


Summary of several usages of inheritance in js (apply, call, prototype)_javascript skills
1. Object inheritance in js
There are three inheritance methods in js
1.js prototype implementation inheritance
2. Constructor implementation inheritance
3.call, apply implements inheritance
How to use apply (detailed introduction)
Both call and apply in js can be inherited. The only parameter difference is that the apply corresponding to func.call(func1,var1,var2,var3) is written as: func.apply(func1,[var1,var2,var3] ).
Explanation of call in the JS manual:
Copy code The code is as follows:
< ;SPAN style="FONT-SIZE: 18px">call method
Call a method of an object to replace the current object with another object.
Explanation The
call method can be used to call a method instead of another object. The call method changes the object context of a function from the initial context to the new object specified by thisObj.
If the thisObj parameter is not provided, the Global object is used as thisObj.
To put it simply, the function of these two functions is actually to change the internal pointer of the object, that is, to change the content pointed to by this of the object. This is sometimes useful in object-oriented js programming. Let's take apply as an example to talk about the important role of these two functions in js. For example:
Copy code
The code is as follows:
function Person(name,age){ //Define a class
this.name=name; //Name
this. age=age; //Age
this.sayhello=function(){alert(this.name)};
}
function Print(){ //Display class attributes
this.funcName ="Print";
this.show=function(){
var msg=[];
for(var key in this){
if(typeof(this[key])!= "function"){
g.join(" "));
};
}
function Student(name,age,grade,school){ //Student class
Person.apply(this,arguments);//Superior to call Place
Print.apply(this,arguments);
this.grade=grade; //Grade
this.school=school; //School
}
var p1=new Person( "Bu Kaihua",80);
p1.sayhello();
var s1=new Student("Bai Yunfei",40,9,"Yuelu Academy");
s1.show();
s1.sayhello();
alert(s1.funcName);
In addition, Function.apply() plays a prominent role in improving program performance:
Let’s start with the Math.max() function. Math.max can be followed by any number of parameters, and finally returns the maximum value among all parameters.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


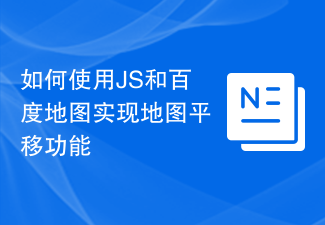
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
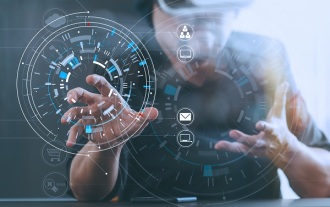
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
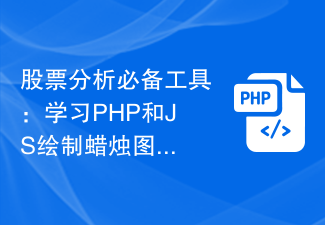
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
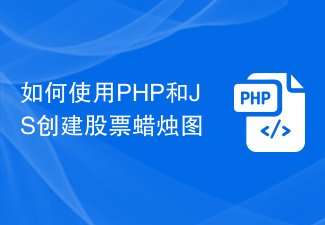
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
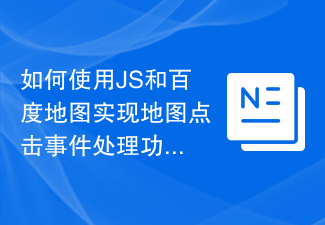
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
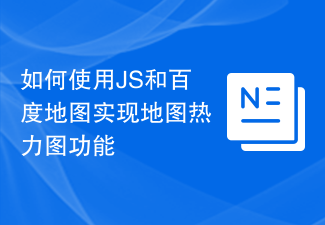
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
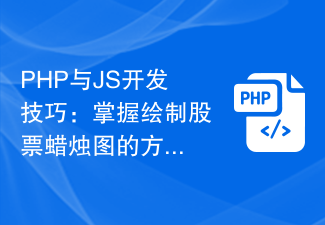
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
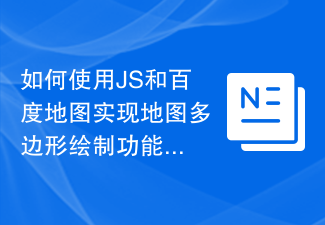
How to use JS and Baidu Maps to implement map polygon drawing function. In modern web development, map applications have become one of the common functions. Drawing polygons on the map can help us mark specific areas for users to view and analyze. This article will introduce how to use JS and Baidu Map API to implement map polygon drawing function, and provide specific code examples. First, we need to introduce Baidu Map API. You can use the following code to import the JavaScript of Baidu Map API in an HTML file
