How to configure file upload size limit in Golang project?
Configuring the file upload size limit in the Golang project: Set the MaxFileSize field of the FileHeader type attribute in http.Request. Create a file upload handler and set an upload file size limit. Parse multi-part forms and extract uploaded files. Save the file to disk. Returns a success response or an error response if the file size exceeds the limit.
How to configure file upload size limit in Golang project
In Golang project, configuring upload size limit involves settingMaxFileSize
field, which is a FileHeader type attribute of http.Request
. Here's how to do it:
package main import ( "fmt" "log" "net/http" "os" ) func main() { // 设置上传文件大小限制为 10 MB maxFileSize := int64(10 * 1024 * 1024) // 创建文件上传处理程序 http.HandleFunc("/upload", func(w http.ResponseWriter, r *http.Request) { // 检查请求是否是多部分表单 if r.Method == "POST" && r.MultipartForm == nil { http.Error(w, "请求不是多部分表单", http.StatusBadRequest) return } // 解析多部分表单 if err := r.ParseMultipartForm(maxFileSize); err != nil { http.Error(w, fmt.Sprintf("解析表单时出错:%v", err), http.StatusInternalServerError) return } // 提取上传文件 file, fileHeader, err := r.FormFile("file") if err != nil { http.Error(w, fmt.Sprintf("提取文件时出错:%v", err), http.StatusBadRequest) return } defer file.Close() // 将文件保存到磁盘 dst, err := os.Create("uploaded_file") if err != nil { http.Error(w, fmt.Sprintf("保存文件时出错:%v", err), http.StatusInternalServerError) return } defer dst.Close() if _, err = io.Copy(dst, file); err != nil { http.Error(w, fmt.Sprintf("复制文件时出错:%v", err), http.StatusInternalServerError) return } // 返回成功响应 fmt.Fprintf(w, "文件上传成功!") }) // 启动服务器 log.Fatal(http.ListenAndServe(":8080", nil)) }
Practical example:
Use Postman or cURL to upload a file larger than 10 MB, you will receive an error response as follows Display:
{ "error": "文件大小超出了限制" }
The above is the detailed content of How to configure file upload size limit in Golang project?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


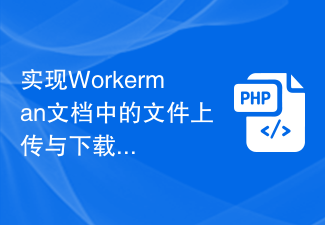
To implement file upload and download in Workerman documents, specific code examples are required. Introduction: Workerman is a high-performance PHP asynchronous network communication framework that is simple, efficient, and easy to use. In actual development, file uploading and downloading are common functional requirements. This article will introduce how to use the Workerman framework to implement file uploading and downloading, and give specific code examples. 1. File upload: File upload refers to the operation of transferring files on the local computer to the server. The following is used
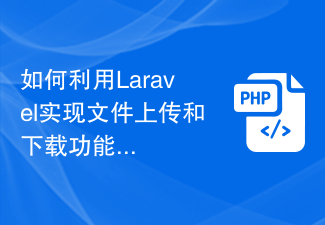
How to use Laravel to implement file upload and download functions Laravel is a popular PHP Web framework that provides a wealth of functions and tools to make developing Web applications easier and more efficient. One of the commonly used functions is file upload and download. This article will introduce how to use Laravel to implement file upload and download functions, and provide specific code examples. File upload File upload refers to uploading local files to the server for storage. In Laravel we can use file upload
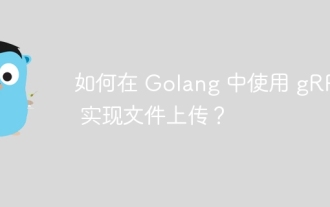
How to implement file upload using gRPC? Create supporting service definitions, including request and response messages. On the client, the file to be uploaded is opened and split into chunks, then streamed to the server via a gRPC stream. On the server side, file chunks are received and stored into a file. The server sends a response after the file upload is completed to indicate whether the upload was successful.
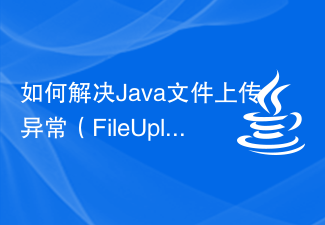
How to solve Java file upload exception (FileUploadException). One problem that is often encountered in web development is FileUploadException (file upload exception). It may occur due to various reasons such as file size exceeding limit, file format mismatch, or incorrect server configuration. This article describes some ways to solve these problems and provides corresponding code examples. Limit the size of uploaded files In most scenarios, limit the file size
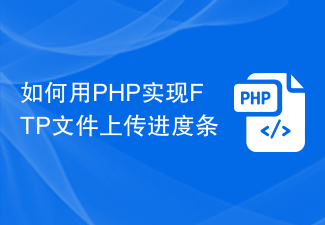
How to use PHP to implement FTP file upload progress bar 1. Background introduction In website development, file upload is a common function. For the upload of large files, in order to improve the user experience, we often need to display an upload progress bar to the user to let the user know the file upload process. This article will introduce how to use PHP to implement the FTP file upload progress bar function. 2. The basic idea of implementing the progress bar of FTP file upload. The progress bar of FTP file upload is usually calculated by calculating the size of the uploaded file and the size of the uploaded file.
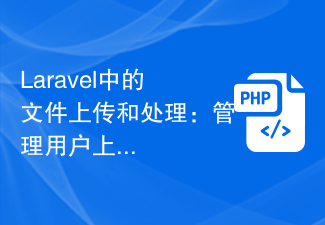
File Uploading and Processing in Laravel: Managing User Uploaded Files Introduction: File uploading is a very common functional requirement in modern web applications. In the Laravel framework, file uploading and processing becomes very simple and efficient. This article will introduce how to manage user-uploaded files in Laravel, including verification, storage, processing, and display of file uploads. 1. File upload File upload refers to uploading files from the client to the server. In Laravel, file uploads are very easy to handle. first,
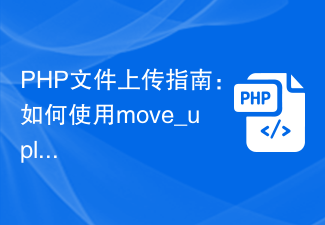
PHP file upload guide: How to use the move_uploaded_file function to handle uploaded files In developing web applications, file upload is a common requirement. PHP provides a convenient function move_uploaded_file() for processing uploaded files. This article will introduce you how to use this function to implement the file upload function. 1. Preparation Before starting, make sure that your PHP environment has been configured with file upload parameters. You can do this by opening php.in
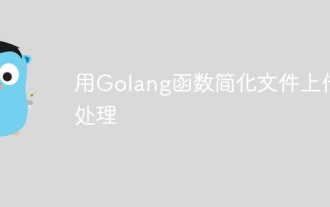
Answer: Yes, Golang provides functions that simplify file upload processing. Details: The MultipartFile type provides access to file metadata and content. The FormFile function gets a specific file from the form request. The ParseForm and ParseMultipartForm functions are used to parse form data and multipart form data. Using these functions simplifies the file processing process and allows developers to focus on business logic.
