PHPUnit 入门案例,phpunit入门案例
PHPUnit 入门案例,phpunit入门案例
了解PHPUnit
本案例是关于创建三角形的一个单元测试入门案例,在netbeans环境中完成,关于在此环境中搭建phpunit这里不再描述,可以参考以下资料完成搭建工作:
http://www.cnblogs.com/x3d/p/phpunit-in-netbeans8.html
https://phpunit.de/manual/current/zh_cn/installation.html
https://github.com/sebastianbergmann/phpunit-skeleton-generator
原代码类:
<?php class Triangle { /** * 三条边 第一条边 * @var int */ protected $a; /** * 三条边 第二条边 * @var int */ protected $b; /** * 三条边 第三条边 * @var int */ protected $c; /** * 类型 * @var string */ protected $type; /** * 等边 */ const TYPE_EQUILATERAL = 'Equilateral'; /** * 等腰 */ const TYPE_ISOSCELES = 'Isosceles'; /** * 普通 */ const TYPE_ORDINARY = 'Ordinary'; public function __construct($a = 0, $b = 0, $c = 0) { $this->initSide($a, $b, $c); } /** * 初始化三边 * @param int $a * @param int $b * @param int $c */ protected function initSide(&$a = 0, &$b = 0, &$c = 0) { $this->a = intval($a); $this->b = intval($b); $this->c = intval($c); return $this; } /** * 组建 */ public function create($a, $b, $c) { return $this->initSide($a, $b, $c)->verifySideIsValid(); } /** * 获取类型 */ public function getType() { return $this->verifyType()->type; } /** * 验证三边是否有效 * @return boolean */ protected function verifySideIsValid() { if (intval($this->a) <= 0 || intval($this->b) <= 0 || intval($this->c) <= 0) { return false; } if ($this->a + $this->b <= $this->c) { return false; } if ($this->a + $this->c <= $this->b) { return false; } if ($this->b + $this->c <= $this->a) { return false; } if ($this->a - $this->b >= $this->c) { return false; } if ($this->a - $this->c >= $this->b) { return false; } if ($this->b - $this->c >= $this->a) { return false; } return true; } /** * 验证类型 */ protected function verifyType() { if ($this->isEquilateral()) { $this->type = self::TYPE_EQUILATERAL; return $this; } if ($this->isIsosceles()) { $this->type = self::TYPE_ISOSCELES; return $this; } $this->type = self::TYPE_ORDINARY; return $this; } /** * 是否为等边三角形 */ protected function isEquilateral() { return (($this->a == $this->b ) && ($this->b == $this->c)) ? true : false; } /** * 是否为等腰三角形 */ protected function isIsosceles() { return (($this->a == $this->b ) || ($this->b == $this->c) || ($this->a == $this->c)) ? true : false; } }
生成的测试类文件:
<?php /** * Generated by PHPUnit_SkeletonGenerator on 2016-03-13 at 19:49:12. */ class TriangleTest extends PHPUnit_Framework_TestCase { /** * @var Triangle */ protected $object; /** * Sets up the fixture, for example, opens a network connection. * This method is called before a test is executed. */ protected function setUp() { $this->object = new Triangle; } /** * Tears down the fixture, for example, closes a network connection. * This method is called after a test is executed. */ protected function tearDown() { } /** * @dataProvider addDataProvider * @covers Triangle::create * @todo Implement testCreate(). */ public function testCreate($a, $b, $c) { // Remove the following lines when you implement this test. /** $this->markTestIncomplete( 'This test has not been implemented yet.' ); * */ /* 实现代码 */ $this->assertTrue($this->object->create($a, $b, $c)); } /** * @covers Triangle::getType * @todo Implement testGetType(). */ public function testGetType() { // Remove the following lines when you implement this test. $this->markTestIncomplete( 'This test has not been implemented yet.' ); } /** * 测试用例 * @return array */ public function addDataProvider() { return [ [3, 4, 5], //yes [2, 2, 2], //yes [8, 10, 8], //yes [2, 3, 4], //yes [1, 2, 3], //no [5, 6, 7], //yes [8, 8, 15], //yes [0, 0, 0], //no [-10, 2, 5], //no [0, 2, 1], //no ]; } }
这里需要注意,在我们执行“创建/更新测试”后生成的测试文件类与上面会有些不同,这里的测试用例是手动加上去的,这里具体实现可以查看手册里的说明!
附执行结果:
"/usr/bin/php" "/usr/local/bin/phpunit" "--colors" "--log-junit" "/tmp/nb-phpunit-log.xml" "--bootstrap" "/var/www/html/phpunit/test/bootstrap.php" "/usr/local/netbeans-8.1/php/phpunit/NetBeansSuite.php" "--" "--run=/var/www/html/phpunit/test/core/triangleTest.php" PHPUnit 5.2.10 by Sebastian Bergmann and contributors. ....F..FFFI 11 / 11 (100%) Time: 105 ms, Memory: 10.50Mb There were 4 failures: 1) TriangleTest::testCreate with data set #4 (1, 2, 3) Failed asserting that false is true. /var/www/html/phpunit/test/core/triangleTest.php:47 2) TriangleTest::testCreate with data set #7 (0, 0, 0) Failed asserting that false is true. /var/www/html/phpunit/test/core/triangleTest.php:47 3) TriangleTest::testCreate with data set #8 (-10, 2, 5) Failed asserting that false is true. /var/www/html/phpunit/test/core/triangleTest.php:47 4) TriangleTest::testCreate with data set #9 (0, 2, 1) Failed asserting that false is true. /var/www/html/phpunit/test/core/triangleTest.php:47 FAILURES! Tests: 11, Assertions: 10, Failures: 4, Incomplete: 1. 完成。

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


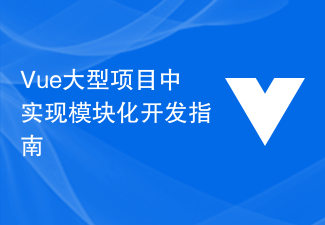
In modern web development, Vue, as a flexible, easy-to-use and powerful front-end framework, is widely used in the development of various websites and applications. When developing large-scale projects, how to simplify the complexity of the code and make the project easier to maintain is a problem that every developer must face. Modular development can help us better organize code, improve development efficiency and code readability. Below, I will share some experiences and guidelines for implementing modular development in Vue large-scale projects: 1. Clear division of labor in a large-scale project
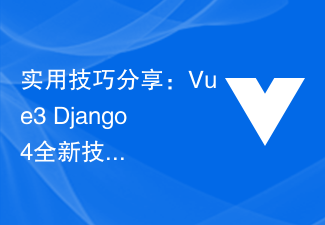
In recent years, front-end technology has developed rapidly, and Vue.js has attracted much attention as an excellent front-end framework. With the official release of Vue.js3 and the upcoming arrival of Django4, combining the two to develop new technology projects is undoubtedly a good solution that can break through technical bottlenecks and improve project development efficiency. This article will share some practical tips to help developers become more comfortable in the Vue3+Django4 technology project development process. First, we need to start a new Vue3+Django4 project,
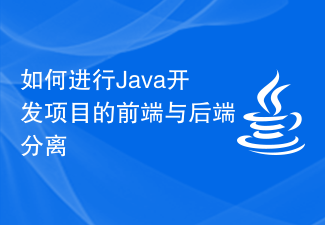
How to separate the front-end and back-end of Java development projects. With the rapid development of the Internet and the continuous improvement of users' experience in Web applications, the development model of separating the front-end and the back-end has gradually become mainstream. In Java development projects, it has a wide range of applications. So, how to separate the front-end and back-end of Java development projects? This article will elaborate on the concept explanation, development process, technology selection, advantages and challenges, etc. 1. Concept explanation The separation of front-end and back-end is a development method that combines the user interface and business logic.
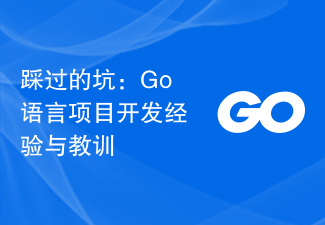
Traps that have been stepped on: Go language project development experience and lessons. On the road of software development, every developer will inevitably step on some pitfalls. Of course, this is no exception for Go language developers. This article will share the pitfalls I have encountered during project development using the Go language, hoping to bring some experience and lessons to other developers. Different versions of Go language When using Go language for project development, we must pay attention to the version of Go language. There may be some language differences or API changes between different versions. These
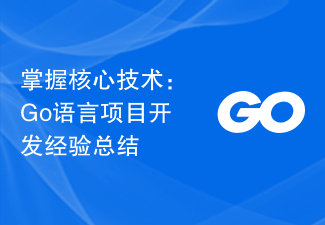
Master the core technology: Summary of Go language project development experience In recent years, with the rapid development of the Internet industry, various new programming languages have emerged one after another and become the new favorite of developers. Among them, Go language, as an open source static compiled language, is loved by many developers for its advantages such as good concurrency performance and high execution efficiency. As a Go language developer, I have practiced in multiple projects and accumulated some experience and summary. In this article, I will share some core technologies and experiences about Go language project development, hoping to
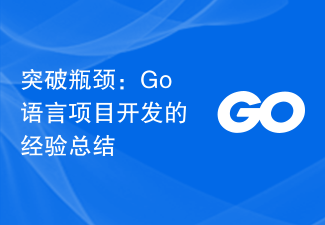
As a relatively new programming language, Go language has received more and more attention in its development in recent years. Especially in project development, the Go language has advantages because it is more suitable than other languages for developing high-performance, concurrent, and distributed systems. However, even using the Go language, you will encounter bottlenecks and challenges in project development, so in this article, we will share some experiences to help break through these bottlenecks. 1. Learn and master the Go language: Before starting project development, you must first master the basic knowledge and programming skills of the Go language.
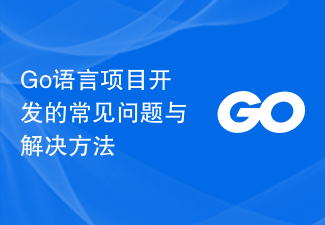
Go language is a high-performance, simple and easy-to-use programming language. More and more developers are beginning to choose it as the preferred language for project development. However, in the actual project development process, we will also encounter some common problems. This article will introduce some of these problems and provide corresponding solutions to help developers better deal with these challenges. Question 1: Dependency management In Go language project development, dependency management is a common problem. Due to the modular nature of the Go language, projects often rely on many third-party packages and libraries. And if
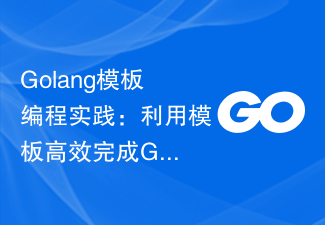
Golang template programming practice: To efficiently use templates to complete project development in Golang, specific code examples are required. Summary: With the continuous development of Golang in the field of software development, more and more developers have begun to pay attention to and use Golang for project development. In Golang, template programming is a very important technology that can help developers complete project development efficiently. This article will introduce how to use templates for project development in Golang and provide specific code examples. Introduction: Gola
