


Summary of three methods for obtaining control values in Jquery_jquery
May 16, 2016 pm 05:00 PMA Jquery method to obtain the server control value
Since the server control will randomly generate the client id after the ASP.NET web page is run, jquery is not easy to operate when obtaining it. , Googled it, and found the following three methods:
Server control code: <asp:TextBox ID="txtUserID" runat="server"></asp:TextBox>
1. $("#<%=txtUserID.ClientID%>").val();
2. $("input[id*=txtUserID]").val();
3. $("*[id$=txtUserID]").val();
Two Jquery methods to obtain the control value
Value:
$("") is a jquery object, not a dom element
value is an attribute of dom element
jquery corresponds to val
val(): Get the first matching element current value.
val(val): Set the value of each matching element.
So, the code should be written like this:
Value: val = $("#id")[0].value;
Assignment:
$("#id ")[0].value = "new value";
or $("#id").val("new value");
Or this can also be done: val = $("#id").attr("value");
Get the value of a set of radio selected items
var item = $('input[@name=items][@checked]').val();
Get the text of the selected item in select
var item = $("select[@name =items] option[@selected]").text();
The second element of the select drop-down box is the currently selected value
$('#select_id')[0].selectedIndex = 1;
The second element of the radio radio group is the currently selected value
$('input[@name=items]').get(1).checked = true;
Get value:
Text box, text area: $("#txt").attr("value");
Multiple selection box checkbox: $("#checkbox_id").attr("value");
Radio group radio: $("input[@type=radio][@checked]").val();
Drop-down box select: $('#sel').val();
Control form elements:
Text box, text area: $("#txt").attr("value",'');//Clear content
$(" #txt").attr("value",'11');//Fill content
Multiple selection box checkbox: $("#chk1").attr("checked",'');//Unchecked
$("#chk2").attr( "checked",true);//Check
if($("#chk1").attr('checked')==undefined) //Judge whether it has been checked
Radio group radio: $("input[@type=radio]").attr("checked",'2');//Set the item with value=2 as the currently selected item
Drop-down box select: $("#sel").attr("value",'-sel3');//Set the item with value=-sel3 as the currently selected item
$ ("<option value='1'>1111</option><option value='2'>2222</option>").appendTo("#sel")//Add the option of the drop-down box
$("#sel").empty(); //Clear the drop-down box
Three Jquery methods to get the DropDownList value of the control
<script type="text/javascript">
function bbOK()
{
var a = $("#ddlGuo option:selected").val();
var b = $("#ddlGuo option:selected").text();
$("#txttext").attr("value", b);
$("#txtval").attr("value", a);
}
</script>
<html>
<asp:DropDownList ID="ddlGuo" runat="server" >
<asp:ListItem Selected="True" Value="001">北京市</asp:ListItem>
<asp:ListItem Value="301">南京</asp:ListItem>
<asp:ListItem Value="313">苏州</asp:ListItem>
</asp:DropDownList>
<asp:TextBox ID="txtval" runat="server"></asp:TextBox>
<asp:TextBox ID="txttext" runat="server"></asp:TextBox>
<br />
<asp:Button ID="Button1" runat="server" Text="点击Select" OnClientClick="bbOK();" />
</html>

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
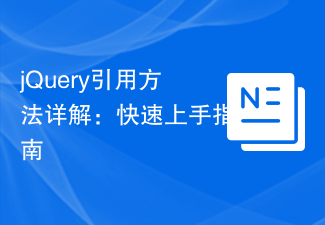
Detailed explanation of jQuery reference methods: Quick start guide
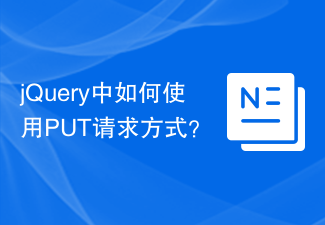
How to use PUT request method in jQuery?
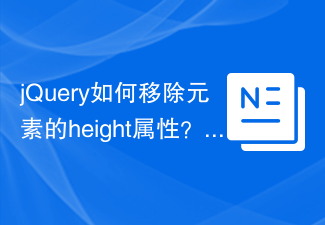
How to remove the height attribute of an element with jQuery?
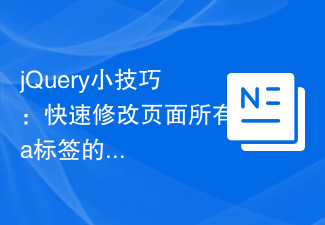
jQuery Tips: Quickly modify the text of all a tags on the page
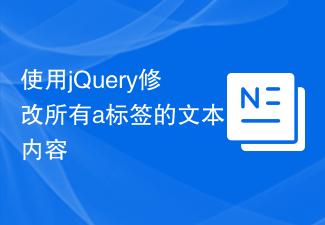
Use jQuery to modify the text content of all a tags
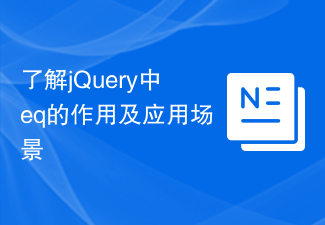
Understand the role and application scenarios of eq in jQuery
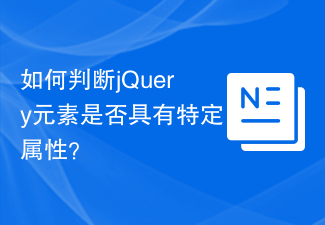
How to tell if a jQuery element has a specific attribute?
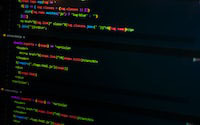
Summary of commonly used file operation functions in PHP
