


javascript-decomposition of simple calculator implementation steps (with pictures)_javascript skills
1. Use of mathematical operations ", -, *, /"
2. Judgment of input content, judgment of the source of event objects
Effect :
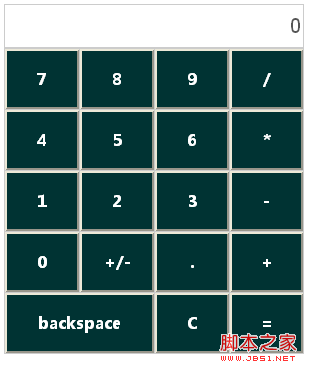
Code:
View Code
Detailed breakdown:
First: The branch calculation part does not use switch statements, but uses the form of name-value pairs.
//Calculation object
var operateExp={
' ':function(num1,num2){return num1 num2;},
'-':function(num1,num2){return num1-num2;},
'*':function(num1, num2){return num1*num2;},
'/':function(num1,num2){return num2===0?0:num1/num2;}
}
Second: Use the properties of the object event to obtain the type of the clicked object. Use event bubbling to capture events and classify them.
calculate_num.onclick=function(e){
var ev = e || window.event;
var target = ev.target || ev.srcElement;
if(target.type=="button"){
var mark=target.getAttribute( "_type"); //Get the custom attributes of the currently clicked button.
var value=target.value;//Get the current value
var num=getScreen();//Get the value of the current box
if(mark==='bs'){//Exit Square key
if(num==0)return;
var snum=Math.abs(num).toString();
if(snum.length<2)
setScreen(0);
else
setScreen(num.toString().slice(0,-1));
}
if(mark==='num'){//numeric key
if (num==='0'||isReset){//There is an operator or the display is 0
setScreen(value);
isReset=false;
return;
}
setScreen(num.toString().concat(value));
}
if(mark==="."){//Decimal point
var hasPoint=num.toString().indexOf(" .")>-1;
if(hasPoint){
if(isReset){
setScreen("0" value);
isReset=false;
return;
}
return;
}
setScreen(num.toString().concat(value));
}
if(mark===" /-"){//Positive and negative No.
setScreen(-num);
}
if(mark==="op"){//If the click is an operator, design the first operand
if(isReset) return;
isReset=true;
if(!operation){
result= num;
operation=value;
return;
}
result=operateNum(result, num,operation);
setScreen(result);
operation=value;
}
if(mark==="cls"){//Clear
result=0;
setScreen(result);
isReset=false;
}
if(mark==="eval"){//Calculate
if(!operation)return;
result= operateNum(result,num,operation);
setScreen(result);
operation=null;
isReset=false;
}
}
}
Third: Use of global variables, use global variables to control the progress of local operations. (State control)
var result=0;//Calculation Result
var isReset=false;//Whether to reset
var operation;//Operator
Fourth: Separate and decouple page operations .
//Set the value of the display screen
function setScreen(num){
calculate_outPut.value=num;
}
//Get the value of the display screen
function getScreen (){
return calculate_outPut.value;
}
Fifth: Filter the operands and complete the calculation.
//Calculate function
var operateNum=function( num1,num2,op){
if(!(num1&&num2))return;
//Guarantee that num1 and num2 are both numbers
num1=Number(num1);
num2=Number(num2) ;
//No operator exists, return num1;
if(!op)return num1;
//Matching operation formula
if(!operateExp[op])return 0;
return operateExp[op](num1,num2);
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


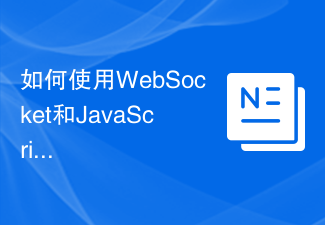
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
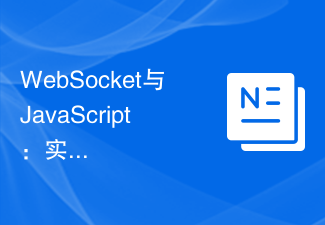
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
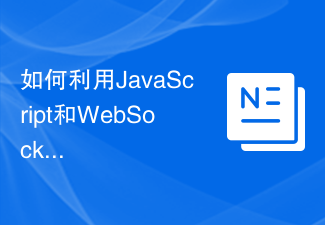
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
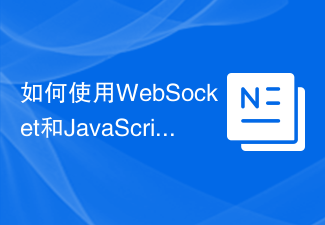
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
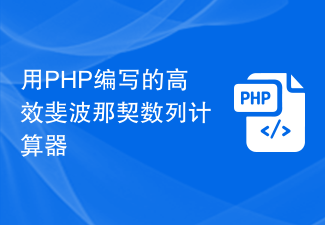
Efficient Fibonacci sequence calculator: PHP implementation of Fibonacci sequence is a very classic mathematical problem. The rule is that each number is equal to the sum of the previous two numbers, that is, F(n)=F(n -1)+F(n-2), where F(0)=0 and F(1)=1. When calculating the Fibonacci sequence, it can be implemented recursively, but performance problems will occur as the value increases. Therefore, this article will introduce how to write an efficient Fibonacci using PHP
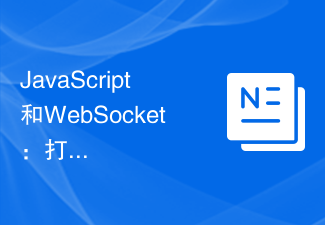
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
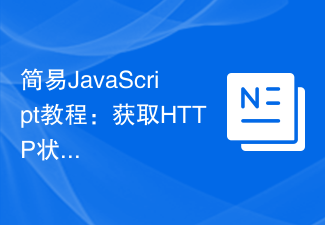
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
