Simple implementation code of JS Map and List_javascript skills
/*
* MAP object, implements MAP function
*
* Interface:
* size() Get MAP element Number of items
* isEmpty() Determine whether MAP is empty
* clear() Delete all elements of MAP
* put(key, value) Add elements (key, value) to MAP
* remove (key) Deletes the element of the specified KEY, returns True if successful, False
on failure * get(key) Gets the element value VALUE of the specified KEY, returns NULL
on failure * element(index) Gets the element of the specified index (use element.key, element.value obtains KEY and VALUE), failure returns NULL
* containsKey(key) Determines whether the MAP contains the element with the specified KEY
* containsValue(value) Determines whether the MAP contains the element with the specified VALUE
* values() Get the array of all VALUEs in the MAP (ARRAY)
* keys() Get the array of all the KEYs in the MAP (ARRAY)
*
* Example:
* var map = new Map();
*
* map.put("key", "value");
* var val = map.get("key")
* ……
*
*/
function Map() {
this.elements = new Array();
//Get the number of MAP elements
this.size = function() {
using using using using . using using out out through through Through out off out through off off through through’’'' through through‐'' ‐‐‐'‐‐‐‐ responsible for to be to go to, ;
};
//Delete all elements of MAP
this.clear = function() {
this.elements = new Array();
};
//Add to MAP Add elements (key, value)
this.put = function(_key, _value) {
this.elements.push( {
key : _key,
value : _value
}) ;
};
//Delete the element of the specified KEY, return True if successful, False if failed
this.remove = function(_key) {
var bln = false;
try {
for (i = 0; i < this.elements.length; i ) {
if (this.elements[i].key == _key) {
this.elements.splice(i, 1 ; , //Get the element value VALUE of the specified KEY, and return NULL
on failure this.get = function(_key) {
{
> } catch (e) {
; (_index) {
if (_index < 0 || _index >= this.elements.length) {
};
//Determine whether the MAP contains the element of the specified KEY
this.containsKey = function(_key) {
var bln = false;
try {
for (i = 0; i < this.elements.length; i ) {
}
} catch (e) {
bln = false; ;
//Determine whether the MAP contains the element of the specified VALUE
this.containsValue = function(_value) {
var bln = false;
try {
for (i = 0; i < this.elements.length; i ) {
}
} catch ( e) {
}
Return bln;
};
// Get the array of all values in the map (🎜> this.values = function () {
var arr = new Array();
for (i = 0; i < this.elements.length; i ) {
arr.push(this.elements[i].value);
}
}; return arr;
};
//Get the array (ARRAY) of all KEYs in MAP
this.keys = function() {
var arr = new Array( );
for (i = 0; i < this.elements.length; i ) {
arr.push(this.elements[i].key);
}
return arr;
};
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


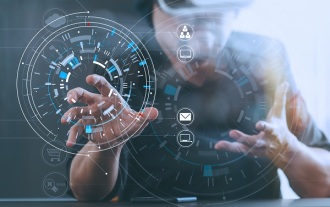
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
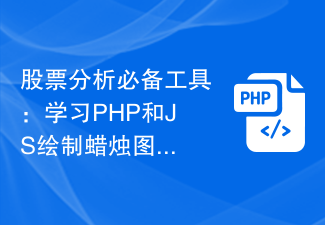
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
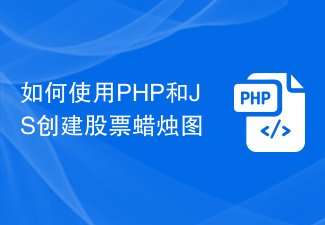
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
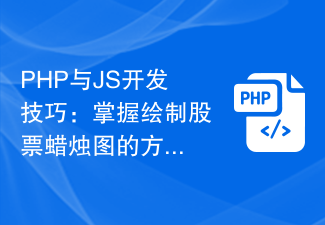
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
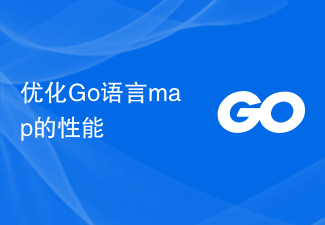
Optimizing the performance of Go language map In Go language, map is a very commonly used data structure, used to store a collection of key-value pairs. However, map performance may suffer when processing large amounts of data. In order to improve the performance of map, we can take some optimization measures to reduce the time complexity of map operations, thereby improving the execution efficiency of the program. 1. Pre-allocate map capacity. When creating a map, we can reduce the number of map expansions and improve program performance by pre-allocating capacity. Generally, we
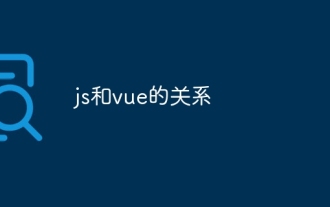
The relationship between js and vue: 1. JS as the cornerstone of Web development; 2. The rise of Vue.js as a front-end framework; 3. The complementary relationship between JS and Vue; 4. The practical application of JS and Vue.
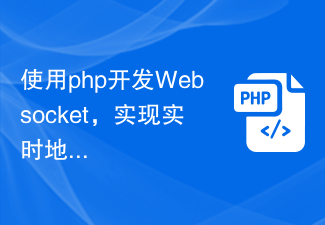
Title: Using PHP to develop Websocket to implement real-time map positioning function Introduction: Websocket is a protocol that implements persistent connections and real-time two-way communication, and can achieve real-time data transmission and updates. This article will use PHP to develop Websocket, combined with the map positioning function, to achieve real-time map positioning function. The specific code implementation process will be introduced in detail below. 1. Preparation: Install PHP environment (version requirement: PHP5.3.0+) Install Composer (PHP third party
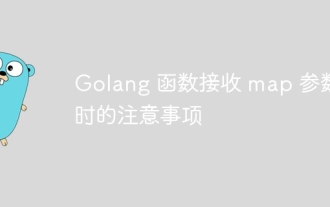
When passing a map to a function in Go, a copy will be created by default, and modifications to the copy will not affect the original map. If you need to modify the original map, you can pass it through a pointer. Empty maps need to be handled with care, because they are technically nil pointers, and passing an empty map to a function that expects a non-empty map will cause an error.
