How to extract data using regular expressions in Golang?
Regular expressions can be used to extract data in Go. Use the regexp package to process regular expressions: Compile regular expressions: regexp.Compile("match pattern") Use the Find or FindAll function to extract data: a. r.FindString(str) match The first match b. r.FindAllString(str, -1) matches all matches
How to use regular expressions to extract data in Golang
Regex is a powerful tool in Golang that can be used to match, search and replace patterns in strings. This article will introduce how to use regular expressions to extract data in Golang and provide a practical case.
Regular expression basics
Regular expressions consist of a set of special characters that are used to match patterns of character sequences. Some common special characters include:
-
.
: matches any single character -
*
: matches the previous character 0 or more times Times -
+
: Match the previous character 1 or more times -
?
: Match the previous character 0 or 1 times -
[]
: Matches any characters within square brackets -
^
: Matches the beginning of the string -
$
: Matches the end of a string
Regular Expressions in Go Language
Golang uses the regexp
package to handle regular expressions. regexp
Package provides various functions for matching, searching and replacing strings.
Here's how to use the regexp
package in Go:
import "regexp" // 编译正则表达式 r, err := regexp.Compile("匹配模式") if err != nil { // 处理错误 }
Extracting data
To extract data from a string , you can use the Find
or FindAll
function. These functions return a slice containing matches.
For example, to extract all numbers from a string:
str := "123abc456def" r := regexp.MustCompile("[0-9]+") // 必须使用 MustCompile 来避免错误处理 matches := r.FindAllString(str, -1) fmt.Println(matches) // 输出:["123", "456"]
Practical case: Parsing JSON
The following is an example of parsing JSON using regular expressions Practical case of string:
import ( "fmt" "regexp" ) const jsonStr = `{ "name": "Alice", "age": 25, "city": "New York" }` // 编译正则表达式 r := regexp.MustCompile(`"(\w+)": (".*?")`) // 匹配 JSON 字符串中的字段 matches := r.FindAllStringSubmatch(jsonStr, -1) // 解析字段 for _, match := range matches { field := match[1] value := match[2] fmt.Printf("Field: %s, Value: %s\n", field, value) }
Output:
Field: name, Value: "Alice" Field: age, Value: "25" Field: city, Value: "New York"
The above is the detailed content of How to extract data using regular expressions in Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
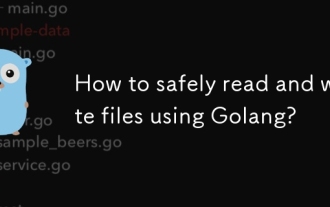
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
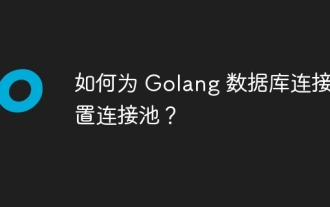
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
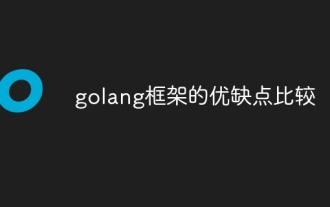
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
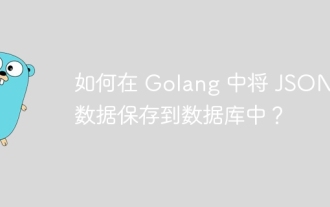
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
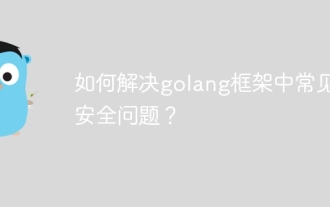
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
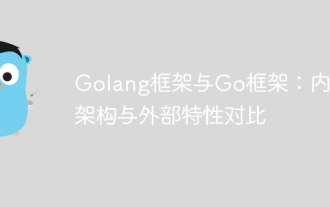
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
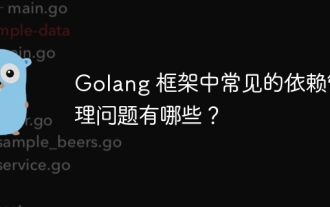
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
