pygame学习笔记(3):运动速率、时间、事件、文字
1、运动速率
上节中,实现了一辆汽车在马路上由下到上行驶,并使用了pygame.time.delay(200)来进行时间延迟。看了很多参考材料,基本每个材料都会谈到不同配置机器下运动速率的问题,有的是通过设定频率解决,有的是通过设定速度解决,自己本身水平有限,看了几篇,觉得还是《Beginning Game Development with Python and Pygame》这里面提到一个方法比较好。代码如下,代码里更改的地方主要是main里的代码,其中利用clock=pygame.time.Clock()来定义时钟,speed=250.0定义了速度,每秒250像素,time_passed=clock.tick()为上次运行时间单位是毫秒,time_passed_seconds=time_passed/1000.0将单位改为秒,distance_moved=time_passed_seconds*speed时间乘以速度得到移动距离,这样就能保证更加流畅。
import pygame,sys
def lineleft():
plotpoints=[]
for x in range(0,640):
y=-5*x+1000
plotpoints.append([x,y])
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
pygame.display.flip()
def lineright():
plotpoints=[]
for x in range(0,640):
y=5*x-2000
plotpoints.append([x,y])
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
pygame.display.flip()
def linemiddle():
plotpoints=[]
x=300
for y in range(0,480,20):
plotpoints.append([x,y])
if len(plotpoints)==2:
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
plotpoints=[]
pygame.display.flip()
def loadcar(yloc):
my_car=pygame.image.load('ok1.jpg')
locationxy=[310,yloc]
screen.blit(my_car,locationxy)
pygame.display.flip()
if __name__=='__main__':
pygame.init()
screen=pygame.display.set_caption('hello world!')
screen=pygame.display.set_mode([640,480])
screen.fill([255,255,255])
lineleft()
lineright()
linemiddle()
clock=pygame.time.Clock()
looper=480
speed=250.0
while True:
for event in pygame.event.get():
if event.type==pygame.QUIT:
sys.exit()
pygame.draw.rect(screen,[255,255,255],[310,(looper+132),83,132],0)
time_passed=clock.tick()
time_passed_seconds=time_passed/1000.0
distance_moved=time_passed_seconds*speed
looper-=distance_moved
if looper
looper=480
loadcar(looper)
2、事件
我理解的就是用来解决键盘、鼠标、遥控器等输入后做出什么反映的。例如上面的例子,可以通过按上方向键里向上来使得小车向上移动,按下向下,使得小车向下移动。当小车从下面倒出时,会从上面再出现,当小车从上面驶出时,会从下面再出现。代码如下。event.type == pygame.KEYDOWN用来定义事件类型,if event.key==pygame.K_UP这里是指当按下向上箭头时,车前进。if event.key==pygame.K_DOWN则相反,指按下向下箭头,车后退。
import pygame,sys
def lineleft():
plotpoints=[]
for x in range(0,640):
y=-5*x+1000
plotpoints.append([x,y])
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
pygame.display.flip()
def lineright():
plotpoints=[]
for x in range(0,640):
y=5*x-2000
plotpoints.append([x,y])
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
pygame.display.flip()
def linemiddle():
plotpoints=[]
x=300
for y in range(0,480,20):
plotpoints.append([x,y])
if len(plotpoints)==2:
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
plotpoints=[]
pygame.display.flip()
def loadcar(yloc):
my_car=pygame.image.load('ok1.jpg')
locationxy=[310,yloc]
screen.blit(my_car,locationxy)
pygame.display.flip()
if __name__=='__main__':
pygame.init()
screen=pygame.display.set_caption('hello world!')
screen=pygame.display.set_mode([640,480])
screen.fill([255,255,255])
lineleft()
lineright()
linemiddle()
looper=480
while True:
for event in pygame.event.get():
if event.type==pygame.QUIT:
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key==pygame.K_UP:
looper=looper-50
if looper
looper=480
pygame.draw.rect(screen,[255,255,255],[310,(looper+132),83,132],0)
loadcar(looper)
if event.key==pygame.K_DOWN:
looper=looper+50
if looper>480:
looper=-480
pygame.draw.rect(screen,[255,255,255],[310,(looper-132),83,132],0)
loadcar(looper)
3、字体及字符显示
使用字体模块用来做游戏的文字显示,大部分游戏都会有诸如比分、时间、生命值等的文字信息。pygame主要是使用pygame.font模块来完成,常用到的一些方法是:
pygame.font.SysFont(None, 16),第一个参数是说明字体的,可以是"arial"等,这里None表示默认字体。第二个参数表示字的大小。如果无法知道当前系统中装了哪些字体,可以使用pygame.font.get_fonts()来获得所有可用字体。
pygame.font.Font("AAA.ttf", 16),用来使用TTF字体文件。
render("hello world!", True, (0,0,0), (255, 255, 255)),render方法用来创建文字。第一个参数是写的文字;第二个参数是否开启抗锯齿,就是说True的话字体会比较平滑,不过相应的速度有一点点影响;第三个参数是字体的颜色;第四个是背景色,无表示透明。
下面将上面的例子添加当前汽车坐标:
import pygame,sys
def lineleft():
plotpoints=[]
for x in range(0,640):
y=-5*x+1000
plotpoints.append([x,y])
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
pygame.display.flip()
def lineright():
plotpoints=[]
for x in range(0,640):
y=5*x-2000
plotpoints.append([x,y])
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
pygame.display.flip()
def linemiddle():
plotpoints=[]
x=300
for y in range(0,480,20):
plotpoints.append([x,y])
if len(plotpoints)==2:
pygame.draw.lines(screen,[0,0,0],False,plotpoints,5)
plotpoints=[]
pygame.display.flip()
def loadcar(yloc):
my_car=pygame.image.load('ok1.jpg')
locationxy=[310,yloc]
screen.blit(my_car,locationxy)
pygame.display.flip()
def loadtext(xloc,yloc):
textstr='location:'+str(xloc)+','+str(yloc)
text_screen=my_font.render(textstr, True, (255, 0, 0))
screen.blit(text_screen, (50,50))
if __name__=='__main__':
pygame.init()
screen=pygame.display.set_caption('hello world!')
screen=pygame.display.set_mode([640,480])
my_font=pygame.font.SysFont(None,22)
screen.fill([255,255,255])
loadtext(310,0)
lineleft()
lineright()
linemiddle()
looper=480
while True:
for event in pygame.event.get():
if event.type==pygame.QUIT:
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key==pygame.K_UP:
looper=looper-50
if looper
looper=480
if event.key==pygame.K_DOWN:
looper=looper+50
if looper>480:
looper=-132
loadtext(310,looper)
screen.fill([255,255,255])
loadtext(310,looper)
lineleft()
lineright()
linemiddle()
loadcar(looper)
这个例子里直接让背景重绘一下,就不会再像1、2里面那样用空白的rect去覆盖前面的模块了。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
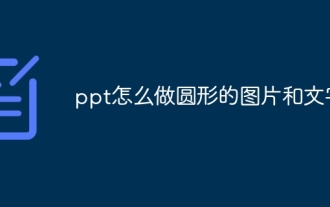
First, draw a circle in PPT, then insert a text box and enter text content. Finally, set the fill and outline of the text box to None to complete the production of circular pictures and text.
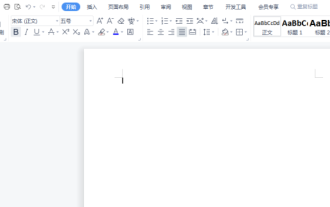
When we create Word documents on a daily basis, we sometimes need to add dots under certain words in the document, especially when there are test questions. To highlight this part of the content, the editor will share with you the tips on how to add dots to text in Word. I hope it can help you. 1. Open a blank word document. 2. For example, add dots under the words "How to add dots to text". 3. We first select the words "How to add dots to text" with the left mouse button. Note that if you want to add dots to that word in the future, you must first use the left button of the mouse to select which word. Today we are adding dots to these words, so we have chosen several words. Select these words, right-click, and click Font in the pop-up function box. 4. Then something like this will appear
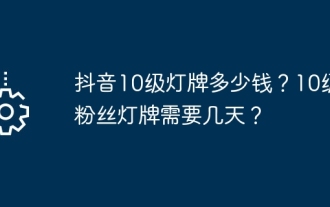
On the Douyin platform, many users are eager to obtain level certification, and the level 10 light sign shows the user's influence and recognition on Douyin. This article will delve into the price of Douyin’s level 10 light boards and the time it takes to reach this level to help users better understand the process. 1. How much does a level 10 Douyin light sign cost? The price of Douyin's 10-level light signs will vary depending on market fluctuations and supply and demand. The general price ranges from a few thousand yuan to ten thousand yuan. This price mainly includes the cost of the light sign itself and possible service fees. Users can purchase level 10 light signs through Douyin’s official channels or third-party service agencies, but they should pay attention to legal channels when purchasing to avoid false or fraudulent transactions. 2. How many days does it take to create a level 10 fan sign? Reach level 10 light sign
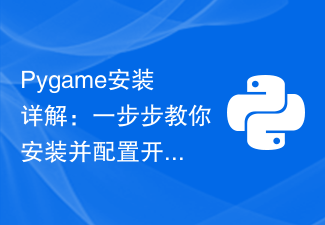
Pygame installation details: Teach you step by step to install and configure the development environment, specific code examples are required Introduction: Pygame is a Python-based game development library. It provides a wealth of tools and functions to make game development simple and interesting. This article will introduce in detail how to install Pygame, configure the development environment, and provide specific code examples. Part 1: Install Pygame Install Python: Before you start installing Pygame, you first need to make sure that Pyt is installed on your computer.
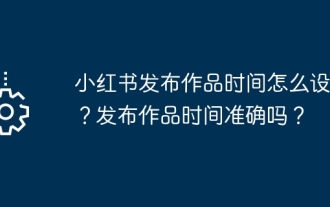
Xiaohongshu, a platform full of life and knowledge sharing, allows more and more creators to express their opinions freely. In order to get more attention and likes on Xiaohongshu, in addition to the quality of content, the time of publishing works is also crucial. So, how to set the time for Xiaohongshu to publish works? 1. How to set the time for publishing works on Xiaohongshu? 1. Understand the active time of users. First, it is necessary to clarify the active time of Xiaohongshu users. Generally speaking, 8 pm to 10 pm and weekend afternoons are the times when user activity is high. However, this time period will also vary depending on factors such as audience group and geography. Therefore, in order to better grasp the active period of users, it is recommended to conduct a more detailed analysis of the behavioral habits of different groups. By understanding users’ lives
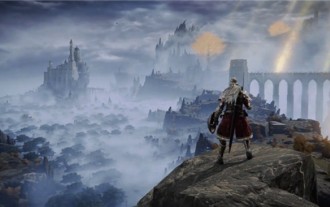
Players can experience the main plot of the game and collect game achievements when playing in Elden's Circle. Many players don't know how long it takes to clear Elden's Circle. The player's clearance process is 30 hours. How long does it take to clear the Elden Ring? Answer: 30 hours. 1. Although this 30-hour clearance time does not refer to a master-like speed pass, it also omits a lot of processes. 2. If you want to get a better game experience or experience the complete plot, then you will definitely need to spend more time on the duration. 3. If players collect them all, it will take about 100-120 hours. 4. If you only take the main line to brush BOSS, it will take about 50-60 hours. 5. If you want to experience it all: 150 hours of base time.
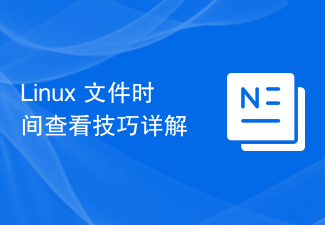
Detailed explanation of Linux file time viewing techniques In Linux systems, file time information is very important for file management and tracking changes. The Linux system records file change information through three main time attributes, namely access time (atime), modification time (mtime) and change time (ctime). This article details how to view and manage this file time information, and provides specific code examples. 1. Check the file time information by using the ls command with the parameter -l to list the files.
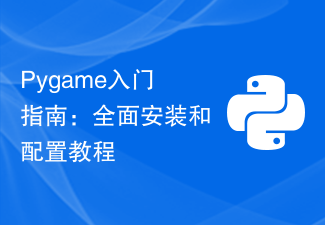
Learn Pygame from scratch: complete installation and configuration tutorial, specific code examples required Introduction: Pygame is an open source game development library developed using the Python programming language. It provides a wealth of functions and tools, allowing developers to easily create a variety of type of game. This article will help you learn Pygame from scratch, and provide a complete installation and configuration tutorial, as well as specific code examples to get you started quickly. Part One: Installing Python and Pygame First, make sure you have
