How to use third-party packages in Go language?
Using third-party packages in Go: Use the go get command to install the package, such as: go get github.com/user/package. Import the package, such as: import ("github.com/user/package"). Example: Use the encoding/json package to parse JSON data: Installation: go get encoding/json Import: import ("encoding/json") Parsing: json.Unmarshal([]byte(jsonString), &data)
How to use third-party packages in Go language
The Go language is famous for its powerful standard library, but sometimes you need to use third-party packages to extend it its function. Third-party packages are externally developed libraries of compiled code that provide a variety of useful functionality.
Install third-party packages
To install third-party packages, you can use the go get
command, followed by the package path:
go get github.com/user/package
This will download and install the specified package in your GOPATH
.
Import package
Once the package is installed, you can import it by using the import
keyword:
import ( "github.com/user/package" )
This This package's code will be imported into your code.
Practical Case: Manipulating JSON Data
Let us use a third-party package to demonstrate the use of third-party packages in the Go language. We use the encoding/json
package to manipulate JSON data.
To install this package, run:
go get encoding/json
Then, import the package:
import ( "encoding/json" )
Now, we can use the encoding/json
package Functions to parse, encode, and decode JSON data. For example, parsing a JSON string:
jsonString := `{"name": "John", "age": 30}` var data map[string]interface{} json.Unmarshal([]byte(jsonString), &data)
data
now contains a map representing the JSON data.
Additional Suggestions
- Make sure the
GOPATH
environment variable is set correctly so that Go can find third-party packages. - Use a version control system to manage third-party package dependencies.
- Check the package's documentation to learn how to use it and what it does.
The above is the detailed content of How to use third-party packages in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
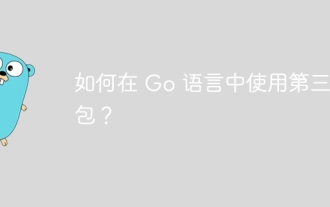
To use third-party packages in Go: Use the goget command to install the package, such as: gogetgithub.com/user/package. Import the package, such as: import("github.com/user/package"). Example: Use the encoding/json package to parse JSON data: Installation: gogetencoding/json Import: import("encoding/json") Parsing: json.Unmarshal([]byte(jsonString),&data)
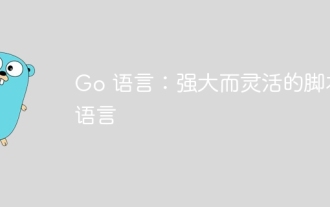
The Go language is a modern open source programming language known for its concurrency support, memory safety, and cross-platform compatibility. It is also an excellent scripting language, providing a rich set of built-in functions and utilities, including: Concurrency support: Simplifies scripting to perform multiple tasks simultaneously. Memory safety: The garbage collector automatically releases unused memory to prevent memory leaks. Cross-platform compatibility: Can be compiled on Windows, Linux, macOS and mobile platforms. Rich standard library: Provides common script functions such as file I/O, network requests, and regular expressions.
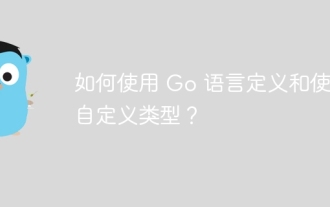
In Go, custom types can be defined using the type keyword (struct) and contain named fields. They can be accessed through field access operators and can have methods attached to manipulate instance state. In practical applications, custom types are used to organize complex data and simplify operations. For example, the student management system uses the custom type Student to store student information and provide methods for calculating average grades and attendance rates.
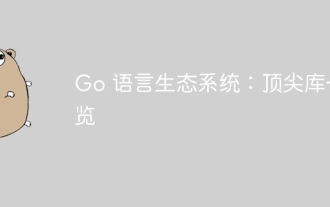
The Go language ecosystem provides a rich and powerful library, including: Gin (a framework for building web applications) Gorm (an ORM for managing database interactions) Zap (for high-performance logging) Viper (for management Application configuration) Prometheus (for monitoring and alerting) These libraries help developers build robust and maintainable Go applications quickly and efficiently.
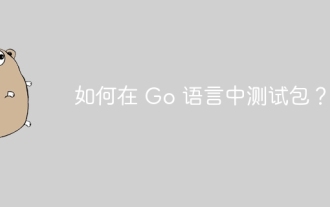
By using the Go language's built-in testing framework, developers can easily write and run tests for their code. The test file ends with _test.go and contains the test function starting with Test, where the *testing.T parameter represents the test instance. Error information is recorded using t.Error(). Tests can be run by running the gotest command. Subtests allow test functions to be broken down into smaller parts and created via t.Run(). The practical case includes a test file written for the IsStringPalindrome() function in the utils package, which uses a series of input strings and expected output to test the correctness of the function.
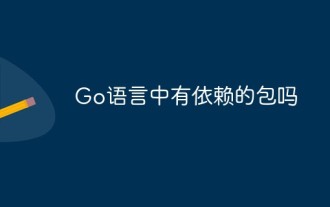
There are dependent packages in the Go language. The methods for installing dependent packages are: 1. Use the "go get" command to install the dependent packages; 2. Enable "go mod", and then use "go get" to pull the package in the project directory; 3. , Manually download the dependency package in github and put it in the corresponding directory; 4. Copy the corresponding package under "GOPATH/pkg/mod"; 5. Put the code directly into the project, and then use "go tidy" to automatically organize the package dependencies That’s it.
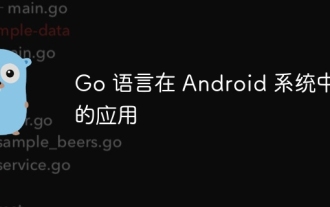
The Go language can be widely used in the Android system and can be used to build AndroidActivities and Services for data processing and analysis, including: using the Go language in AndroidActivity: introducing the Go language library, creating Go language classes, and in the AndroidManifest.xml file Register Go language class. Use Go language in AndroidService: Create a Go language class and register the Go language class in the AndroidManifest.xml file. Use Go language for data processing and analysis: it can be used to build HTTP API, concurrent processing tasks, and encode and decode binary data.
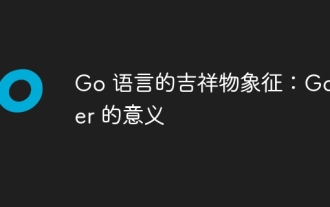
The Go language mascot is a marmot named Gopher, which symbolizes the core principle of the Go language: parallelism: Gopher's digging burrow embodies the concurrency of Go. Simplicity: Gopher's cuteness and simplicity mirror the simplicity of Go. Community: Gopher represents the Go community, a thriving, supportive ecosystem.
