


In-depth understanding of PHP object-oriented programming: interaction between classes and objects
Object-oriented programming (OOP) is a programming paradigm that encapsulates data and behavior in objects to represent real-world entities. In PHP, OOP allows the creation of classes and objects to represent entities in the real world: Classes: Define the data (properties) and operations (methods) of an object. Object: An instance of a class that contains the properties and methods of that class and can interact with other objects. OOP practical example: A shopping cart contains a series of products, modeled by the following two classes: Product: Represents a single product, with a name and price. Cart: Represents a shopping cart, containing a list of products and providing methods for adding products and calculating the total price.
PHP In-depth understanding of object-oriented programming: interaction between classes and objects
What is object-oriented programming?
Object-oriented programming (OOP) is a programming paradigm that encapsulates data and behavior in objects. In PHP, OOP allows us to create classes and objects to represent entities in the real world.
Classes and Objects
- Class: A class is a blueprint for a group of objects with common characteristics and behaviors. It defines the object's data (properties) and operations (methods).
- Object: An object is an instance of a class. It contains the properties and methods of the class and can interact with other objects.
Class definition
class Person { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } public function setName($name) { $this->name = $name; } public function getAge() { return $this->age; } public function setAge($age) { $this->age = $age; } }
Object creation
To create a human object, we can use The following code:
$person = new Person('John Doe', 30);
Accessing properties and methods
We can use the ->
operator to access the properties and methods of an object:
echo $person->getName(); // 输出:"John Doe" $person->setAge(35);
Practical Case: Shopping Cart
Consider an example of a shopping cart that contains a list of products:
class Product { private $name; private $price; public function __construct($name, $price) { $this->name = $name; $this->price = $price; } // ... } class Cart { private $products = []; public function addProduct(Product $product) { $this->products[] = $product; } public function getTotalPrice() { $totalPrice = 0; foreach ($this->products as $product) { $totalPrice += $product->getPrice(); } return $totalPrice; } // ... }
We can use these classes to create a shopping cart And add products:
$cart = new Cart(); $product1 = new Product('Apple', 10); $product2 = new Product('Orange', 5); $cart->addProduct($product1); $cart->addProduct($product2); echo $cart->getTotalPrice(); // 输出:"15"
This object-oriented approach allows us to create reusable and maintainable programs to represent and manipulate data and behavior in real-world scenarios.
The above is the detailed content of In-depth understanding of PHP object-oriented programming: interaction between classes and objects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


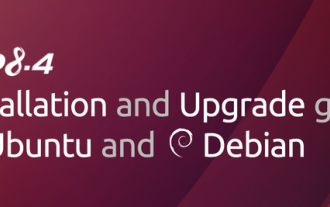
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
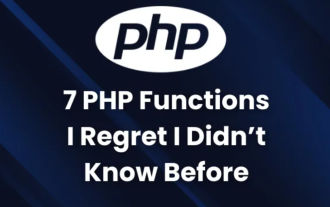
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
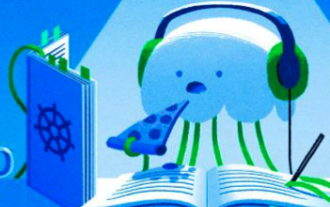
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
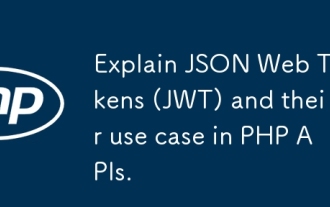
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
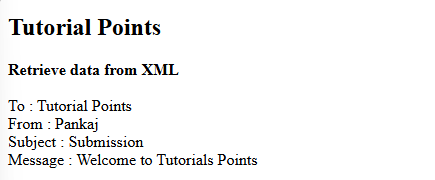
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
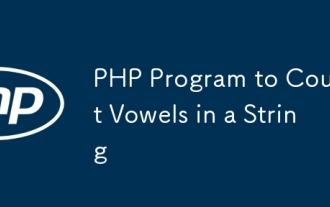
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
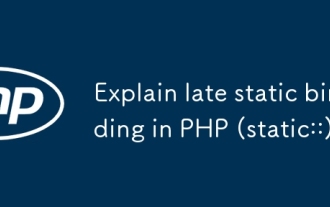
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
