


What are the best practices to improve efficiency in development based on Java framework?
This guide provides Java framework best practices to improve development efficiency: Choose a framework (Spring Boot, Play Framework, and Vert.x) that meets your functionality, performance, and scalability needs. Simplify testing and modularization with dependency injection (@Autowired). Use ORM (Hibernate, JPA) to connect to database and map objects. Adopt RESTful architecture to improve maintainability and reusability. Use caching (Ehcache, Redis) to improve performance. Automate build and deployment processes (Maven, Gradle, Jenkins, Travis CI). Practical case: Spring Boot API development (@RestController, @RequestMapping, @Autowired, @GetMapping, @PostMapping).
Based on the best practices of Java framework to improve development efficiency
Introduction
Using Java frameworks can significantly improve the efficiency of Web application development. This article will explore some best practices to help you maximize the power of these frameworks.
Choose the right framework
Choosing the right framework is crucial. Consider the following:
- Functional Requirements
- Performance Goals
- Scalability Requirements
- Development Team Skills
Popular Java frameworks include Spring Boot, Play Framework and Vert.x.
Leverage Dependency Injection
Dependency Injection (DI) is a design pattern that allows you to decouple objects and their dependencies. This simplifies unit testing and modular development. In Spring Boot, use the @Autowired
annotation to implement DI.
Using ORM for Data Management
The Object Relational Mapping (ORM) framework simplifies interacting with databases. They map database tables to Java objects, allowing data to be queried and modified without writing raw SQL. Popular ORMs include Hibernate and JPA.
Adopt RESTful architecture
RESTful architecture is a lightweight, scalable web service design style. It is based on HTTP verbs and Resource Representation State Transfer (REST). Using RESTful APIs improves code maintainability and reusability.
Utilizing cache
Cache can significantly improve performance, especially for frequently accessed data. Spring Boot provides several built-in caching solutions such as Ehcache and Redis. Use them to cache query results and expensive calculations.
Automated Build and Deployment
Automating the build and deployment process saves time and reduces errors. Use a build tool like Maven or Gradle, combined with a continuous integration (CI) system like Jenkins or Travis CI.
Practical case
Spring Boot API development
Build RESTful API using Spring Boot:
@RestController @RequestMapping("/api/customers") public class CustomerController { @Autowired private CustomerService customerService; @GetMapping public List<Customer> getAllCustomers() { return customerService.findAll(); } @PostMapping public Customer createCustomer(@RequestBody Customer customer) { return customerService.save(customer); } }
After completing these steps, you will have a basic customer API that can be used to create, read, update, and delete customer records.
Conclusion
By adopting the best practices outlined in this article, you can leverage the power of Java frameworks and significantly improve your web application development productivity. From choosing the right framework to automating builds and deployments, these practices will help you create maintainable, scalable, and efficient applications.
The above is the detailed content of What are the best practices to improve efficiency in development based on Java framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


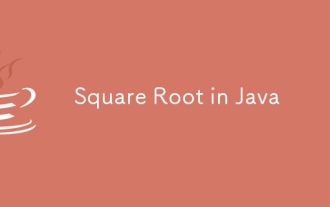
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
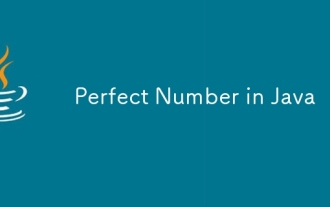
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
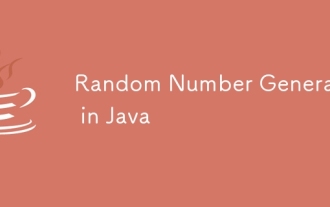
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
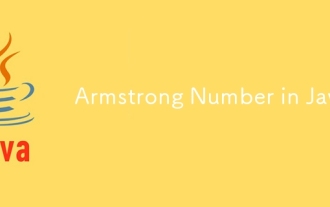
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
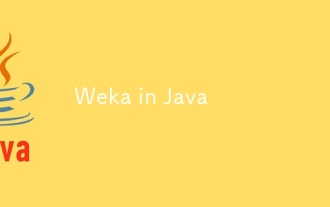
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
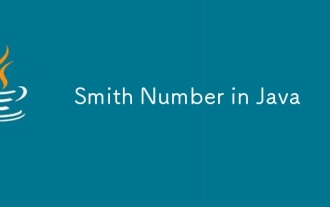
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
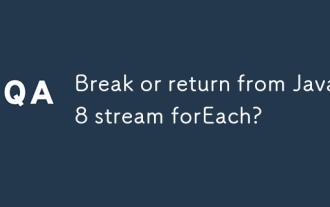
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
