


PHP data structure: application of priority queue, controlling the acquisition of ordered elements
Priority queues allow elements to be stored and accessed by priority, setting priorities based on comparable criteria such as value, timestamp, or custom logic. Implementation methods in PHP include the SplPriorityQueue class and the Min/Max heap. The practical case demonstrates how to use the SplPriorityQueue class to create a priority queue and obtain elements by priority.
PHP data structure: application of priority queue, controlling the acquisition of ordered elements
Priority queue is a data structure, It allows you to store elements and access them by priority. Priority can be based on any comparable criteria, such as the element's value, timestamp, or other custom logic.
Implementation of priority queue
There are many ways to implement priority queue in PHP:
- ##SplPriorityQueue class: The SplPriorityQueue class implemented in the standard PHP library provides an out-of-the-box priority queue implementation.
- Min/Max Heap: You can use the Min/Max heap to implement a priority queue. The lowest priority element in a min-heap is at the root, while the highest-priority element in a max-heap is at the root.
Practical case
The following is a practical case using the SplPriorityQueue class to implement a priority queue:<?php // 创建一个优先队列 $queue = new SplPriorityQueue(); // 将元素添加到队列,并指定它们的优先级 $queue->insert('Item 1', 1); $queue->insert('Item 2', 3); $queue->insert('Item 3', 2); // 循环队列并按优先级获取元素 foreach ($queue as $item) { echo $item . PHP_EOL; } ?>
Item 2 Item 3 Item 1
Conclusion
Priority queue is a useful tool in data structures that allows you to store elements and access them according to priority. This tutorial provides methods for implementing priority queues in PHP and a practical case to help you understand its application.The above is the detailed content of PHP data structure: application of priority queue, controlling the acquisition of ordered elements. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


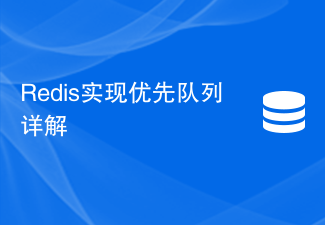
Detailed explanation of Redis implementation of priority queue Priority queue is a common data structure that can sort elements according to certain rules and maintain this order during queue operations, so that elements taken out of the queue always follow the preset priority conduct. As an in-memory database, Redis also has advantages in implementing priority queues because of its fast and efficient data access capabilities. This article will introduce in detail the method and application of Redis to implement priority queue. 1. Basic principles of Redis implementation Basic principles of Redis implementation of priority queue
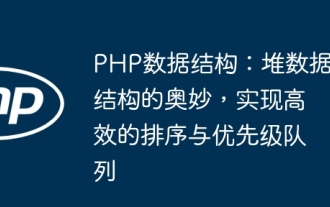
The heap data structure in PHP is a tree structure that satisfies the complete binary tree and heap properties (the parent node value is greater/less than the child node value), and is implemented using an array. The heap supports two operations: sorting (extracting the largest element from small to large) and priority queue (extracting the largest element according to priority). The properties of the heap are maintained through the heapifyUp and heapifyDown methods respectively.
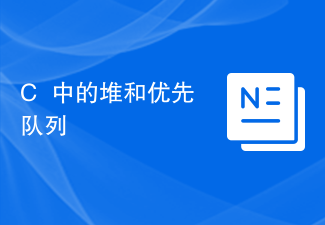
Heap and priority queue are commonly used data structures in C++, and they both have important application value. This article will introduce and analyze the heap and priority queue respectively to help readers better understand and use them. 1. Heap is a special tree data structure that can be used to implement priority queues. In the heap, each node satisfies the following properties: its value is not less than (or not greater than) the value of its parent node. Its left and right subtrees are also a heap. We call a heap that is no smaller than its parent node a "min-heap" and a heap that is no larger than its parent node a "max-heap"

What are the usage scenarios of heap and priority queue in Python? The heap is a special binary tree structure that is often used to efficiently maintain a dynamic collection. The heapq module in Python provides a heap implementation and can easily perform heap operations. A priority queue is also a special data structure. Unlike an ordinary queue, each element of it has a priority associated with it. The highest priority element is taken out first. The heapq module in Python can also implement the priority queue function. Below we introduce some
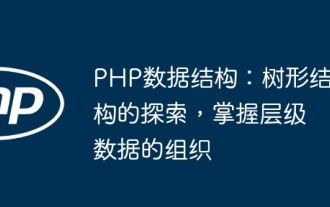
The tree structure is a non-linear structure that organizes data hierarchically, and can be represented and traversed recursively or iteratively in PHP. Representation methods include recursion (using class) and iteration (using array); traversal methods include recursive traversal and iterative traversal (using stack). In the actual case, the file system directory tree is efficiently organized using a tree structure to facilitate browsing and obtaining information.
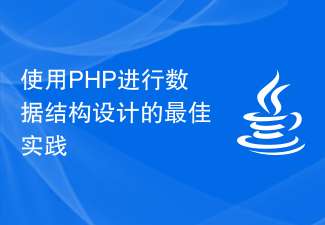
As one of the most widely used programming languages, PHP also has its own advantages and best practices when designing data structures. When designing data structures, PHP developers need to consider some key factors, including data type, performance, code readability, and reusability. The following will introduce the best practices for data structure design using PHP. Selection of data types Data types are one of the key factors in data structure design because they affect program performance, memory usage, and code readability. In PHP, there is
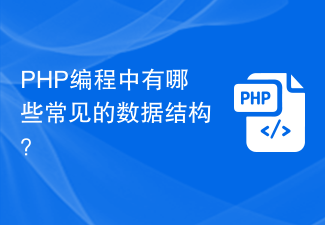
In the PHP programming language, data structure is a very important concept. It is a method used to organize and store data in program design. PHP has various data structure capabilities, such as arrays, linked lists, stacks, etc., making it highly valuable in actual programming. In this article, we will introduce several common data structures in PHP programming so that programmers can master them proficiently and apply them flexibly. Array Array is a basic data type in PHP programming. It is an ordered collection composed of the same type of data and can be stored under a single variable name.
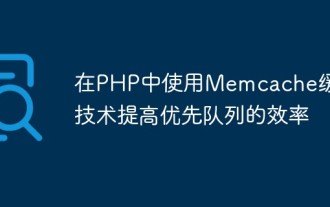
With the continuous development of society, people's requirements for computer technology are becoming higher and higher. In computers, queues are a very important data structure that can help us solve many problems efficiently. However, in actual application processes, the efficiency of the queue is often limited by some factors, such as network delay, database query speed, etc. So, today we will introduce a way to solve this problem: using Memcache caching technology in PHP to improve the efficiency of the priority queue. 1. What is a priority queue?
