


5 implementation methods and comparisons of js removing spaces before and after strings_javascript skills
If we write in the project that when the user enters spaces during registration, how do we remove the spaces?
The following is the js I often use to share with you:
The first type: Loop check and replace
[javascript]
//For users to call
function trim(s){
return trimRight(trimLeft(s));
}
//Remove the left blank
function trimLeft( s){
if(s == null) {
return "";
}
var whitespace = new String(" tnr");
var str = new String(s) ;
if (whitespace.indexOf(str.charAt(0)) != -1) {
var j=0, i = str.length;
while (j < i && whitespace.indexOf (str.charAt(j)) != -1){
j ;
}
str = str.substring(j, i);
}
return str;
}
//Remove the whitespace on the right www.jb51.net
function trimRight(s){
if(s == null) return "";
var whitespace = new String(" tnr" );
var str = new String(s);
if (whitespace.indexOf(str.charAt(str.length-1)) != -1){
var i = str.length - 1;
while (i >= 0 && whitespace.indexOf(str.charAt(i)) != -1){
i--;
}
str = str.substring( 0, i 1);
}
return str;
}
Second: regular replacement
[javascript]
Third method: use jquery
[javascript]
$.trim(str)
The internal implementation of jquery is:
[javascript]
function trim(str){
return str.replace(/^(s|u00A0) / ,'').replace(/(s|u00A0) $/,'');
}
The fourth way: use motorcycles
[javascript ]
function trim(str){
return str .replace(/^(s|xA0) |(s|xA0) $/g, '');
}
The fifth way: cutting strings
[javascript]
function trim(str) {
str = str.replace(/^(s|u00A0) /,'');
for(var i=str.length-1; i>=0; i--){
if(/S/.test(str.charAt(i))){
str = str.substring(0, i 1);
break;
}
}
return str ;
}
After testing, the fifth method is the most efficient when processing long strings.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


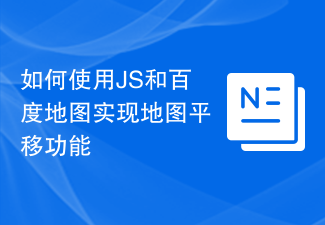
How to use JS and Baidu Map to implement map pan function Baidu Map is a widely used map service platform, which is often used in web development to display geographical information, positioning and other functions. This article will introduce how to use JS and Baidu Map API to implement the map pan function, and provide specific code examples. 1. Preparation Before using Baidu Map API, you first need to apply for a developer account on Baidu Map Open Platform (http://lbsyun.baidu.com/) and create an application. Creation completed
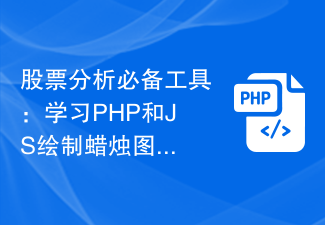
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
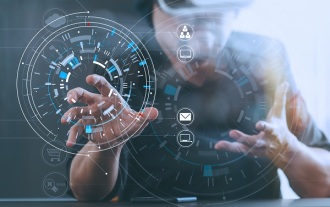
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
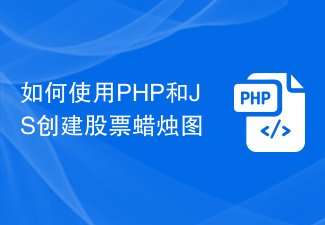
How to use PHP and JS to create a stock candle chart. A stock candle chart is a common technical analysis graphic in the stock market. It helps investors understand stocks more intuitively by drawing data such as the opening price, closing price, highest price and lowest price of the stock. price fluctuations. This article will teach you how to create stock candle charts using PHP and JS, with specific code examples. 1. Preparation Before starting, we need to prepare the following environment: 1. A server running PHP 2. A browser that supports HTML5 and Canvas 3
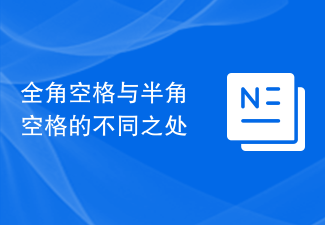
The difference between full-width spaces and half-width spaces. When we use word processing software or edit text content, we sometimes encounter the concept of spaces. Space is a very basic element in typesetting and formatting text, but many people may not know the difference between full-width spaces and half-width spaces. In daily use, we may feel that full-width spaces and half-width spaces have different effects in different situations, but we may not be aware of the subtle differences. First of all, the difference between full-width spaces and half-width spaces is the width they occupy.
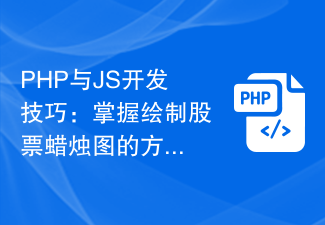
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
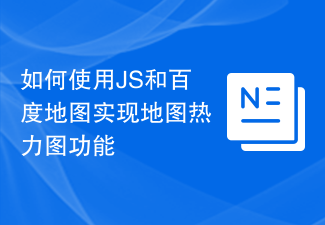
How to use JS and Baidu Maps to implement the map heat map function Introduction: With the rapid development of the Internet and mobile devices, maps have become a common application scenario. As a visual display method, heat maps can help us understand the distribution of data more intuitively. This article will introduce how to use JS and Baidu Map API to implement the map heat map function, and provide specific code examples. Preparation work: Before starting, you need to prepare the following items: a Baidu developer account, create an application, and obtain the corresponding AP
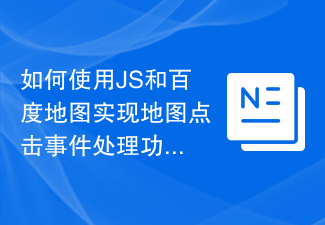
Overview of how to use JS and Baidu Maps to implement map click event processing: In web development, it is often necessary to use map functions to display geographical location and geographical information. Click event processing on the map is a commonly used and important part of the map function. This article will introduce how to use JS and Baidu Map API to implement the click event processing function of the map, and give specific code examples. Steps: Import the API file of Baidu Map. First, import the file of Baidu Map API in the HTML file. This can be achieved through the following code:
