


js sort Usage summary of two-dimensional array sorting_javascript skills
I have recently been working on the issue of js sorting, because database sorting consumes too many resources. If sorting can be transferred to the client, it can greatly reduce server memory consumption. As for the client, in addition to js, it is as. Unfortunately, I am too bad at as, so I can only choose js to study. . . After my testing, the js built-in method sort is very efficient
We know that the sort function is provided by default in js, but by default this function sorts the array contents in ascending order according to the ASCII code. What if we want to sort a two-dimensional array? There is a multi_sort function that can be called in php, but there seems to be no such function in js, but it doesn't matter, because the sort function of js actually also provides parameters to define a comparison function to sort two-dimensional arrays.
1. Sort by value
Suppose there is the following array
var arr = [[1, 2, 3], [7, 2, 3], [3, 2, 3]];
What if we want to sort by the first column of each sub-array? We can define a comparison function:
arr.sort(function(x, y){
return x[0] – y[0];
});
What is the role of the comparison function here? In fact, the array copies the array elements to x and y in sequence. For example, first assign arr[0] to x, arr[1] to y, and then use x[0] – y[0]. According to the returned value, if If the number returned is greater than 0, then put x in the array after y. If the returned number is 0, it will remain unchanged. If it is less than 0, put x in front of y. Then, after the first one is sorted, proceed to the following Sort the two until the entire array is sorted. This is the default ascending comparison function. If you want to sort in descending order, you only need to modify the comparison method to return y[0] – x[0]. Here x[0] means sorting by the first column. We Sorting by other columns can also be done here. The sorting here will modify the array structure of arr by default, so after sorting arr will be an array in ascending order of the first column.
2. Sort by string
If you sort by string, we can use the localeCompare method provided by js,
localeCompare function: use locale-specific To compare two strings sequentially.
The usage rule of localeCompare method is stringObject.localeCompare(target). If stringObject is less than target, localeCompare() returns a number less than 0. If stringObject is greater than target, this method returns a number greater than 0. If the two strings are equal, or there is no difference according to the local sorting rules, this method returns 0. The comparer uses local rules. The local rules mean that the underlying rules of the operating system are used to sort these local characters. By default For example, a comparison using the greater than sign simply compares the unicode numbers of two characters, which is inconsistent with many languages.
For example
var arr = [['中' ,'国'], ['Ah','的'], ['Oh','的']];
arr.sort(function(x, y){
return x[0]. localeCompare(y[0]);
});
The results will be sorted by the pinyin of the Chinese characters in the first column. If it contains English, the default is to put the English in front. If If it is pure English, it will be in alphabetical order, with uppercase letters coming after lowercase letters, so that string sorting can be achieved, including Chinese and Chinese-English mixed sorting. As for sorting in descending order, the method is the same as above, change to return y[0].localeCompare(x[0]);.
In this way, the sorting problem is realized. There are still many places where js two-dimensional array sorting is used. Hope it can help some people
function tblSort(s){
for(r = 0;r < row_len;r ){
arrs[r]=[]
for(c=0;c< cel_len;c ){
arrs[r][c] ={}//Create another object in the two-dimensional array;
arrs[r][c].html = table.rows[r].cells [c].innerHTML//Get the table HTML and put it into an associative array for sorting and displaying on the page; The text content is used for the following judgment; arrs [r][c].text='-1';
[c].text='-2';
}else if(text == ''){
arrs[r][c] rrs[r][c].text=table.rows[r].cells [c].innerText//Get the text content of the table and put it into an associative array. Used for sorting below.
0]['text'])
Reverse order;
if(reve){
arrs.sort(function(x,y){
']) - parseFloat(x[s][' text']) 🎜> arrs.sort(function(x,y){
arrs.sort(function (x,y) { ]) - parseFloat(y[s]['text'])//Sort
//Put the arranged html content into the table
for(r = 0;r < row_len;r ){
for(c=0;c
🎜>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


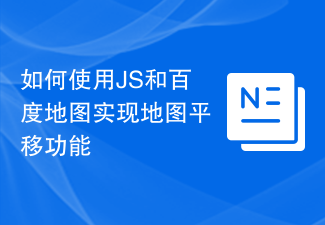
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
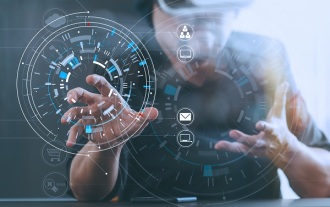
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
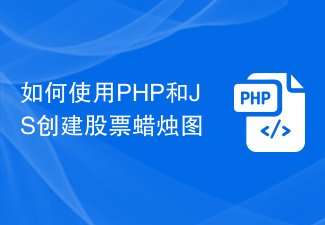
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
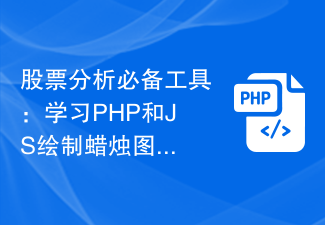
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
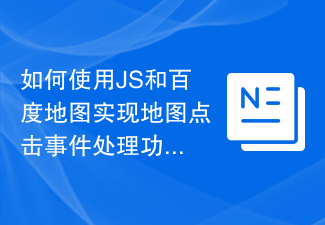
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
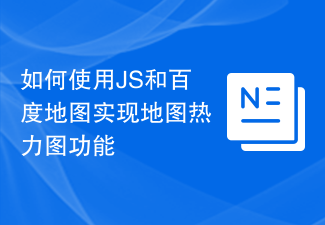
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
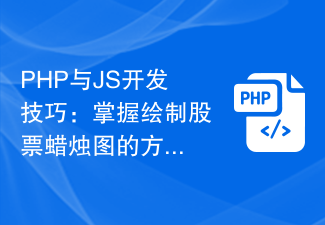
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
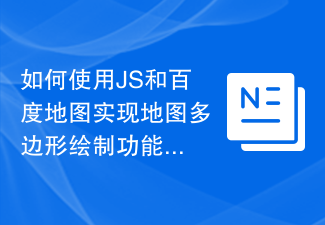
How to use JS and Baidu Maps to implement map polygon drawing function. In modern web development, map applications have become one of the common functions. Drawing polygons on the map can help us mark specific areas for users to view and analyze. This article will introduce how to use JS and Baidu Map API to implement map polygon drawing function, and provide specific code examples. First, we need to introduce Baidu Map API. You can use the following code to import the JavaScript of Baidu Map API in an HTML file
