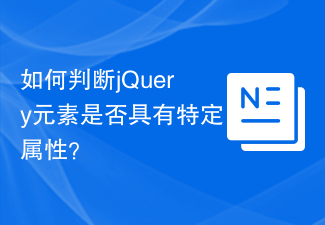
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
