Optimizing C++ server architecture to improve throughput
Optimize C++ server throughput strategy: Thread pool: Create a thread pool in advance to respond to requests quickly. Non-blocking I/O: Perform other tasks while waiting for I/O to improve throughput. HTTP/2: Uses a binary protocol, supports multiplexing and content compression, and improves performance.
Optimizing C++ Server Architecture for Increased Throughput
In modern applications, server throughput is critical. In this article, we'll explore some strategies for optimizing throughput in C++ server applications and provide concrete practical examples.
Thread Pool
Thread pool is a common strategy in asynchronous server design to improve throughput. By pre-creating threads and storing them in a pool, the server can quickly respond to incoming requests without waiting for thread creation.
Practical case:
// 创建线程池 std::shared_ptr<ThreadPool> pool = std::make_shared<ThreadPool>(4); // 处理请求的函数 void handleRequest(std::shared_ptr<Request> request) { // ... } // 主线程循环 while (true) { auto request = server.accept(); if (request) { pool->submit(std::bind(handleRequest, request)); } }
Non-blocking I/O
Non-blocking I/O allows the server to perform other operations while waiting for the I/O operation to complete. Task. This can significantly improve throughput under high concurrency conditions.
Practical case:
// 创建非阻塞套接字 int sock = socket(AF_INET, SOCK_STREAM | SOCK_NONBLOCK, 0); // 监听套接字 int ret = bind(sock, (sockaddr*)&addr, sizeof(addr)); if (ret < 0) { // 处理错误 } ret = listen(sock, 10); if (ret < 0) { // 处理错误 } // 主线程循环 while (true) { struct pollfd pollfds[1]; pollfds[0].fd = sock; pollfds[0].events = POLLIN; int ret = poll(pollfds, 1, -1); if (ret < 0) { // 处理错误 } else if (pollfds[0].revents & POLLIN) { // 接受新连接 } }
HTTP/2
HTTP/2 is a binary protocol, compared with HTTP/1.1, it has more Good throughput. It allows for multiplexing, server push, and content compression to improve performance.
Practical case:
// 使用 OpenSSL 创建安全的 HTTP/2 服务器 SSL_CTX *ctx = SSL_CTX_new(TLS_server_method()); // 监听套接字 int sock = listen(ctx, sockfd, 10); // 主线程循环 while (true) { struct pollfd pollfds[1]; pollfds[0].fd = sock; pollfds[0].events = POLLIN; int ret = poll(pollfds, 1, -1); if (ret < 0) { // 处理错误 } else if (pollfds[0].revents & POLLIN) { // 接受新连接 SSL *ssl = SSL_new(ctx); SSL_set_fd(ssl, sockfd); } }
Conclusion
By implementing these strategies, you can significantly improve the throughput of your C++ server application. The specific implementation depends on the specific requirements and limitations of the application.
The above is the detailed content of Optimizing C++ server architecture to improve throughput. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
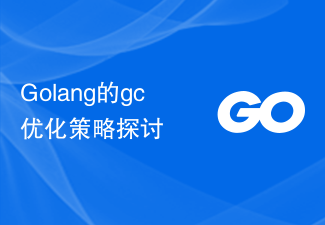
Golang's garbage collection (GC) has always been a hot topic among developers. As a fast programming language, Golang's built-in garbage collector can manage memory very well, but as the size of the program increases, some performance problems sometimes occur. This article will explore Golang’s GC optimization strategies and provide some specific code examples. Garbage collection in Golang Golang's garbage collector is based on concurrent mark-sweep (concurrentmark-s
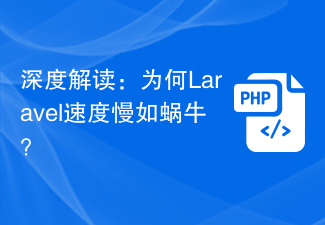
Laravel is a popular PHP development framework, but it is sometimes criticized for being as slow as a snail. What exactly causes Laravel's unsatisfactory speed? This article will provide an in-depth explanation of the reasons why Laravel is as slow as a snail from multiple aspects, and combine it with specific code examples to help readers gain a deeper understanding of this problem. 1. ORM query performance issues In Laravel, ORM (Object Relational Mapping) is a very powerful feature that allows

Decoding Laravel performance bottlenecks: Optimization techniques fully revealed! Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems. 1. Database query optimization using Eloquent delayed loading When using Eloquent to query the database, avoid
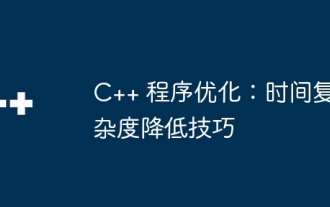
Time complexity measures the execution time of an algorithm relative to the size of the input. Tips for reducing the time complexity of C++ programs include: choosing appropriate containers (such as vector, list) to optimize data storage and management. Utilize efficient algorithms such as quick sort to reduce computation time. Eliminate multiple operations to reduce double counting. Use conditional branches to avoid unnecessary calculations. Optimize linear search by using faster algorithms such as binary search.
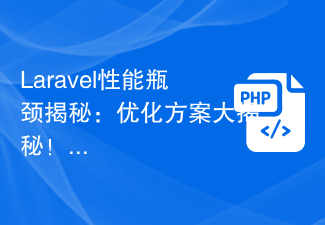
Laravel performance bottleneck revealed: optimization solution revealed! With the development of Internet technology, the performance optimization of websites and applications has become increasingly important. As a popular PHP framework, Laravel may face performance bottlenecks during the development process. This article will explore the performance problems that Laravel applications may encounter, and provide some optimization solutions and specific code examples so that developers can better solve these problems. 1. Database query optimization Database query is one of the common performance bottlenecks in Web applications. exist

Vivox100s parameter configuration revealed: How to optimize processor performance? In today's era of rapid technological development, smartphones have become an indispensable part of our daily lives. As an important part of a smartphone, the performance optimization of the processor is directly related to the user experience of the mobile phone. As a high-profile smartphone, Vivox100s's parameter configuration has attracted much attention, especially the optimization of processor performance has attracted much attention from users. As the "brain" of the mobile phone, the processor directly affects the running speed of the mobile phone.
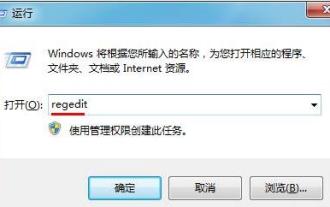
1. Press the key combination (win key + R) on the desktop to open the run window, then enter [regedit] and press Enter to confirm. 2. After opening the Registry Editor, we click to expand [HKEY_CURRENT_USERSoftwareMicrosoftWindowsCurrentVersionExplorer], and then see if there is a Serialize item in the directory. If not, we can right-click Explorer, create a new item, and name it Serialize. 3. Then click Serialize, then right-click the blank space in the right pane, create a new DWORD (32) bit value, and name it Star
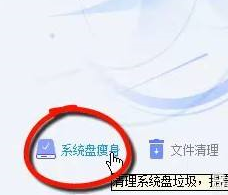
Are you also using Tencent Computer Manager software? So do you know how to optimize the Tencent Computer Manager system? The following is the method that the editor brings to you to optimize the Tencent Computer Manager system. Interested users can take a look below. . Open Tencent Computer Manager and click Junk Cleanup. After selecting System Disk Slimming to pop up the System Disk Slimming dialog box, we click to start scanning. After scanning the results, we click Release immediately. Return to the junk cleaning interface again and click File Cleanup. Clean Junk Tab - Recommended Choice - Start Scan - Clean Now. The same goes for clearing traces. It is recommended to select - Start Scan - Clean Now. Finally, system acceleration-select all-rescan
