How to use proxy for HTTP requests in Golang?
Jun 01, 2024 pm 02:17 PMUsing a proxy for HTTP requests in Go can bypass firewalls, hide IP addresses, and improve performance. The following steps implement proxy requests: 1. Import the necessary packages. 2. Create a proxy URL. 3. Create a proxy HTTP transport. 4. Create an HTTP client. 5. Use the client to make the request. Practical case: Use code to bypass the firewall to access restricted URLs, make requests through proxy transmission, and print the response status.
How to use a proxy for HTTP requests in Golang
Using a proxy for HTTP requests is useful in many situations, such as:
- Bypass firewalls or geo-restrictions
- Hide your real IP address
- Improve request performance
Steps to use a proxy for HTTP requests in Go As follows:
- Import the necessary packages:
package main import ( "fmt" "net/http" "net/http/httputil" "net/url" )
- Create a proxy URL:
proxyURL, err := url.Parse("http://127.0.0.1:8080") if err != nil { fmt.Println(err) return }
- Create a proxy HTTP transfer:
transport := &http.Transport{ Proxy: http.ProxyURL(proxyURL), }
- Create an HTTP client:
client := &http.Client{ Transport: transport, }
- Use HTTP client to make a request:
resp, err := client.Get("https://www.example.com") if err != nil { fmt.Println(err) return }
Practical Case
Consider a scenario where you need to bypass the firewall to access restricted URLs. This can be done using the following code:
import ( "fmt" "net/http" "net/http/httputil" "net/url" ) func main() { proxyURL, err := url.Parse("http://127.0.0.1:8080") if err != nil { fmt.Println(err) return } transport := &http.Transport{ Proxy: http.ProxyURL(proxyURL), } client := &http.Client{ Transport: transport, } resp, err := client.Get("https://firewall-protected-url.com") if err != nil { fmt.Println(err) return } fmt.Println(resp.Status) }
The above is the detailed content of How to use proxy for HTTP requests in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
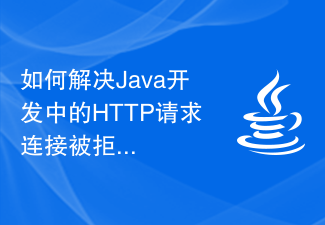
How to solve the problem of HTTP request connection refused in Java development
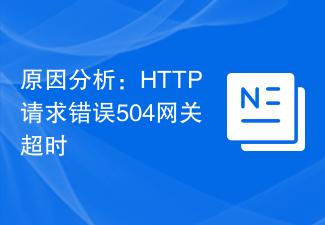
Cause analysis: HTTP request error 504 gateway timeout
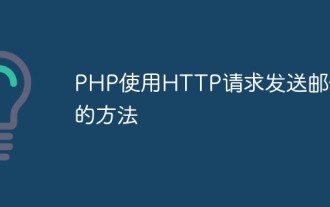
How to send email using PHP HTTP request
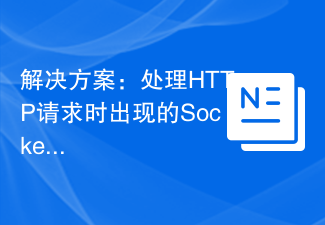
Solution: Socket Error when handling HTTP requests
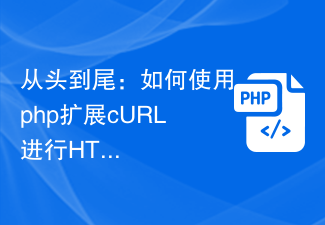
From start to finish: How to use php extension cURL to make HTTP requests
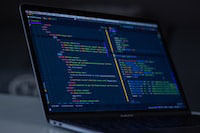
Python HTTP Request Optimization Guide: Improve the Performance of Your Web Applications
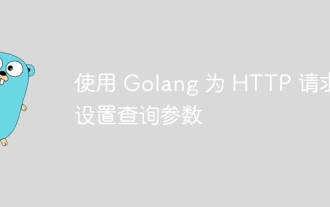
Set query parameters for HTTP requests using Golang
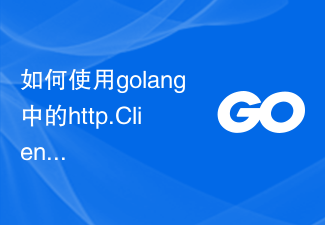
How to use http.Client in golang for advanced operations of HTTP requests
