How to implement message queue in Goroutine?
How to implement message queue in Goroutine? Use the make function to create an unbuffered channel. Use the <- operator to send messages. Use the -> operator to receive messages.
#How to implement message queue in Goroutine?
Introduction
Goroutines in Go are lightweight concurrency primitives that can be used to create parallel execution code. A message queue is a communication mechanism that allows Goroutines to send and receive messages asynchronously. This tutorial will introduce how to use channels to implement message queues in Go, and provide a practical case.
Implementing message queue
Channel in Go is a two-way communication pipe that can be used to transfer values between Goroutines. To create a channel, you can use the make
function. For example:
ch := make(chan int)
This code creates an unbuffered channel, which means it can only hold one value at a time.
Send a message
To send a message, use the channel's <-
operator. For example:
ch <- 42
This code sends the value 42
to the channel.
Receive messages
To receive messages, use the channel's ->
operator. For example:
msg := <-ch
This code will receive a value from the channel and store it in the msg
variable.
Practical Case
Let us create a simple producer-consumer application, in which the producer Goroutine will send messages, and the consumer Goroutine will receive and process them these messages.
Producer code
package main import "time" func main() { ch := make(chan int) go func() { for i := 0; i < 10; i++ { ch <- i time.Sleep(time.Second) } close(ch) }() }
Consumer code
package main import "time" func main() { ch := make(chan int) go func() { for { msg, ok := <-ch if !ok { break } time.Sleep(time.Second) println(msg) } }() }
In this example, the producer sends one message every second into the channel, and the consumer will receive and process these messages from the channel at the same frequency.
The above is the detailed content of How to implement message queue in Goroutine?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
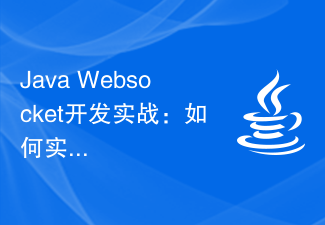
Java Websocket development practice: How to implement the message queue function Introduction: With the rapid development of the Internet, real-time communication is becoming more and more important. In many web applications, real-time updates and notification capabilities are required through real-time messaging. JavaWebsocket is a technology that enables real-time communication in web applications. This article will introduce how to use JavaWebsocket to implement the message queue function and provide specific code examples. Basic concepts of message queue
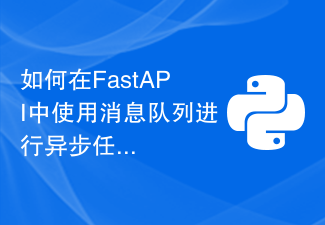
How to use message queues for asynchronous task processing in FastAPI Introduction: In web applications, it is often necessary to process time-consuming tasks, such as sending emails, generating reports, etc. If these tasks are placed in a synchronous request-response process, users will have to wait for a long time, reducing user experience and server response speed. In order to solve this problem, we can use message queue for asynchronous task processing. This article will introduce how to use message queues to process asynchronous tasks in the FastAPI framework.
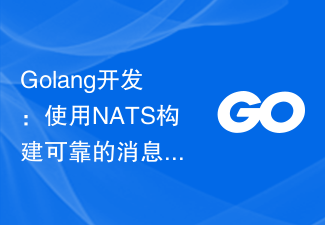
Golang development: Using NATS to build a reliable message queue, specific code examples are required Introduction: In modern distributed systems, the message queue is an important component used to handle asynchronous communication, decouple system components and achieve reliable message delivery. This article will introduce how to use the Golang programming language and NATS (the full name is "High Performance Reliable Message System") to build an efficient and reliable message queue, and provide specific code examples. What is NATS? NATS is a lightweight, open source messaging system.
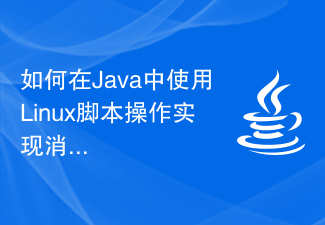
How to use Linux script operations to implement message queues in Java requires specific code examples. Message queues are a common communication mechanism used to transfer data between different processes. In Java, we can implement message queues using Linux script operations so that we can easily send messages to or receive messages from the queue. In this article, we will detail how to implement message queues using Java and Linux scripts, and provide specific code examples. To get started with Java and Lin
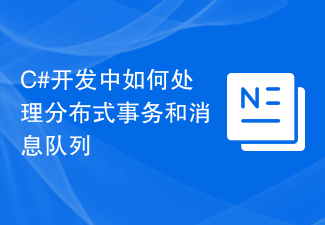
How to handle distributed transactions and message queues in C# development Introduction: In today's distributed systems, transactions and message queues are very important components. Distributed transactions and message queues play a crucial role in handling data consistency and system decoupling. This article will introduce how to handle distributed transactions and message queues in C# development, and give specific code examples. 1. Distributed transactions Distributed transactions refer to transactions that span multiple databases or services. In distributed systems, how to ensure data consistency has become a major challenge. Here are two types of
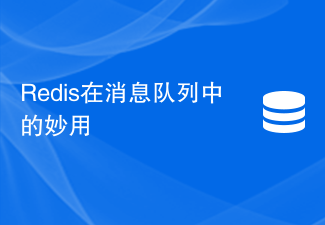
The wonderful use of Redis in message queues Message queues are a common decoupled architecture used to deliver asynchronous messages between applications. By sending a message to a queue, the sender can continue performing other tasks without waiting for a response from the receiver. And the receiver can get the message from the queue and process it at the appropriate time. Redis is a commonly used open source in-memory database with high performance and persistent storage capabilities. In message queues, Redis's multiple data structures and excellent performance make it an ideal choice
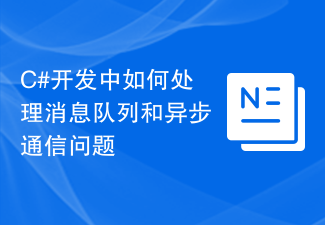
How to handle message queues and asynchronous communication issues in C# development Introduction: In modern software development, as the size and complexity of applications continue to increase, it is very important to effectively handle message queues and implement asynchronous communication. Some common application scenarios include message passing between distributed systems, background task queue processing, event-driven programming, etc. This article will explore how to deal with message queues and asynchronous communication issues in C# development, and provide specific code examples. 1. Message queue Message queue is an asynchronous communication mechanism that allows messages to be sent by
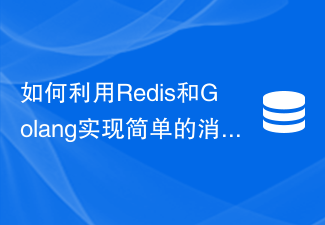
How to use Redis and Golang to implement a simple message queue Introduction Message queues are widely used in various application scenarios, such as decoupling system components, peak shaving and valley filling, asynchronous communication, etc. This article will introduce how to use Redis and Golang to implement a simple message queue, helping readers understand the basic principles and implementation methods of message queues. Introduction to Redis Redis is an open source in-memory database written in C language, which provides key-value pair storage and processing functions for other commonly used data structures. Redis is known for its high performance,
