


Two methods of jQuery plug-in development and detailed explanation of $.fn.extend_jquery
There are two types of jQuery plug-in development:
1 Class level
Class level can be understood as extending the jquery class. The most obvious example is $.ajax(...), which is equivalent to static method.
Use the $.extend method when developing and extending its methods, that is, jQuery.extend(object);
$.extend({
add:function(a,b){return a b;} ,
minus:function(a, b){return a-b;}
});
Called in the page:
var i = $.add(3,2);
var j = $.minus(3,2);
2 Object level
The object level can be understood as object-based expansion, such as $("#table").changeColor(...); The changeColor here is an object-based expansion.
Use the $.fn.extend method when developing and extending its methods, that is, jQuery.fn.extend(object);
$.fn.extend({
check:function(){
return this.each({
this. checked=true;
});
},
uncheck:function(){
return this.each({
this.checked=false;
});
}
});
Call in the page:
$('input[type=checkbox]').check();
$('input[type=checkbox]').uncheck();
3. Extension
$.xy = {
add:function(a,b){return a b;} ,
minus:function(a,b){return a-b;},
voidMethod:function(){ alert("void"); }
};
var i = $.xy.add(3,2);
var m = $.xy.minus(3,2);
$.xy.voidMethod();

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


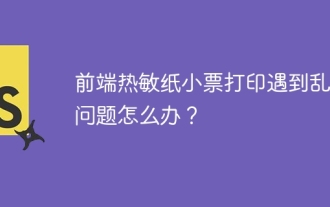
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
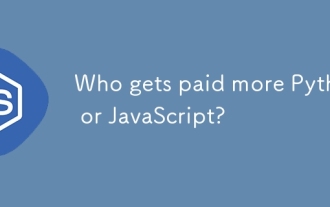
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
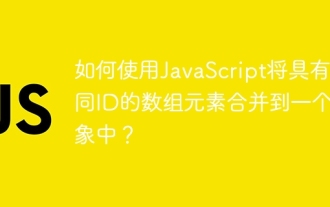
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
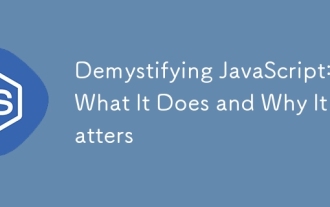
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
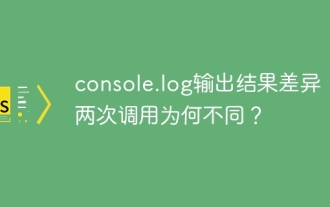
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
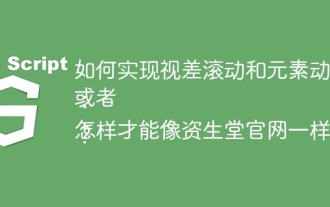
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
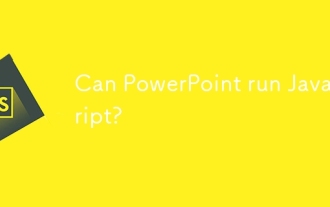
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
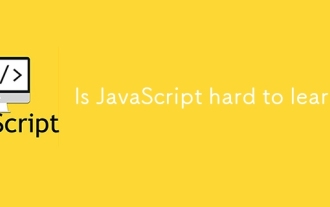
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
