How to use Golang's recover() function to handle panics?
By using the recover() function, you can capture panics in the current function context, prevent program crashes and handle errors gracefully: recover() returns nil when no panic occurs, and when an uncaught panic occurs or recovers from the function where the panic occurred Returns the panic value. Add defer callbacks around function calls to catch panics and perform custom handling, such as logging error information. recover() only captures panics in the current function context, does not cancel panics, and only works on unhandled errors.
How to use Golang’s recover() function to deal with panic
Introduction
Panic is a special error handling mechanism in the Go language. When the program encounters an error that cannot be handled, it will cause the program to crash. recover()
The function can catch and handle panics, allowing the program to recover from errors gracefully.
recover()
Function
recover()
function is a built-in function that can be captured from the current function context Recent panic. It is returned if:
- A panic occurred and was not caught.
- The program recovers from the panicked function.
If no panic occurs, recover()
will return a nil
value.
Practical case
Consider a function that reads a file. The function may have the following errors:
func readFile(filename string) ([]byte, error) { data, err := os.ReadFile(filename) if err != nil { return nil, err } return data, nil }
To use recover( )
function to catch this error, you can add a defer
statement around the calling function:
func main() { defer func() { if err := recover(); err != nil { log.Printf("捕获到恐慌: %v", err) } }() _, err := readFile("non-existent-file.txt") if err != nil { log.Printf("读取文件出错:%v", err) } }
When the program tries to read a file that does not exist, a panic will occur and then defer
The recover()
function in the callback captures the panic. This allows the program to log errors and exit gracefully.
Note
-
recover()
can only capture panics from the current function context, so if the panic occurs in a nested function , it cannot be captured. -
recover()
function does not cancel the panic, which means that the program will continue to crash even if the panic is caught. -
recover()
The function should only be used to handle unhandled errors and should not replace normal error handling mechanisms.
The above is the detailed content of How to use Golang's recover() function to handle panics?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
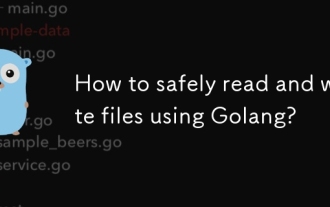
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
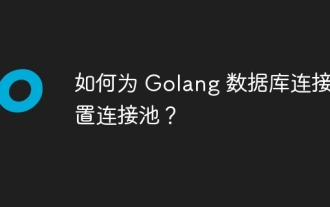
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
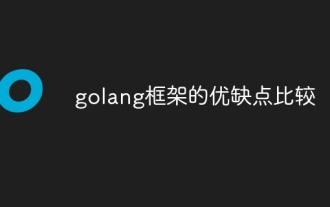
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
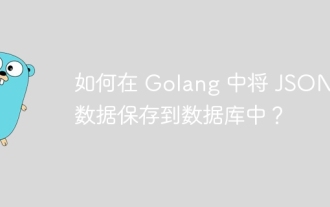
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
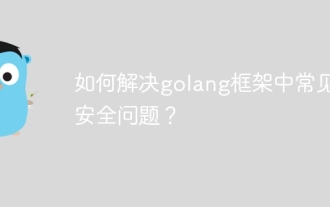
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
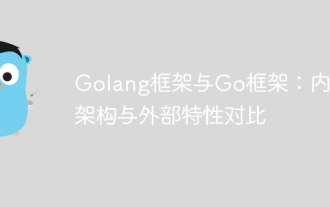
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
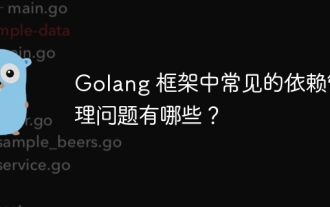
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
