


The difference between struct pointer and struct value transfer in Golang functions
In Go, function parameters can be passed by value or pointer. Value passing passes a copy, and modifications to the copy will not affect the original value; pointer passing passes the address, and modifications to the content will be reflected in the original value. In practice, pointer passing can be used to optimize code, for example when sorting slices. Choosing the right delivery method is critical to optimizing your code and improving performance.
Function parameter passing in Go: value passing and pointer passing
In Go, function parameters can be passed by value or pointer Delivery by delivery. Understanding the difference between these two delivery methods is crucial to mastering Go programming.
Value passing
Value passing means that the function receives a copy of the incoming parameter value. Any modification to the parameter value will not affect the original value. For example:
1 2 3 4 5 6 7 8 9 |
|
In the changeValue
function, s
is a copy of the string
type. Modifications to s
will not affect the original s
variables in the main
function.
Pointer passing
Pointer passing refers to the address of the function receiving the incoming parameters. Any modifications to the parameter content will be reflected in the original variable. For example:
1 2 3 4 5 6 7 8 9 |
|
In the changePointer
function, s
is a pointer to the original string
variable s
. Modifications to *s
will directly modify the original s
variable in the main
function.
Practical case
The following is an example of using pointer passing to optimize code in a real scenario:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
In this example, ## The #sortByName function receives a pointer to a
Person slice. By passing in a pointer, this function can directly modify the contents of the slice without creating a copy. This improves the efficiency of the function, especially when the slices are large.
Conclusion
Understanding the difference between passing by value and passing by pointer is crucial to writing Go programs efficiently. Passing by value is suitable when a copy needs to be modified rather than the original value, while passing by pointer is suitable when the original value needs to be modified. Choosing the correct delivery method for your needs can optimize your code and improve your program's performance.The above is the detailed content of The difference between struct pointer and struct value transfer in Golang functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
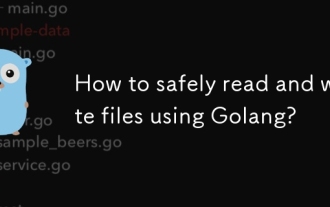
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
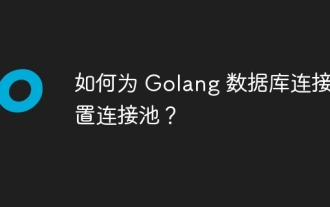
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
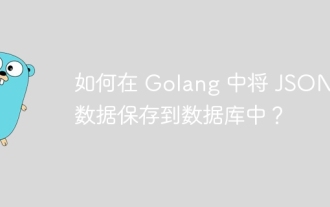
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
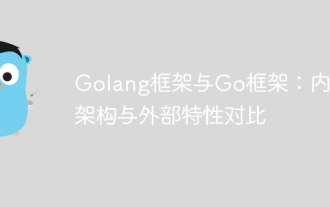
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
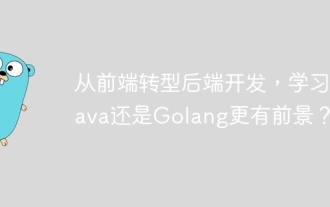
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
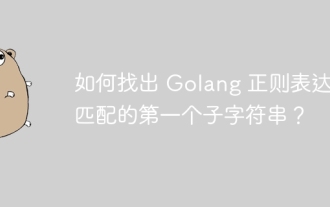
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
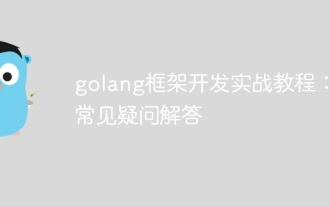
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
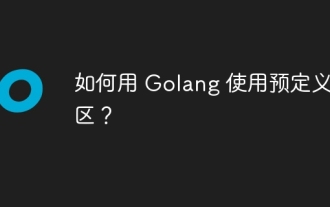
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
