Best practices and common pitfalls of Java frameworks?
Java Framework Best Practices and Common Pitfalls
Using Java frameworks can greatly simplify web development, but following best practices is crucial. to avoid common pitfalls.
Best Practices:
- Choose the right framework: Choose a framework carefully based on your project needs, considering its functionality, performance, and community support.
- Use Dependency Injection: This helps decouple components and facilitate testing.
- Follow the MVC design pattern: Clearly separate views, models, and controllers to improve maintainability.
- Use RESTful API: Provide a standardized and reusable interface for interacting with client applications.
- Implement security measures: Protect applications from attacks such as XSS, CSRF, and SQL injection.
- Conduct unit testing: Verify the correctness of the code and improve the reliability of the application.
Common pitfalls:
- Overuse of framework features: The framework only provides a structure, you should only use it when necessary Use its functionality.
- Abuse of Dependency Injection: Excessive dependency injection can increase complexity and reduce performance.
- Violation of MVC principles: Mixing business logic into views or controllers destroys the clarity of the design.
- Neglecting Security: Failure to implement appropriate security measures can lead to application vulnerabilities.
- Lack of testing: Skipping unit tests can lead to increased bugs and maintenance issues.
Practical case:
Create a simple web application using Spring Boot:
@SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } } @RestController @RequestMapping("/user") public class UserController { @GetMapping public String greet() { return "Hello, world!"; } }
In this example, we use Spring Boot framework, dependency injection injects @Component
annotated beans into UserController
, MVC design pattern clearly separates controllers and views, RESTful API through @RestController
and @RequestMapping
implementations.
The above is the detailed content of Best practices and common pitfalls of Java frameworks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


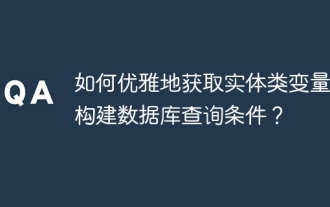
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
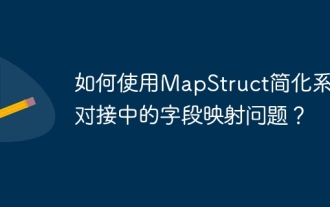
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
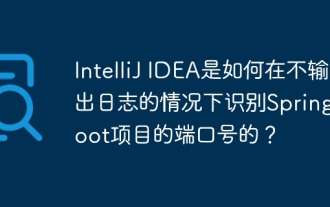
Start Spring using IntelliJIDEAUltimate version...
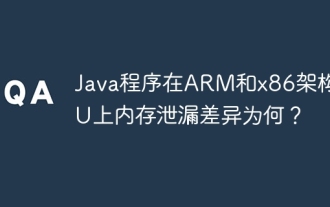
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
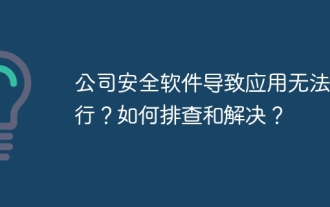
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
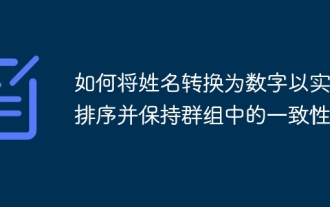
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
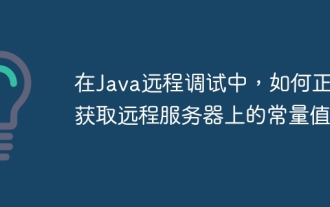
Questions and Answers about constant acquisition in Java Remote Debugging When using Java for remote debugging, many developers may encounter some difficult phenomena. It...
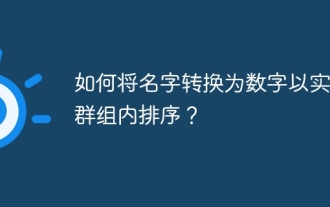
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
