


Specific implementation method of js class inheritance_Basic knowledge
Before you start tinkering with code, you should understand the purpose and benefits of using inheritance. Generally speaking, when designing classes, we hope to reduce repetitive code and try to weaken the coupling between classes. It is difficult to balance both. We need to decide what method we should take based on specific conditions and circumstances. According to our understanding of inheritance in object-oriented languages, inheritance will bring direct strong coupling of classes, but due to its unique flexibility, js can design codes with strong coupling and weak coupling, high efficiency and low efficiency. What to use depends on the situation.
The following are three ways to implement inheritance in js: class inheritance, prototypal inheritance, and metaclasses. Class inheritance will be briefly described here first, and the last two will be briefly described later. Please pay more attention and guidance, thank you.
Classic inheritance.
The implementation of js class inheritance relies on the prototype chain. What is a prototype chain? Objects in js have an attribute called prototype. This attribute returns a reference to the object type and is used to provide a set of basic functions of the object's class.
It seems that I have an impression of prototype. By the way, we often use code like this.
var Person = function(){
this. name = "liyatang";
};
Person.prototype = {
//The basic functions of Person can be provided here
getName: function(){
return this.name;
}
}
We put the basic functions of the class in the prototype attribute, indicating that the reference to the Person object has XXX functions.
After understanding prototypes, you need to understand what a prototype chain is. When accessing a member (property or method) of an object, if this member is not found in the current object, js will search for it in the object pointed to by the prototype attribute. If it is not found yet, it will continue to the next-level prototype. Search the pointed object until it is found. If not found, undefined will be returned.
So what hints does the prototype chain give us? It is easy to think that the prototype chain means that for one class to inherit another class, you only need to set the prototype of the subclass to point to an instance of the parent class. This binds the members of the parent class to the child class, because if a member cannot be found in the child class, it will be searched in the parent class. (The wording in the above two paragraphs is not rigorous, just describing it in easy-to-understand terms)
Next we need a Chinese class, which needs to inherit the name and getName members of the Person class.
var Chinese = function(name, nation){
//Inheritance, you need to call the constructor of the parent class, which can be called with call, this points to Chinese
//Only when Person is in this scope can you call members of Person
Person.call(this,name );
this.nation = nation;
};
Chinese.prototype = Person.prototype;
//This cannot be the same as before, because the prototype attribute is overwritten
//Chinese .prototype = {
// getNation : function(){
// return this.nation;
// }
//};
//All subsequent methods need to be added like this
Chinese.prototype.getNation = function(){
return this.nation;
};
The inheritance relationship is established, we call it like this
var c = new Chinese("liyatang","China ");
alert(c.getName());// liyatang
So class inheritance is completed. Is it really completed? Use firebug to set a breakpoint in alert, and you will find that the original Person.prototype has been modified and the getNation method has been added.
This is because in the above code Chinese.prototype = Person.prototype; this is a reference type. Modifying Chinese also modifies Person. This in itself is intolerable and creates strong coupling between classes. This is not the effect we want.
We can create a new object or instantiate an instance to weaken coupling.
//First type
//Chinese. prototype = new Person();
//Second type
//var F = function(){};
//F.prototype = Person.prototype;
//Chinese.prototype = F.prototype;
What is the difference between these two methods. In the second type, an empty function F is added. This can avoid creating an instance of the parent class, because the parent class may be relatively large, and the constructor of the parent class will have some side effects or perform a large amount of calculations. Task. Therefore, we strongly recommend the second method.
This is it, it’s over, not yet! There is an attribute constructor under the object's prototype attribute, which holds a reference to the function that constructs a specific object instance. According to this statement Chiese.prototype.constructor should be equal to Chinese, but it is not.
Recall that when setting up the prototype chain of Chiese, we overwrote Chiese.prototype with Person.prototype. So the Chiese.prototype.constructor at this time is Person. We also need to add the following code
//You don’t need to study the if condition here in detail, you know Just Chinese.prototype.constructor = Chinese
if(Chinese.prototype.constructor == Object.prototype.constructor){
Chinese.prototype.constructor = Chinese;
}
Organize all the codes as follows
var Person = function( name){
this.name = name;
};
Person.prototype = {
getName : function(){
return this.name;
}
} ;
var Chinese = function(name, nation){
Person.call(this,name);
this.nation = nation;
};
var F = function (){};
F.prototype = Person.prototype;
Chinese.prototype = F.prototype;
if(Chinese.prototype.constructor == Object.prototype.constructor){
Chinese .prototype.constructor = Chinese;
}
Chinese.prototype.getNation = function(){
return this.nation;
};
var c = new Chinese(" liyatang","China");
alert(c.getName());
If you can put the inherited code in a function to facilitate code reuse, the final code is organized as follows
function extend(subClass,superClass){
var F = function(){};
F.prototype = superClass.prototype;
subClass.prototype = new F();
subClass.prototype.constructor = subClass;
subClass.superclass = superClass .prototype; //Add an extra attribute to point to the parent class itself in order to call the parent class function
if(superClass.prototype.constructor == Object.prototype.constructor){
superClass.prototype.constructor = superClass;
}
}
var Person = function(name){
this.name = name;
};
Person.prototype = {
getName : function( ){
return this.name;
}
};
var Chinese = function(name, nation){
Person.call(this,name);
this.nation = nation;
};
extend(Chinese, Person);
Chinese.prototype.getNation = function(){
return this.nation;
};
var c = new Chinese("liyatang","China");
alert(c.getName());
Revised after publishing:
Under the comments on the first floor, I have new views on the extend function. Two methods were proposed before when discussing how to set up the prototype chain
//First type
//Chinese. prototype = new Person();
//Second type
//var F = function(){};
//F.prototype = Person.prototype;
//Chinese.prototype = F.prototype;
Although the second method reduces the need to call the constructor of the parent class, Person.call(this,name) was used when designing the Chinese class; this is also equivalent to calling the constructor of the parent class.
However, using the first method can reduce the need to write Person.call(this,name); in Chinese. This part of the code is often forgotten in subclasses. You might as well put this functional code in extend. Just write
Chinese.prototype = new Person(); also achieves the same purpose: the coupling is not strong.
But the forgotten thing is that Chinese.prototype = new Person(); is written correctly. The answer is no! Obviously new Person() needs to pass a name parameter. We can't do this part of the work in the extend function, so we have to call the constructor of the parent class in the Chinese class. This is also in line with object-oriented thinking.
So, it is still highly recommended to use the second method.
This is the first time I write a technical article like this. I basically develop it according to my own ideas. It is inevitable that there will be some places that have not been taken into consideration and unclear explanations. I hope to leave a message for feedback, thank you.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
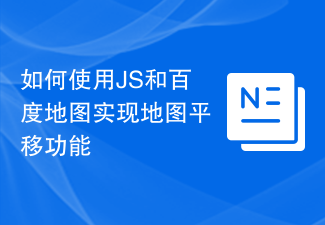
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
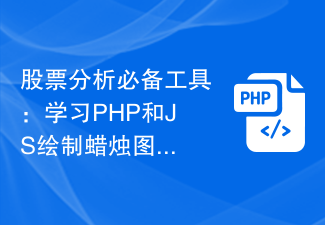
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
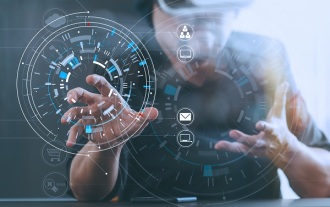
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
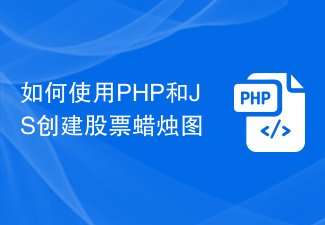
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
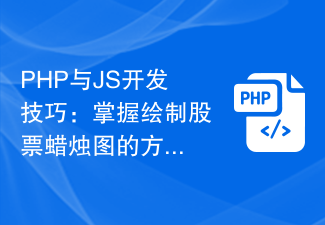
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
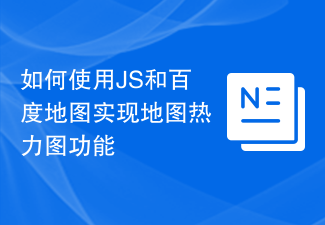
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
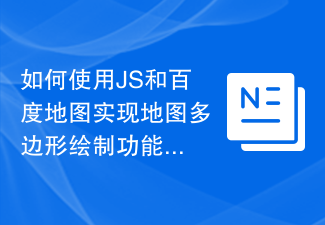
How to use JS and Baidu Maps to implement map polygon drawing function. In modern web development, map applications have become one of the common functions. Drawing polygons on the map can help us mark specific areas for users to view and analyze. This article will introduce how to use JS and Baidu Map API to implement map polygon drawing function, and provide specific code examples. First, we need to introduce Baidu Map API. You can use the following code to import the JavaScript of Baidu Map API in an HTML file
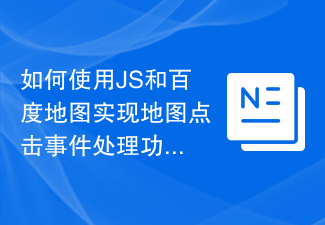
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
