JQuery's Pager implementation code_jquery
The example in this article shares the specific implementation code of JQuery's Pager for your reference. The specific content is as follows
Rendering:
Code:
html code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>分页器</title> <link href="pager.css" rel="stylesheet"/> </head> <body> <div id="pager"></div> <script src="pager.js"></script> <script> function doChangePage(obj){ //TO DO } var pagerBox = document.getElementById('pager'); var pager = new Pager({ index: 1, total: 15, parent: pagerBox, onchange: doChangePage }); </script> </body> </html>
css code:
.pager-box:after{ display:block; height:0; visibility:hidden; clear:both; content:''; } .pager{ float:left; position:relative; left:50%; font-family:微软雅黑; } .pager a,.pager span{ position:relative; left:-50%; display:block; float:left; margin-left:5px; border:1px solid #b6bcc1; padding: 5px 10px; text-decoration:none; color:#b6bcc1; border-radius:3px; } .pager span{ border:0; } .pager a.js-selected{ background:#b6bcc1; color:#fff; cursor:default; } .pager a.js-disabled{ background:#f1f1f1; border-color:#f1f1f1; cursor:default; color:#fff; }
pager.js code
(function(window, undefined){ /** * 创建元素节点并返回 */ function create(tagName, className, parent){ var element = document.createElement(tagName); element.className = className; parent.appendChild(element); return element; } /** * 数组消除重复 */ function clearRepeat(arr){ var obj = {}, result = []; for(var i = 0, len = arr.length; i < len; i++){ obj[arr[i]] = 1; } for(var i in obj){ result.push(i); } return result; } /** * 添加类名 */ function addClassName(element, className){ var aClass = element.className.split(' '); aClass.push(className); aClass = clearRepeat(aClass); element.className = aClass.join(' '); } /** * 删除类名 */ function delClassName(element, className){ var aClass = element.className.split(' '), index = aClass.indexOf(className); if(index > 0) aClass.splice(index, 1); element.className = aClass.join(' '); } /** * 检查是否含有类名 * @param element * @param className * @returns {boolean} */ function hasClassName(element, className){ var aClass = element.className.split(' '), index = aClass.indexOf(className); if(index > 0) return true; return false; } var Pager = function(obj){ this.__total = obj.total || 1; this.__index = obj.index || 1; this.__parent = obj.parent; this.__onchange = obj.onchange; //初始化分页器 this.__init(obj); }; var pro = Pager.prototype; /** * 初始化分页器 */ pro.__init = function(obj){ if(this.__total < this.__index) return; //存储数字 this.__numbers = []; //储存省略号 this.__dots = []; this.__wrapper = create('div', 'pager-box', this.__parent); this.__body = create('div', 'pager', this.__wrapper); //存储上一页 this.__preBtn = create('a', 'prev', this.__body); this.__preBtn.href = 'javascript:void(0);'; this.__preBtn.innerText = (obj.label && obj.label.prev) || '上一页'; //存储数字 if(this.__total < 8){ for(var i = 0; i < this.__total; i++){ var t = create('a', 'number', this.__body); t.href = 'javascript:void(0);'; t.innerText = i + 1; this.__numbers.push(t); } }else{ for(var i = 0; i < 2; i++){ var t = create('span', 'dots', this.__body); t.innerText = '...'; this.__dots.push(t); }; for(var i = 0; i < 7; i++){ var t = create('a', 'number', this.__body); t.href = 'javascript:void(0);'; this.__numbers.push(t); } } //存储下一页 this.__nextBtn = create('a', 'next', this.__body); this.__nextBtn.href = 'javascript:void(0);'; this.__nextBtn.innerText = (obj.label && obj.label.next) || '下一页'; // this._$setIndex(this.__index); // this.__body.onclick = this.__doClick.bind(this); }; pro.__doClick = function(e){ var e = e || window.event, target = e.target || e.srcElement; //点击省略号 if(target.tagName.toLowerCase() == 'span') return; //点击了不能点击的上一页或者下一页 if(hasClassName(target, 'js-disabled')) return; //点击了当前页 if(hasClassName(target, 'js-selected')) return; if(target == this.__preBtn){ //点击了上一页 this._$setIndex(this.__index - 1); }else if(target == this.__nextBtn){ //点击了下一页 this._$setIndex(this.__index + 1); }else{ //点击了数字 var index = target.innerText; this._$setIndex(index); } }; /** * 跳转页数 */ pro._$setIndex = function(index){ index = parseInt(index); //更新信息 if(index != this.__index){ this.__last = this.__index; this.__index = index; } //处理 delClassName(this.__preBtn, 'js-disabled'); delClassName(this.__nextBtn, 'js-disabled'); if(this.__total < 8){ //总页数小于8的情况 if(this.__last) delClassName(this.__numbers[this.__last - 1], 'js-selected'); addClassName(this.__numbers[this.__index - 1], 'js-selected'); if(this.__index == 1) addClassName(this.__preBtn, 'js-disabled'); if(this.__index == this.__total) addClassName(this.__nextBtn, 'js-disabled'); }else{ this.__dots[0].style.display = 'none'; this.__dots[1].style.display = 'none'; for(var i = 0; i < 7; i++){ delClassName(this.__numbers[i], 'js-selected'); }; if(this.__index < 5){ for(var i = 0; i < 6; i++){ this.__numbers[i].innerText = i + 1; } this.__numbers[6].innerText = this.__total; this.__dots[1].style.display = 'block'; this.__body.insertBefore(this.__dots[1], this.__numbers[6]); addClassName(this.__numbers[this.__index - 1], 'js-selected'); if(this.__index == 1) addClassName(this.__preBtn, 'js-disabled'); }else if(this.__index > this.__total - 4){ for(var i = 1; i < 7; i++){ this.__numbers[i].innerText = this.__total + i -6; } this.__numbers[0].innerText = '1'; this.__dots[0].style.display = 'block'; this.__body.insertBefore(this.__dots[0], this.__numbers[1]); addClassName(this.__numbers[this.__index + 6 - this.__total], 'js-selected'); if(this.__index == this.__total) addClassName(this.__nextBtn, 'js-disabled'); }else{ this.__numbers[0].innerText = '1'; for(var i = 1; i < 6; i++){ this.__numbers[i].innerText = this.__index - 3 + i; if(i == 3) addClassName(this.__numbers[i], 'js-selected'); } this.__numbers[6].innerText = this.__total; this.__dots[0].style.display = 'block'; this.__body.insertBefore(this.__dots[0], this.__numbers[1]); this.__dots[1].style.display = 'block'; this.__body.insertBefore(this.__dots[1], this.__numbers[6]); } } if(typeof this.__onchange == 'function'){ this.__onchange({ index: this.__index, last: this.__last, total: this.__total }) } }; /** * 得到总页数 */ pro._$getIndex = function(){ return this.__index; }; /** * 得到上一个页数 */ pro._$getLast = function(){ return this.__last; }; //变成全局 window.Pager = Pager; })(window);
Main idea:
The paginator is divided into the following 4 situations:
Case 1, when total < 8, all page numbers are displayed.
Case 2, when total >= 8 and index < 5, display 1-6 and the last page.
Case 3, when total >= 8 and index > total - 4, display 1 and the last 6 items.
Case 4, when total >= 8 and 5 <= index <= total - 4, display 1 and the last page, and the 5 items in the middle.
Pass in a settings object when instantiating the Pager class:
{ parent: element, //给分页器设置父节点 index: index, //设置当前页 total: total, //设置总页数 onchange: function(){} //页数变化回调函数 }
When we instantiate Pager, execute the statements in the Pager function body, first assign the value, and then execute the initialization function:
var Pager = function(obj){ //赋值 this.__total = obj.total || 1; this.__index = obj.index || 1; this.__parent = obj.parent; this.__onchange = obj.onchange; //初始化分页器 this.__init(obj); };
Initialization function this.__init structure:
Pager.prototype.__init = function(obj){ (根据上面分析的情况进行处理) ... this._$setIndex(this.__index); //跳转到初始页 //绑定分页器点击函数 this.__body.onclick = this.__doClick.bind(this); };
Initialization is completed. After clicking, the corresponding judgment will be made and this._$setIndex(index) will be used to jump.
For more articles on pagination tutorials, please check out the following topics:
javascript paging function operation
jquery paging function operation
php paging function operation
ASP.NET paging function operation
Download: paper
The above is the entire content of this article, I hope it will be helpful to everyone’s study.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


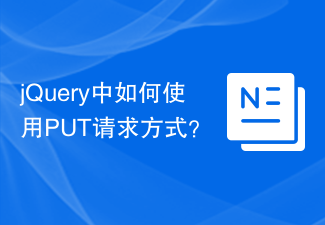
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
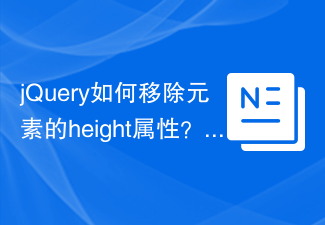
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
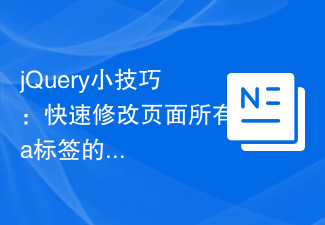
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
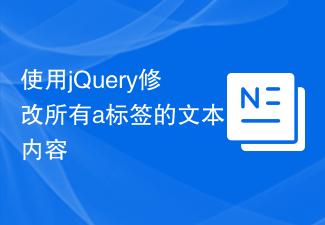
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
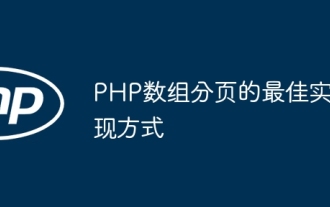
There are two most common ways to paginate PHP arrays: using the array_slice() function: calculate the number of elements to skip, and then extract the specified range of elements. Use built-in iterators: implement the Iterator interface, and the rewind(), key(), current(), next(), and valid() methods are used to traverse elements within the specified range.
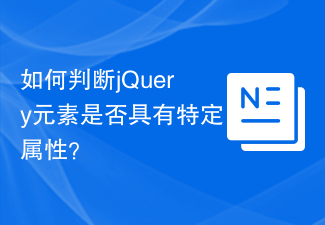
How to tell if a jQuery element has a specific attribute? When using jQuery to operate DOM elements, you often encounter situations where you need to determine whether an element has a specific attribute. In this case, we can easily implement this function with the help of the methods provided by jQuery. The following will introduce two commonly used methods to determine whether a jQuery element has specific attributes, and attach specific code examples. Method 1: Use the attr() method and typeof operator // to determine whether the element has a specific attribute
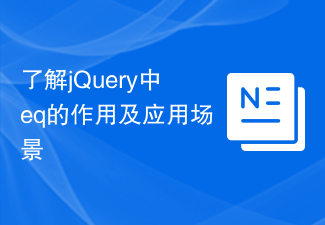
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
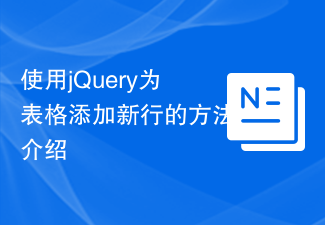
jQuery is a popular JavaScript library widely used in web development. During web development, it is often necessary to dynamically add new rows to tables through JavaScript. This article will introduce how to use jQuery to add new rows to a table, and provide specific code examples. First, we need to introduce the jQuery library into the HTML page. The jQuery library can be introduced in the tag through the following code:
