The application practice of Golang in game development
Golang is favored in game development for its concurrency features (goroutines and channels), which provide high concurrency. In addition, it has a powerful network connection library for establishing and managing network connections. Golang's build toolset (Go Compiler, Go Test, Go Build) simplifies the game development process. The practical case (Snake Game) shows Golang in action in game development, using concurrent programming and network connectivity to create highly responsive and maintainable game applications.
The application practice of Golang in game development
##ForewordGolang (also known as Go ) is an open source programming language that has become popular among game developers since its launch in 2009 for its high concurrency, rapid development, and powerful concurrency features. This article will explore the specific application of Golang in game development and demonstrate its advantages through practical cases.
Concurrency Programming Concurrency is a key aspect of game development as it involves handling multiple tasks occurring simultaneously. Golang provides excellent concurrency support through built-in goroutines and channels. Goroutines are similar to threads in that they are lightweight and can execute simultaneously, while channels are used for communication between goroutines. By leveraging Golang's concurrency features, game developers can create highly responsive, parallel games.
Network connectionNetwork connectivity is critical for multiplayer games and online services. Golang provides a powerful standard library to easily establish and manage network connections. For example, the net package can be used to create server sockets, while the net/http package supports HTTP servers and clients. By using Golang, developers can quickly establish robust network connections to achieve a stable gaming experience.
Build ToolsGolang has a powerful set of build tools that simplifies game development. The tool chain includes:
- Go Compiler: used to compile Go source code into executable files
- Go Test: used to write and run unit tests
- Go Build: for building and packaging applications
Practical Case: Snake GameTo demonstrate the practical application of Golang in game development, let us build a classic snake game.
Code snippet 1: Snake.go
package main import ( "fmt" "time" ) const SIZE = 20 const MOVE_INTERVAL = 100 type Snake struct { bodySegments []*Segment } type Segment struct { x int y int } func main() { snake := NewSnake() go snake.Move() ReadInput() } func (s *Snake) Move() { for { time.Sleep(MOVE_INTERVAL * time.Millisecond) s.bodySegments[len(s.bodySegments)-1].x += 1 for i := len(s.bodySegments) - 1; i > 0; i-- { s.bodySegments[i].x, s.bodySegments[i].y = s.bodySegments[i-1].x, s.bodySegments[i-1].y } Render(s) } } func ReadInput() { for { ch := make(chan string) go ReadKeyPress(ch) select { case key := <-ch: switch key { case "w": // Move up fmt.Println("Move up") case "s": // Move down fmt.Println("Move down") case "a": // Move left fmt.Println("Move left") case "d": // Move right fmt.Println("Move right") } } } }
ConclusionGolang is a popular choice due to its concurrency, network connectivity, and build tool capabilities Ideal for game development. By leveraging its built-in features and powerful libraries, game developers can create efficient, responsive, and maintainable game applications.
The above is the detailed content of The application practice of Golang in game development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
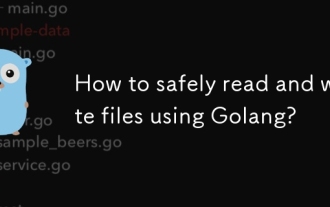
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
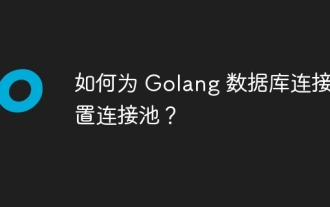
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
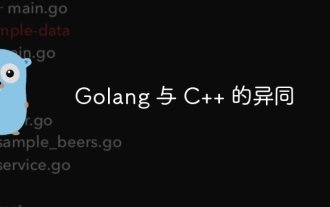
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
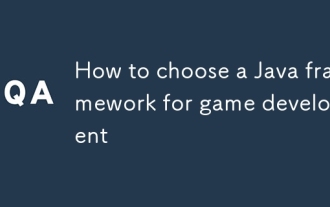
When choosing a Java framework in game development, you should consider the specific needs of your project. Available Java game frameworks include: LibGDX: suitable for cross-platform 2D/3D games. JMonkeyEngine: used to build complex 3D games. Slick2D: Suitable for lightweight 2D games. AndEngine: A 2D game engine developed specifically for Android. Kryonet: Provides network connection capabilities. For 2DRPG games, for example, LibGDX is ideal because of its cross-platform support, lightweight design, and active community.
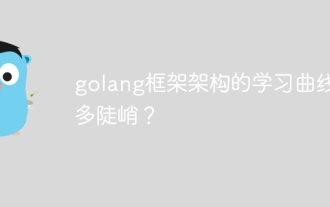
The learning curve of the Go framework architecture depends on familiarity with the Go language and back-end development and the complexity of the chosen framework: a good understanding of the basics of the Go language. It helps to have backend development experience. Frameworks that differ in complexity lead to differences in learning curves.
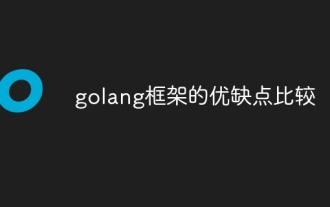
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
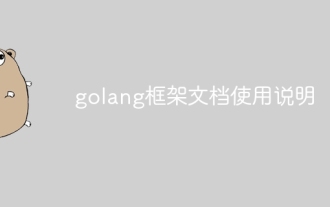
How to use Go framework documentation? Determine the document type: official website, GitHub repository, third-party resource. Understand the documentation structure: getting started, in-depth tutorials, reference manuals. Locate the information as needed: Use the organizational structure or the search function. Understand terms and concepts: Read carefully and understand new terms and concepts. Practical case: Use Beego to create a simple web server. Other Go framework documentation: Gin, Echo, Buffalo, Fiber.
