


Some issues you must know about the performance of string concatenation in JavaScript_Basic knowledge
The core of JavaScript is ECMAScript. Similar to other languages, ECMAScript strings are immutable, that is, their values cannot be changed.
Consider the following code:
var str = "hello ";
str = "world";In fact, the steps performed by this code behind the scenes are as follows:
1. Create the character that stores "hello " string.
2. Create a string to store "world".
3. Create a string to store the connection result.
4. Copy the current content of str to the result.
5. Copy "world" into the result.
6. Update str so that it points to the result.
Steps 2 to 6 are performed every time string concatenation is completed, making this operation very resource intensive. If this process is repeated hundreds, or even thousands, of times, it can cause performance problems. The solution is to use an Array object to store the string and then use the join() method (parameter is an empty string) to create the final string. Imagine replacing the previous code with the following code:
var arr = new Array();
arr[0] = "hello ";
arr[1] = "world";
var str = arr.join("");
In this way, no matter how many strings are introduced into the array, it will not be a problem, because the join operation only occurs when the join() method is called. At this point, the steps to perform are as follows:
1. Create the strings to store the results
2. Copy each string to the appropriate location in the result
While this solution is good, there is a better way. The problem is, this code doesn't reflect exactly what it's intended to do. To make it easier to understand, you can wrap the functionality with the StringBuffer class:
function StringBuffer () {
this._strings_ = new Array();
}
StringBuffer.prototype.append = function(str) {
this._strings_.push(str);
};
StringBuffer.prototype.toString = function() {
return this._strings_.join("");
};
The first thing to pay attention to in this code is strings Attributes are meant to be private attributes. It has only two methods, namely append() and toString() methods. The append() method has a parameter, which appends the parameter to the string array. The toString() method calls the join method of the array and returns the actual concatenated string. To concatenate a set of strings using StringBuffer objects, you can use the following code:
var buffer = new StringBuffer ();
buffer.append("hello ");
buffer.append("world");
var result = buffer.toString();
Based on the above implementation, let’s compare the running time, that is, use " " to connect strings and our encapsulated tools one by one. The following code can be used to test the performance of StringBuffer objects and traditional string concatenation methods. Enter the code in the chrome console and run:
var d1 = new Date();
var str = "";
for (var i=0; i < 10000; i ) {
str = "text";
}
var d2 = new Date();
console.log("Concatenation with plus: "
(d2.getTime() - d1.getTime()) " milliseconds");
var buffer = new StringBuffer();
d1 = new Date();
for (var i=0; i < 10000; i ) {
buffer.append("text") ;
}
var result = buffer.toString();
d2 = new Date();
console.log("Concatenation with StringBuffer: "
(d2.getTime() - d1.getTime()) " milliseconds");
This code performs two tests for string concatenation, the first using the plus sign and the second using the StringBuffer class. Each operation concatenates 10,000 strings. The date values d1 and d2 are used to determine how long it takes to complete the operation. Please note that when creating a Date object without parameters, the current date and time are assigned to the object. To calculate how long the join operation took, subtract the millisecond representation of the date (using the return value of the getTime() method). This is a common way to measure JavaScript performance. The results of this test can help you compare the efficiency of using the StringBuffer class versus using the plus sign.
The results of the above example are as follows:
Then some people may say that the String object in JavaScript also encapsulates a concat() method. We will also use the concat() method below to do the same thing. Enter the following code in the consoel:
var d1 = new Date();
var str = "";
for (var i=0; i < 10000; i ) {
str.concat("text");
}
var d2 = new Date();
console.log("Concatenation with plus: "
(d2.getTime() - d1.getTime()) " milliseconds");
We can see that it is done 10000 times The time it takes to concatenate characters is:
It can be concluded that when it comes to a certain number of string connections, we can improve performance by encapsulating a StringBuffer object (function) similar to Java in Javascript to perform operations.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


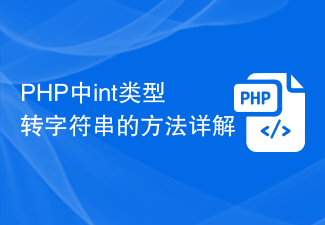
Detailed explanation of the method of converting int type to string in PHP In PHP development, we often encounter the need to convert int type to string type. This conversion can be achieved in a variety of ways. This article will introduce several common methods in detail, with specific code examples to help readers better understand. 1. Use PHP’s built-in function strval(). PHP provides a built-in function strval() that can convert variables of different types into string types. When we need to convert int type to string type,
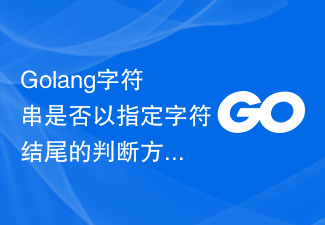
Title: How to determine whether a string ends with a specific character in Golang. In the Go language, sometimes we need to determine whether a string ends with a specific character. This is very common when processing strings. This article will introduce how to use the Go language to implement this function, and provide code examples for your reference. First, let's take a look at how to determine whether a string ends with a specified character in Golang. The characters in a string in Golang can be obtained through indexing, and the length of the string can be
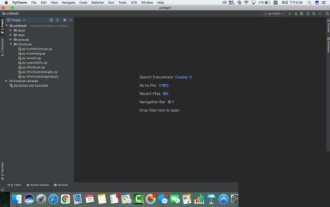
1. First open pycharm and enter the pycharm homepage. 2. Then create a new python script, right-click - click new - click pythonfile. 3. Enter a string, code: s="-". 4. Then you need to repeat the symbols in the string 20 times, code: s1=s*20. 5. Enter the print output code, code: print(s1). 6. Finally run the script and you will see our return value at the bottom: - repeated 20 times.
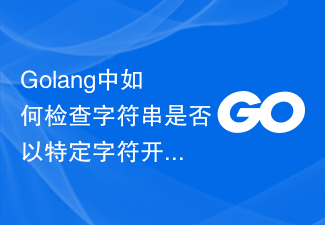
How to check if a string starts with a specific character in Golang? When programming in Golang, you often encounter situations where you need to check whether a string begins with a specific character. To meet this requirement, we can use the functions provided by the strings package in Golang to achieve this. Next, we will introduce in detail how to use Golang to check whether a string starts with a specific character, with specific code examples. In Golang, we can use HasPrefix from the strings package
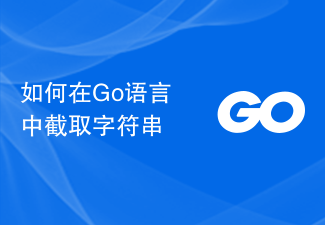
Go language is a powerful and flexible programming language that provides rich string processing functions, including string interception. In the Go language, we can use slices to intercept strings. Next, we will introduce in detail how to intercept strings in Go language, with specific code examples. 1. Use slicing to intercept a string. In the Go language, you can use slicing expressions to intercept a part of a string. The syntax of slice expression is as follows: slice:=str[start:end]where, s
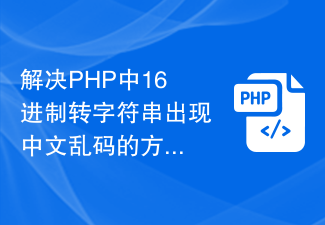
Methods to solve Chinese garbled characters when converting hexadecimal strings in PHP. In PHP programming, sometimes we encounter situations where we need to convert strings represented by hexadecimal into normal Chinese characters. However, in the process of this conversion, sometimes you will encounter the problem of Chinese garbled characters. This article will provide you with a method to solve the problem of Chinese garbled characters when converting hexadecimal to string in PHP, and give specific code examples. Use the hex2bin() function for hexadecimal conversion. PHP’s built-in hex2bin() function can convert 1
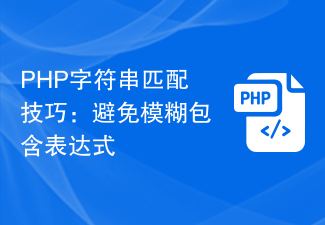
PHP String Matching Tips: Avoid Ambiguous Included Expressions In PHP development, string matching is a common task, usually used to find specific text content or to verify the format of input. However, sometimes we need to avoid using ambiguous inclusion expressions to ensure match accuracy. This article will introduce some techniques to avoid ambiguous inclusion expressions when doing string matching in PHP, and provide specific code examples. Use preg_match() function for exact matching In PHP, you can use preg_mat
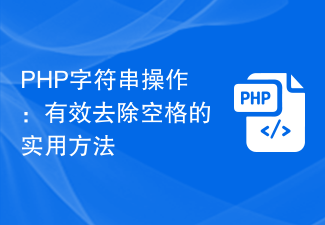
PHP String Operation: A Practical Method to Effectively Remove Spaces In PHP development, you often encounter situations where you need to remove spaces from a string. Removing spaces can make the string cleaner and facilitate subsequent data processing and display. This article will introduce several effective and practical methods for removing spaces, and attach specific code examples. Method 1: Use the PHP built-in function trim(). The PHP built-in function trim() can remove spaces at both ends of the string (including spaces, tabs, newlines, etc.). It is very convenient and easy to use.
