How to control the life cycle of Golang coroutines?
Controlling the life cycle of the Go coroutine can be done in the following ways: Create a coroutine: Use the go keyword to start a new task. Terminate coroutines: wait for all coroutines to complete, use sync.WaitGroup. Use channel closing signals. Use context context.Context.
How to control the life cycle of Go coroutines?
In the Go language, coroutines (also known as Goroutines) are lightweight concurrent execution bodies used to perform specific tasks without blocking the main thread. Managing the lifecycle of coroutines is critical to writing robust and maintainable parallel code.
Create a coroutine
Use the go
keyword to create a coroutine. It starts a new task in the background so that the main thread can continue execution.
go func() { // 执行任务 }
Terminating the coroutine
Cannot terminate the coroutine directly, but there are the following methods to achieve this purpose indirectly:
Wait for all coroutines to complete
Use sync.WaitGroup
Synchronization task completed:
import "sync" var wg sync.WaitGroup func main() { for i := 0; i < 5; i++ { wg.Add(1) go func(i int) { // 执行任务 wg.Done() }(i) } wg.Wait() }
Use channel close signal
Use channel to send "close" signal to coroutine:
func main() { done := make(chan struct{}) for i := 0; i < 5; i++ { go func(i int) { for { select { case <-done: return // 协程停止 default: // 执行任务 } } }(i) } close(done) // 向所有协程发送"关闭"信号 }
Use context
Use context.Context
to manage the execution of the coroutine. When the context is canceled, the coroutine will also terminate:
import "context" func main() { ctx, cancel := context.WithCancel(context.Background()) for i := 0; i < 5; i++ { go func(i int) { for { select { case <-ctx.Done(): return // 协程停止 default: // 执行任务 } } }(i) } cancel() // 取消上下文 }
Actual case
The following It is a practical case of using channel closing signal:
package main import "fmt" import "time" func main() { // 使用信道告诉协程何时退出 stop := make(chan struct{}) // 创建 5 个协程 for i := 0; i < 5; i++ { go func(i int) { for { // 检查是否已经收到退出信号 select { case <-stop: fmt.Printf("协程 %d 已退出\n", i) return default: fmt.Printf("协程 %d 正在运行\n", i) time.Sleep(time.Second) } } }(i) } // 运行 5 秒,然后发送退出信号 time.Sleep(time.Second * 5) close(stop) // 等待所有协程退出 time.Sleep(time.Second * 1) }
The above is the detailed content of How to control the life cycle of Golang coroutines?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
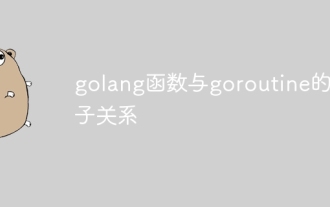
There is a parent-child relationship between functions and goroutines in Go. The parent goroutine creates the child goroutine, and the child goroutine can access the variables of the parent goroutine but not vice versa. Create a child goroutine using the go keyword, and the child goroutine is executed through an anonymous function or a named function. A parent goroutine can wait for child goroutines to complete via sync.WaitGroup to ensure that the program does not exit before all child goroutines have completed.
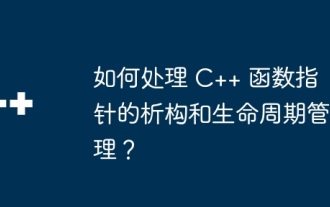
In C++, function pointers require proper destruction and life cycle management. This can be achieved by manually destructing the function pointer and releasing the memory. Use smart pointers, such as std::unique_ptr or std::shared_ptr, to automatically manage the life cycle of function pointers. Bind the function pointer to the object, and the object life cycle manages the destruction of the function pointer. In GUI programming, using smart pointers or binding to objects ensures that callback functions are destructed at the appropriate time, avoiding memory leaks and inconsistencies.
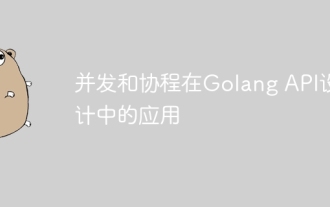
Concurrency and coroutines are used in GoAPI design for: High-performance processing: Processing multiple requests simultaneously to improve performance. Asynchronous processing: Use coroutines to process tasks (such as sending emails) asynchronously, releasing the main thread. Stream processing: Use coroutines to efficiently process data streams (such as database reads).
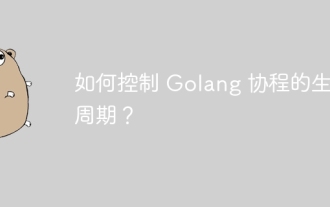
Controlling the life cycle of a Go coroutine can be done in the following ways: Create a coroutine: Use the go keyword to start a new task. Terminate coroutines: wait for all coroutines to complete, use sync.WaitGroup. Use channel closing signals. Use context context.Context.
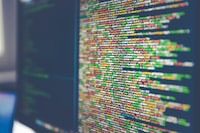
Concurrent and Asynchronous Programming Concurrent programming deals with multiple tasks executing simultaneously, asynchronous programming is a type of concurrent programming in which tasks do not block threads. asyncio is a library for asynchronous programming in python, which allows programs to perform I/O operations without blocking the main thread. Event loop The core of asyncio is the event loop, which monitors I/O events and schedules corresponding tasks. When a coroutine is ready, the event loop executes it until it waits for I/O operations. It then pauses the coroutine and continues executing other coroutines. Coroutines Coroutines are functions that can pause and resume execution. The asyncdef keyword is used to create coroutines. The coroutine uses the await keyword to wait for the I/O operation to complete. The following basics of asyncio
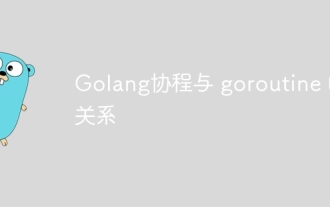
Coroutine is an abstract concept for executing tasks concurrently, and goroutine is a lightweight thread function in the Go language that implements the concept of coroutine. The two are closely related, but goroutine resource consumption is lower and managed by the Go scheduler. Goroutine is widely used in actual combat, such as concurrently processing web requests and improving program performance.
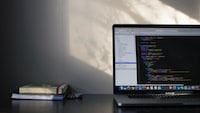
1. Why use asynchronous programming? Traditional programming uses blocking I/O, which means that the program waits for an operation to complete before continuing. This may work well for a single task, but may cause the program to slow down when processing a large number of tasks. Asynchronous programming breaks the limitations of traditional blocking I/O and uses non-blocking I/O, which means that the program can distribute tasks to different threads or event loops for execution without waiting for the task to complete. This allows the program to handle multiple tasks simultaneously, improving the program's performance and efficiency. 2. The basis of Python asynchronous programming The basis of Python asynchronous programming is coroutines and event loops. Coroutines are functions that allow a function to switch between suspending and resuming. The event loop is responsible for scheduling
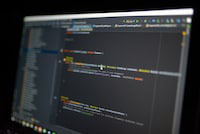
Asynchronous programming, English Asynchronous Programming, means that certain tasks in the program can be executed concurrently without waiting for other tasks to complete, thereby improving the overall operating efficiency of the program. In Python, the asyncio module is the main tool for implementing asynchronous programming. It provides coroutines, event loops, and other components required for asynchronous programming. Coroutine: Coroutine is a special function that can be suspended and then resumed execution, just like a thread, but a coroutine is more lightweight and consumes less memory than a thread. The coroutine is declared with the async keyword and execution is suspended at the await keyword. Event loop: Event loop (EventLoop) is the key to asynchronous programming
