How to optimize Golang cache performance?
Tips to improve Golang cache performance include: choosing an appropriate cache library, such as sync.Map, github.com/patrickmn/go-cache and github.com/go-cache/cache. Optimize the data structure, use map to store data, and consider using jump tables to implement hierarchical cache storage. Take advantage of concurrency control, using read-write locks, sync.Map, or channels to manage concurrency.
Tips to improve Golang cache performance
Optimizing cache performance is crucial, it can greatly improve the response time of the application and throughput. This article will explore practical techniques for optimizing cache performance in Golang and explain them through practical cases.
1. Choose an appropriate cache library
- sync.Map: suitable for small-scale concurrent caching scenarios.
- github.com/patrickmn/go-cache: Provides functions such as expiration time, synchronous and asynchronous operations.
- github.com/go-cache/cache: Supports TTL, multiple storage backends and concurrency control.
2. Optimize the data structure
- Use mapping to store cached data and quickly find values based on keys.
- Consider using skiplist to implement hierarchical cache storage and achieve fast range search and update.
3. Utilize concurrency control
- The go-cache library provides read-write locks to allow concurrent reading and writing.
- sync.Map provides safe concurrent operations and is suitable for lock-free environments.
- Use channels to manage concurrent writes to avoid data competition.
Practical case: using go-cache library
import ( "context" "fmt" "time" "github.com/patrickmn/go-cache" ) func main() { // 创建一个缓存,过期时间为 5 分钟 c := cache.New(5 * time.Minute, 10 * time.Minute) // 设置缓存值 c.Set("key", "value", cache.DefaultExpiration) // 获取缓存值 value, ok := c.Get("key") if ok { fmt.Println(value) } // 定期清理过期的缓存值 go func() { for { c.DeleteExpired() time.Sleep(5 * time.Second) } }() // 等待缓存处理器退出后再退出程序 ctx := context.Background() <-ctx.Done() }
Other optimization techniques
- Use LRU The cache policy evicts the least used items.
- Consider using a distributed cache such as Redis or Memcached to meet large-scale caching needs.
- Use cache hit rate monitoring to evaluate cache efficiency.
The above is the detailed content of How to optimize Golang cache performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


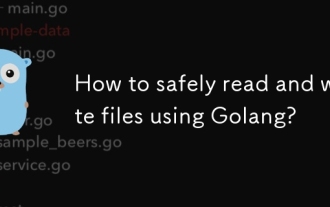
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
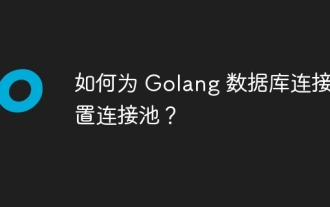
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
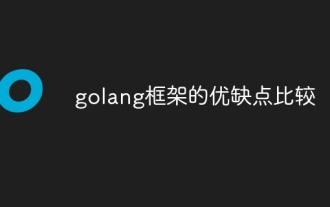
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
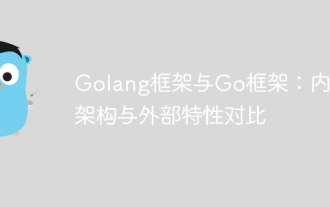
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
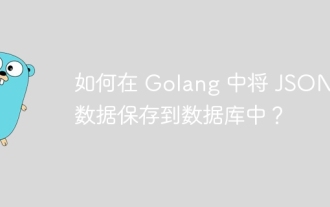
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
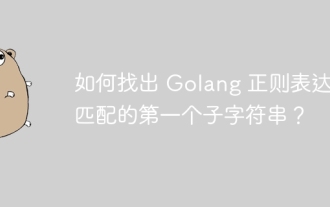
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
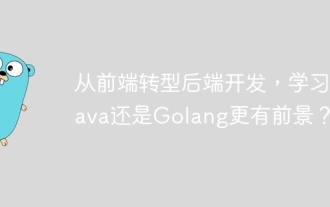
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
