


Analysis and comparison of js objects and print objects_javascript skills
JS object introduction:
1. Basic concepts
1, Custom object.
According to the object extension mechanism of JS, users can customize JS objects, which is similar to the Java language.
Corresponding to custom objects are JS standard objects, such as Date, Array, Math, etc.
2. Prototype
In JS, this is a way to create object properties and methods. New properties and methods can be added to objects through prototype.
Through prototype we can add new properties and methods to JS standard objects, for example, for String objects, we can add a new method trim().
Unlike strict programming languages such as Java, we can dynamically add new properties to JS objects during runtime.
2. Grammar rules
1, object creation method
1) Object initializer method
Format: objectName = {property1:value1, property2:value2,…, propertyN:valueN}
property is the property of the object
value is the value of the object. The value can be a string, a number or an object. One of them
For example: var user={name:“user1”,age:18};
var user={name:“user1”,job:{salary:3000,title:programmer}
The method of the object can also be initialized in this way, for example:
var user={name:"user1",age:18,getName:function(){
> }
The following will focus on the constructor method, including the definition of attributes and methods, etc., and also explain the constructor method.
2) Constructor method
Write a constructor and create the object through new. The constructor can have construction parameters
For example:this.age=age;
this.canFly= false;
}
var use=new User();
2, define object properties
1) Three types of properties can be defined for objects in JS: private properties, instance properties and class properties. Similar to Java, private properties can only be used inside the object, and instance properties must be referenced through the instance of the object. Class attributes can be referenced directly by the class name.
2) Private property definition
Private properties can only be defined and used inside the constructor.Syntax format: var propertyName=value;
For example:
var isChild=age<12;
this.isLittleChild=isChild;
}
var user=new User (15);
alert(user.isLittleChild);//The correct way
alert(user.isChild);//Error: The object does not support this property or method
3 ) instance attribute definition, there are also two ways:
this method, syntax format: this.propertyName=value, pay attention to the position of this in the following examples
The value above can be characters, numbers and objects.
For example:
User.prototype.age=18;
var user=new User();
alert(user.age);
———— ——————————–
function User(name,age,job){
this.name="user1";
this.age=18;
this.job =job;
}
alert(user.age);
3) Class attribute definition
For example:
User.MIN_AGE=0;
alert(User.MAX_AGE);
Refer to the class attributes of JS standard objects:
Number.MAX_VALUE //Maximum numerical value Math.PI //Pi
4) For the definition of attributes, in addition to the more formal way above, there is also a very special way of definition, the syntax format: obj[index]=value
Example:
function User(name){
this.name=name;
this.age =18;
this[1]=“ok”;
this[200]=“year”;
}
var user=new User(“user1”);
alert( user[1]);
In the above example, please note: the age attribute cannot be obtained through this[1], and the name attribute cannot be obtained through this[0], that is, through the index method Those defined must be referenced using the index method, and those defined without the index method must be referenced in the normal way
3. Define object methods
1) Three types of methods can be defined for objects in JS: private methods, instance methods and class methods, similar to Java:
Private methods can only be used inside the object
Instance methods must be in the object instance
Class methods can be used directly through the class name.
Note: The method cannot be defined through the index method mentioned above.
2) Define private methods
Private methods must be defined within the constructor body and can only be used within the constructor body.
Syntax format: function methodName(arg1,…,argN){ }
For example:
function User(name){
this.name=name; This .nameLength=getNameLength(this.name);
}
3) Define instance methods. Currently, you can use two methods:
prototype method, used outside the constructor, syntax Format:
functionName.prototype.methodName=method;
functionName.prototype.methodName=function(arg1,…,argN){};
This method is used inside the constructor, syntax Format:
this.methodName=method;
or
this.methodName=function(arg1,…,argN){};
In the above syntax description, method is an external method that already exists , the method of the object to be defined by methodName means directly assigning an external method to a certain method of the object.
Defining object methods in the form of function(arg1,…,argN){} is what developers should master.
Some examples of defining instance methods: Example 1
Copy the code
}
function getUserName(){
return this .name;
}
Function setUserName(name){
this.name=name;
}
Some examples of defining instance methods: Example 2
Copy code
};
this.setName=function(newName){
this.name=newName;
};
}
Some examples of defining instance methods: Example 3
Copy code
function User(name){
this.name=name;
}
User.prototype.getName=getUserName;
User.prototype.setName=setUserName();
function getUserName(){
return this.name;
}
Function setUserName(name){
this.name=name;
}
Some examples of defining instance methods: Example 4
function User( name){
this.name=name;
}
User.prototype.getName=function(){
return this.name;
};
User.prototype.setName =function(newName){
this.name=newName;
};
4) Define class methods
Class methods need to be defined outside the constructor and can be directly constructed through The function name refers to it.
Syntax format:
functionName.methodName=method;
or
functionName.methodName=function(arg1,…,argN){};
Example:
function User(name){
this.name=name;
}
User.getMaxAge=getUserMaxAge;
function getUserMaxAge(){
return 200;
}
or
User.getMaxAge=function(){return 200;};
alert (User.getMaxAge());
4, references to properties and methods
1) In terms of visibility:
Private properties and methods can only be referenced within the object.
Instance properties and methods can be used anywhere, but must be referenced through objects.
Class attributes and methods can be used anywhere, but cannot be referenced through instances of objects (this is different from Java, where static members can be accessed through instances).
2) From the object level:
is similar to Java bean references and can be referenced in depth.
Several ways:
Simple properties: obj.propertyName
Object properties: obj.innerObj.propertyName
Index properties: obj.propertyName[index]
For deeper references, similar to above .
3) In terms of definition:
Attributes defined through index must be referenced through index.
Attributes defined through non-index methods must be referenced through normal methods.
Also note: Object methods cannot be defined through index.
5. Dynamic addition and deletion of attributes and methods
1) For an instantiated object, we can dynamically add and delete its attributes and methods. The syntax is as follows (assuming the object instance is obj):
Dynamically add object properties
obj.newPropertyName=value;
Dynamicly add object methods
obj.newMethodName=method or =function(arg1,…,argN){}
Dynamicly delete object properties
delete obj.propertyName
Dynamic deletion of object method
delete obj.methodName
2) Example:
function User(name){
this.name=name;
this.age=18;
}
var user= new User(“user1”);
user.sister=“susan”;
alert(user.sister);//Run through
delete user.sister;
alert(user.sister) ;//Error: The object does not support this attribute
user.getMotherName=function(){return “mary”;}
alert(user.getMotherName());//Run through
delete user.getMotherName;
alert(user.getMotherName( ));//Error: The object does not support this method
JS object printing:

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
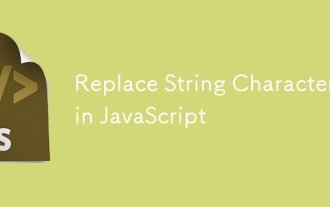
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
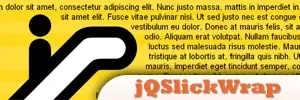
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
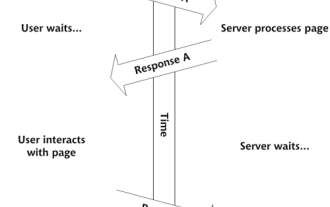
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
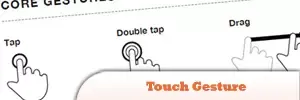
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
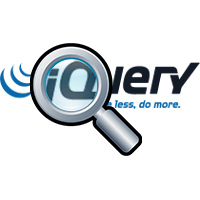
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
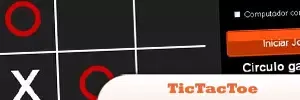
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
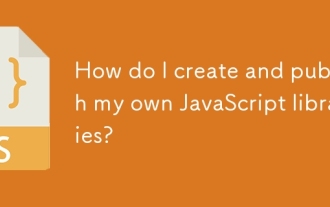
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
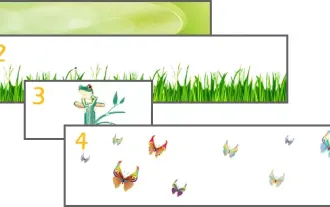
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
