How to choose golang framework
When choosing the appropriate Web framework in Go, you can choose according to your needs: Gin: lightweight, high-performance RESTful Web framework; Echo: scalable, robust RESTful Web framework; Gorilla: modular REST API construction Package; Beego: full-stack web framework, providing RESTful API, MVC and ORM; Hugo: static website generator, built based on Go, fast and flexible.
Go Framework Selection Guide
Choosing the right framework in Go can be a daunting task as there are many available Options to choose from. This article aims to help developers make an informed decision by providing a comprehensive comparison and a practical example.
The most popular framework in Go
- Gin: A REST-oriented web framework known for its simplicity, performance and functionality Rich and famous.
- Echo: Another RESTful web framework focused on scalability and robustness.
- Gorilla: A set of modular packages for building RESTful APIs, validation, routing and session handling.
- Beego: A full-stack web framework that provides RESTful API, MVC architecture and ORM integration.
- Hugo: A static website generator built on Go and known for its speed and flexibility.
Frame comparison
Features | Gin | Echo | Gorilla | Beego | Hugo |
---|---|---|---|---|---|
Support nested routing | Support nested routing | Support nested routing | Support nested routing | None | |
Integrated validator | Integrated validator | None | Integrated validator | None | |
None | None | None | Integrated ORM | None | |
Full support | Full support | Partial support | Full support | None | |
Support | Support | Support | Support | Support | |
Highly scalable | Highly scalable | Medium scalability | Low scalability | None | |
Excellent | Good | Good | Good | Excellent |
Now let’s create a simple RESTful API to demonstrate the Gin framework .
package main import ( "github.com/gin-gonic/gin" ) // 定义一个用于存储 JSON 数据的结构体 type Person struct { Name string `json:"name"` Age int `json:"age"` Address string `json:"address"` } // 主函数 func main() { // 创建 Gin 路由器 router := gin.Default() // 定义一个路由来获取所有人员 router.GET("/persons", getAllPersons) // 定义一个路由来获取特定人员 router.GET("/persons/:id", getPersonByID) // 定义一个路由来创建新的人员 router.POST("/persons", createPerson) // 定义一个路由来更新人员 router.PUT("/persons/:id", updatePerson) // 定义一个路由来删除人员 router.DELETE("/persons/:id", deletePerson) // 启动服务器 router.Run(":8080") } // 实现控制器函数 // getAllPersons 获取所有人员 func getAllPersons(c *gin.Context) { // 从数据库获取所有人员 persons := []Person{} // 将人员数据返回给客户端 c.JSON(200, persons) } // getPersonByID 通过 ID 获取人员 func getPersonByID(c *gin.Context) { // 从 URL 参数获取 ID id := c.Param("id") // 从数据库获取与 ID 匹配的人员 person := Person{} // 如果人员不存在,返回 404 错误 if person.ID == 0 { c.JSON(404, gin.H{"error": "Person not found"}) return } // 将人员数据返回给客户端 c.JSON(200, person) } // createPerson 创建新的人员 func createPerson(c *gin.Context) { // 从请求正文中解析 Person 数据 var person Person if err := c.BindJSON(&person); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } // 将新的人员保存到数据库 if err := person.Save(); err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } // 返回创建的 Person 数据 c.JSON(201, person) } // updatePerson 更新人员 func updatePerson(c *gin.Context) { // 从 URL 参数获取 ID id := c.Param("id") // 从数据库获取与 ID 匹配的人员 person := Person{} // 如果人员不存在,返回 404 错误 if person.ID == 0 { c.JSON(404, gin.H{"error": "Person not found"}) return } // 从请求正文中解析更新后的 Person 数据 var updatedPerson Person if err := c.BindJSON(&updatedPerson); err != nil { c.JSON(400, gin.H{"error": err.Error()}) return } // 更新人员数据 if err := person.Update(updatedPerson); err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } // 返回更新后的 Person 数据 c.JSON(200, person) } // deletePerson 删除人员 func deletePerson(c *gin.Context) { // 从 URL 参数获取 ID id := c.Param("id") // 从数据库获取与 ID 匹配的人员 person := Person{} // 如果人员不存在,返回 404 错误 if person.ID == 0 { c.JSON(404, gin.H{"error": "Person not found"}) return } // 删除人员 if err := person.Delete(); err != nil { c.JSON(500, gin.H{"error": err.Error()}) return } // 返回成功消息 c.JSON(200, gin.H{"message": "Person deleted successfully"}) }
The above is the detailed content of How to choose golang framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


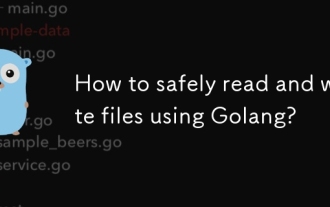
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
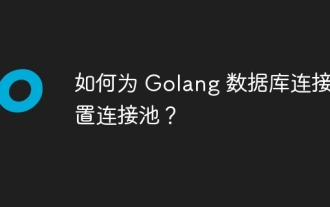
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
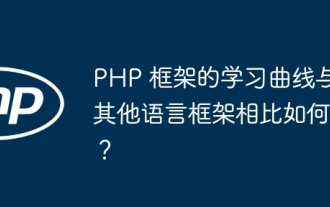
The learning curve of a PHP framework depends on language proficiency, framework complexity, documentation quality, and community support. The learning curve of PHP frameworks is higher when compared to Python frameworks and lower when compared to Ruby frameworks. Compared to Java frameworks, PHP frameworks have a moderate learning curve but a shorter time to get started.

The lightweight PHP framework improves application performance through small size and low resource consumption. Its features include: small size, fast startup, low memory usage, improved response speed and throughput, and reduced resource consumption. Practical case: SlimFramework creates REST API, only 500KB, high responsiveness and high throughput
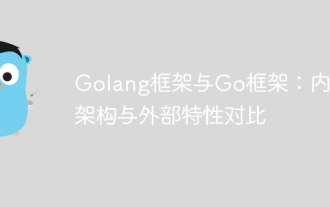
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
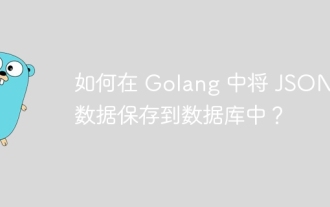
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
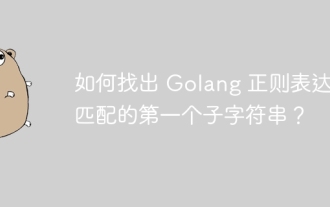
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
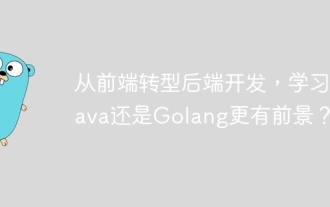
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
