How to use PHP for MySQL data migration?
PHP MySQL Data Migration Guide: Establishing connections to source and target databases. Extract data from the source database. Create a structure in the target database that matches the source table. Migrate data from the source database to the target database row by row using row-by-row insert.
How to use PHP for MySQL data migration
Introduction
Data migration is The important task of moving data from one system to another. When developing applications, it is often necessary to migrate data from a test environment to a production environment. This article will guide you on how to use PHP for MySQL data migration.
Steps
1. Establish a connection
First, you need to connect to the source database and target database:
$sourceConn = new mysqli("localhost", "sourceuser", "sourcepass", "sourcedb"); $targetConn = new mysqli("localhost", "targetuser", "targetpass", "targetdb");
2. Get the source data
Use mysqli_query()
to get the data that needs to be migrated from the source database:
$result = $sourceConn->query("SELECT * FROM `source_table`");
3. Prepare the target table
In the target database, create the target table and match the structure of the source table:
$targetConn->query("CREATE TABLE IF NOT EXISTS `target_table` LIKE `source_table`");
4. Insert data row by row
Loop through the source query results and insert data into the target table row by row:
while ($row = $result->fetch_assoc()) { $insertQuery = "INSERT INTO `target_table` SET "; foreach ($row as $field => $value) { $insertQuery .= "`$field` = '$value', "; } $insertQuery = substr($insertQuery, 0, -2); $targetConn->query($insertQuery); }
Practical case
For example, to users
To migrate tables from development
database to production
database, you can run the following PHP code:
$sourceConn = new mysqli("localhost", "devuser", "devpass", "development"); $targetConn = new mysqli("localhost", "produser", "prodpass", "production"); $result = $sourceConn->query("SELECT * FROM `users`"); $targetConn->query("CREATE TABLE IF NOT EXISTS `users` LIKE `users`"); while ($row = $result->fetch_assoc()) { $insertQuery = "INSERT INTO `users` SET `id` = '{$row['id']}', `name` = '{$row['name']}', `email` = '{$row['email']}'"; $targetConn->query($insertQuery); }
The above is the detailed content of How to use PHP for MySQL data migration?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


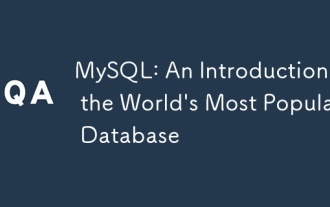
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
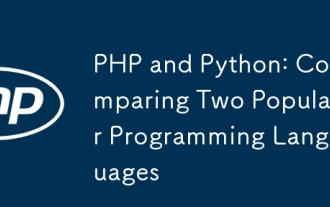
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
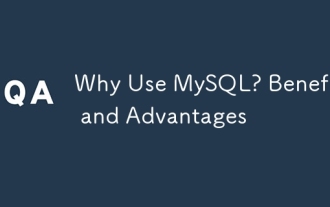
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
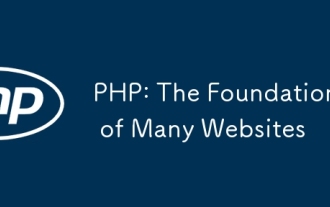
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
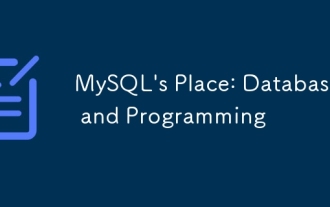
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
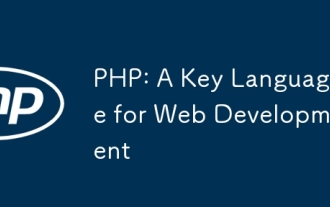
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
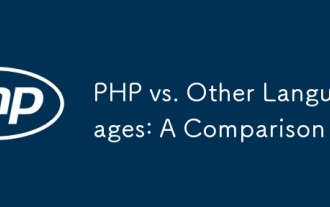
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
