jquery basic tutorial: How to use deferred objects_jquery
1. What is a deferred object?
In the process of developing websites, we often encounter certain JavaScript operations that take a long time. Among them, there are both asynchronous operations (such as ajax reading server data) and synchronous operations (such as traversing a large array), and the results are not available immediately.
The usual approach is to specify callback functions for them. That is, specify in advance which functions should be called once they have finished running.
However, jQuery is very weak when it comes to callback functions. In order to change this, the jQuery development team designed the deferred object.
To put it simply, the deferred object is jQuery’s callback function solution. In English, defer means "delay", so the meaning of a deferred object is to "delay" execution until a certain point in the future.
It solves the problem of how to handle time-consuming operations, provides better control over those operations, and a unified programming interface. Its main functions can be summarized into four points. Below we will learn step by step through sample code.
2. Chain writing method of ajax operation
First, let’s review the traditional way of writing jQuery’s ajax operation:
$.ajax({
url: "test .html",
success: function(){
alert("Haha, successful!");
},
error:function(){
alert("An error occurred!");
}
});
In the above code, $.ajax() accepts an object parameter. This object contains two methods: the success method specifies the callback function after the operation is successful, and the error method specifies the callback function after the operation fails.
After the $.ajax() operation is completed, if you are using a version of jQuery lower than 1.5.0, the XHR object will be returned and you cannot perform chain operations; if the version is higher than 1.5.0, the returned Deferred objects can be chained.
Now, the new way of writing is like this:
$.ajax("test.html")
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
As you can see, done() is equivalent to the success method, and fail() is equivalent to the error method. After adopting the chain writing method, the readability of the code is greatly improved.
3. Specify multiple callback functions for the same operation
One of the great benefits of the deferred object is that it allows you to add multiple callback functions freely.
Taking the above code as an example, if after the ajax operation is successful, in addition to the original callback function, I also want to run another callback function, what should I do?
It’s very simple, just add it at the end.
$.ajax("test.html")
.done(function(){ alert("Haha, successful!");} )
.fail(function(){ alert("An error occurred!"); } )
.done(function( ){ alert("Second callback function!");} );
You can add as many callback functions as you like, and they will be executed in the order they are added.
4. Specify callback functions for multiple operations
Another great benefit of the deferred object is that it allows you to specify a callback function for multiple events, which is not possible with traditional writing.
Please look at the following code, which uses a new method $.when():
$.when($.ajax("test1.html "), $.ajax("test2.html"))
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert(" Something went wrong! "); });
The meaning of this code is to first perform two operations $.ajax("test1.html") and $.ajax("test2 .html"), if all succeed, the callback function specified by done() will be executed; if one fails or both fail, the callback function specified by fail() will be executed.
5. Callback function interface for common operations (Part 1)
The biggest advantage of the deferred object is that it extends this set of callback function interfaces from ajax operations to all operations. In other words, any operation - whether it is an ajax operation or a local operation, whether it is an asynchronous operation or a synchronous operation - can use various methods of the deferred object to specify a callback function.
Let’s look at a specific example. Suppose there is a time-consuming operation wait:
var wait = function(){
var tasks = function(){
alert("Execution completed!");
};
setTimeout(tasks,5000);
};
We specify a callback function for it. What should we do?
Naturally, you will think that you can use $.when():
$.when(wait())
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
However, if written like this, the done() method will be executed immediately and will not function as a callback function. The reason is that the parameters of $.when() can only be deferred objects, so wait() must be rewritten:
var dtd = $.Deferred(); // Create a new deferred object
var wait = function(dtd){
var tasks = function(){
alert("Execution completed!");
dtd.resolve(); // Change the execution status of the deferred object
};
setTimeout(tasks,5000);
return dtd;
};
Now, the wait() function returns a deferred object, so chain operations can be added.
$.when(wait(dtd))
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
After the wait() function is run, the callback function specified by the done() method will automatically run.
6. deferred.resolve() method and deferred.reject() method
If you look carefully, you will find that there is another place in the wait() function above that I did not explain. That's what dtd.resolve() does?
To clarify this issue, we need to introduce a new concept "execution state". jQuery stipulates that deferred objects have three execution states - unfinished, completed and failed. If the execution status is "completed" (resolved), the deferred object immediately calls the callback function specified by the done() method; if the execution status is "failed", the callback function specified by the fail() method is called; if the execution status is "unsuccessful" Completed", continue to wait, or call the callback function specified by the progress() method (added in jQuery 1.7 version).
During the ajax operation in the previous part, the deferred object will automatically change its execution status based on the return result; however, in the wait() function, this execution status must be manually specified by the programmer. The meaning of dtd.resolve() is to change the execution status of the dtd object from "unfinished" to "completed", thus triggering the done() method.
Similarly, there is also a deferred.reject() method, which changes the execution status of the dtd object from "incomplete" to "failed", thereby triggering the fail() method.
var dtd = $.Deferred(); // New A Deferred object
var wait = function(dtd){
var tasks = function(){
alert("Execution completed!");
dtd.reject(); // Change the execution status of the Deferred object
};
setTimeout(tasks,5000);
return dtd;
};
$.when(wait(dtd))
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
7. deferred.promise() method
There are still problems with the way of writing above. That is, dtd is a global object, so its execution status can be changed from the outside.
Please look at the code below:
var dtd = $.Deferred(); // Create a new Deferred object
var wait = function(dtd){
var tasks = function(){
alert("Execution completed!");
dtd.resolve(); // Change the execution status of the Deferred object
};
setTimeout(tasks,5000);
return dtd;
};
$.when(wait(dtd))
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
dtd.resolve();
I added a line of dtd.resolve() at the end of the code, which changed the execution status of the dtd object, thus causing the done() method to be executed immediately, and the "Haha, successful!" prompt box popped up, etc. 5 After a few seconds, the "Execution Completed!" prompt box will pop up.
To avoid this situation, jQuery provides the deferred.promise() method. Its function is to return another deferred object on the original deferred object. The latter only opens methods that are not related to changing the execution status (such as the done() method and fail() method), and blocks methods related to changing the execution status ( Such as resolve() method and reject() method), so that the execution status cannot be changed.
Please look at the code below:
var dtd = $.Deferred(); // Create a new Deferred object
var wait = function(dtd){
var tasks = function(){
alert("Execution completed!");
dtd.resolve(); // Change the execution status of the Deferred object
};
setTimeout(tasks,5000);
return dtd.promise(); // Return promise object
};
var d = wait(dtd); // Create a new d object and operate on this object instead
$.when(d)
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
d.resolve(); // At this time, this statement is invalid
In the above code, the wait() function returns a promise object. Then, we bind the callback function to this object instead of the original deferred object. The advantage of this is that the execution status of this object cannot be changed. If you want to change the execution status, you can only operate the original deferred object.
However, a better way to write it is as pointed out by allenm, to turn the dtd object into the internal object of the wait() function.
var wait = function(dtd){
var dtd = $.Deferred(); //Within the function, create a new Deferred object
var tasks = function(){
alert("Execution completed!");
dtd.resolve(); // Change the execution status of the Deferred object
};
setTimeout(tasks,5000);
return dtd.promise(); // Return promise object
};
$.when(wait())
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
8. Callback function interface for normal operations (middle)
Another way to prevent the execution state from being changed externally is to use the constructor function $.Deferred() of the deferred object.
At this time, the wait function remains unchanged, and we directly pass it into $.Deferred():
$.Deferred(wait)
.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
jQuery stipulates that $.Deferred() can accept a function name (note, it is a function name) as a parameter, and the deferred object generated by $.Deferred() will be used as the default parameter of this function.
9. Callback function interface for common operations (Part 2)
In addition to the above two methods, we can also deploy the deferred interface directly on the wait object.
var dtd = $.Deferred(); // Generate Deferred object
var wait = function(dtd){
var tasks = function(){
alert("Execution completed!");
dtd.resolve(); // Change the execution status of the Deferred object
};
setTimeout(tasks,5000);
};
dtd.promise(wait);
wait.done(function(){ alert("Haha, successful!"); })
.fail(function(){ alert("Error!"); });
wait(dtd);
The key here is the line dtd.promise(wait), which is used to deploy the Deferred interface on the wait object. It is precisely because of this line that done() and fail() can be called directly on wait later.
10. Summary: Methods of deferred objects
We have already talked about the various methods of deferred objects. Here is a summary:
(1) $.Deferred() generates a deferred object.
(2) deferred.done() specifies the callback function when the operation is successful
(3) deferred.fail() specifies the callback function when the operation fails
(4) When deferred.promise() has no parameters, it returns a new deferred object, and the running status of the object cannot be changed; when it accepts parameters, it serves to deploy the deferred interface on the parameter object.
(5) deferred.resolve() Manually changes the running status of the deferred object to "Completed", thus triggering the done() method immediately.
(6) deferred.reject() This method is exactly the opposite of deferred.resolve(). After being called, the running status of the deferred object will be changed to "failed", thus triggering the fail() method immediately.
(7) $.when() specifies callback functions for multiple operations.
In addition to these methods, the deferred object also has two important methods, which are not covered in the above tutorial.
(8)deferred.then()
Sometimes to save trouble, done() and fail() can be written together. This is the then() method.
$.when($.ajax( "/main.php" ))
.then(successFunc, failureFunc );
If then() has two parameters, then the first parameter is the callback function of the done() method, and the second parameter is the callback method of the fail() method. If then() has only one parameter, it is equivalent to done().
(9)deferred.always()
This method is also used to specify the callback function. Its function is that no matter whether deferred.resolve() or deferred.reject() is called, it will always be executed in the end.
$.ajax( "test.html" )
.always( function() { alert("Executed!");} );

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


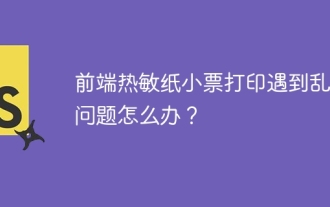
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
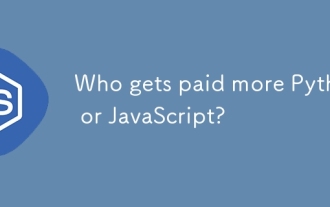
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
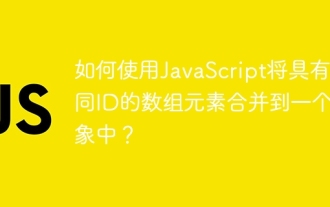
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
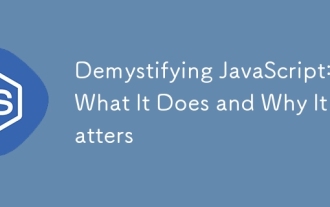
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
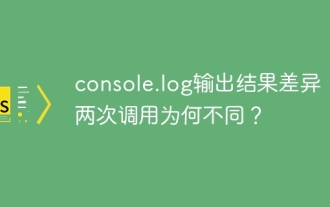
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
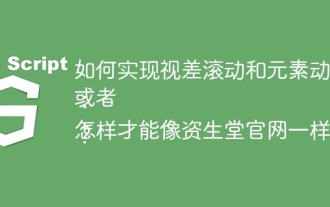
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
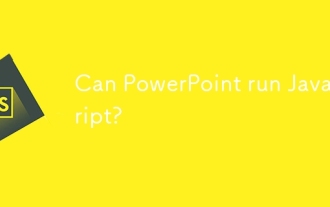
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
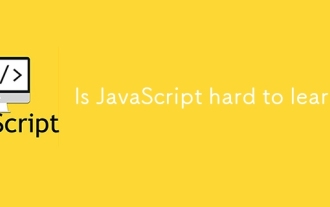
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
