Challenges and strategies for testing multi-threaded programs in C++
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools such as valgrind to find potential data races. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
Challenges and Strategies for C++ Multi-Threaded Program Testing
Challenge:
- Non-repeatability: The behavior of multi-threaded programs may vary due to thread scheduling and data races.
- Concurrency error (data race): When multiple threads access shared data at the same time, data may be inconsistent.
- Deadlock: Threads wait for each other for resources, causing the system to stop.
- Lack of visibility: трудно отслеживать состояние многопоточных программ во время выполнения.
##Strategy:
1. Unit testing
- #Write unit tests for each thread or a group of threads.
- Use assertions and mocks to verify thread behavior.
- For example, you can test whether a thread completes its task within a specified time.
2. Multi-thread simulation
- Use a multi-thread simulation framework (such as gtest, Catch2, cppunit) to test multi-threaded programs.
- Create a simulation environment and control thread scheduling.
- For example, data races can be deliberately introduced in a simulated environment to test whether the program handles them correctly.
3. Data race detection
- Use data race detection tools (such as valgrind, helgrind, sanitizers) to find potential data races state.
- These tools can detect situations where multiple threads are accessing shared data simultaneously.
- For example, valgrind can detect unprotected access to global variables.
4. Debugging
- Use a debugger (such as gdb, lldb, MSVC debugger) to check the status of the multi-threaded program at runtime.
- You can use breakpoints, watchpoints, and single-step execution to track execution.
- For example, you can set breakpoints when a data race occurs to find out the source of the problem.
Practical case:
Consider a multi-threaded program containing three threads:- Thread 1: Read shared data and update.
- Thread 2: Write shared data.
- Thread 3: Polling for changes to shared data.
Testing strategy:
- Unit testing: Test each thread for correct behavior.
- Multi-threaded simulation: Create data races in a simulated environment and verify that the program handles them correctly.
- Data race detection: Use the valgrind analysis program to find potential data races.
- Debugging: Use gdb to check program status when a data race occurs.
The above is the detailed content of Challenges and strategies for testing multi-threaded programs in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


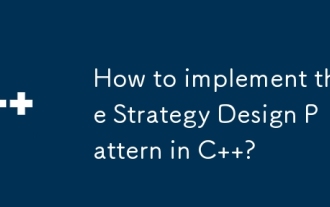
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
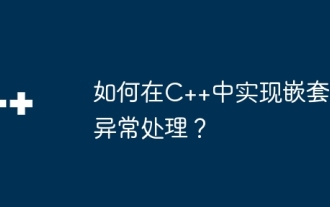
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
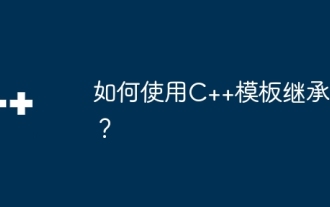
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
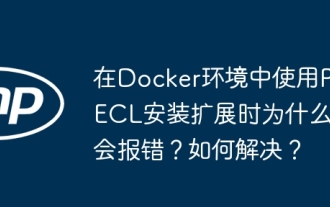
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
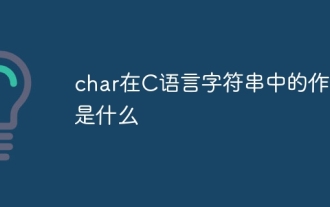
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
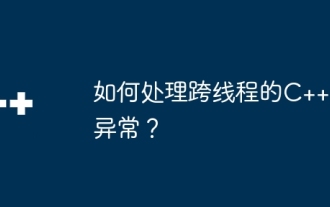
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
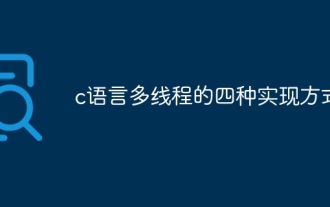
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
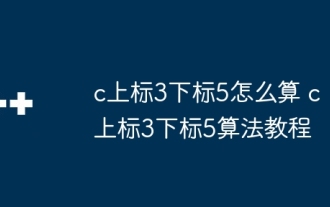
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
