How to handle database connections and operations using C++?
在C++中使用Data Access Objects (DAO) 库连接和操作数据库,包括建立数据库连接、执行SQL查询、插入新记录和更新现有记录。具体步骤为:1. 包含必要的库语句;2. 打开数据库文件;3. 创建Recordset对象执行SQL查询或操作数据;4. 遍历结果或按照具体需求更新记录。
如何在C++中进行数据库连接和操作
C++能够使用不同的库来连接和操作数据库,最常用的库是[Data Access Objects (DAO) Library](https://docs.microsoft.com/en-us/cpp/win32/data-access-objects-library?view=msvc-170)。
建立数据库连接
#include <dao.h> int main() { try { // 打开数据库文件 Database db("mydb.mdb"); // or Database db(L"c:\\mydb\\mydb.mdb", DB_SHARED_DENY); return 0; } catch (const DatabaseException& e) { std::cerr << "Error connecting to the database: " << e.what() << std::endl; return 1; } }
执行SQL查询
#include <dao.h> int main() { try { // 打开数据库文件 Database db("mydb.mdb"); // 创建一个Recordset对象来执行SQL查询 Recordset rs = db.OpenRecordset("SELECT * FROM Customers"); // 遍历并打印结果 while (!rs.IsEOF()) { std::cout << "Customer ID: " << rs("CustomerID").lVal << std::endl; std::cout << "Customer Name: " << rs("CustomerName").StringValue << std::endl; std::cout << "Customer Email: " << rs("CustomerEmail").StringValue << std::endl; rs.MoveNext(); } return 0; } catch (const DatabaseException& e) { std::cerr << "Error executing SQL query: " << e.what() << std::endl; return 1; } }
插入新记录
#include <dao.h> int main() { try { // 打开数据库文件 Database db("mydb.mdb"); // 创建一个Recordset对象来插入新记录 Recordset rs = db.OpenRecordset("Customers"); rs.AddNew(); rs("CustomerID") = 4; rs("CustomerName") = "New Customer"; rs("CustomerEmail") = "new-customer@email.com"; // 更新记录 rs.Update(); return 0; } catch (const DatabaseException& e) { std::cerr << "Error inserting new record: " << e.what() << std::endl; return 1; } }
更新现有记录
#include <dao.h> int main() { try { // 打开数据库文件 Database db("mydb.mdb"); // 创建一个Recordset对象来执行SQL查询 Recordset rs = db.OpenRecordset("SELECT * FROM Customers WHERE CustomerID = 4"); // 更新记录 if (!rs.IsEOF()) { rs.Edit(); rs("CustomerEmail") = "updated-new-customer@email.com"; rs.Update(); } return 0; } catch (const DatabaseException& e) { std::cerr << "Error updating existing record: " << e.what() << std::endl; return 1; } }
The above is the detailed content of How to handle database connections and operations using C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


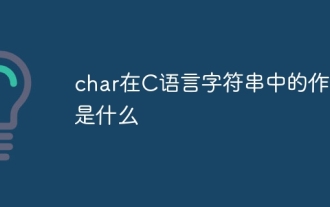
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
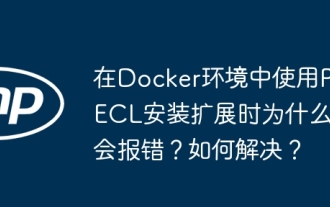
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
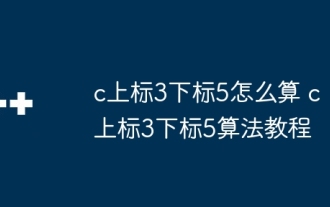
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
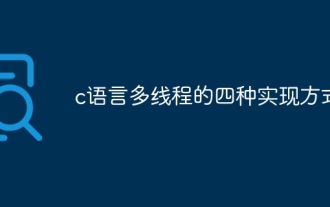
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
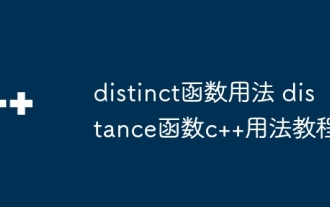
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
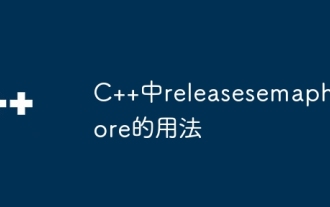
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
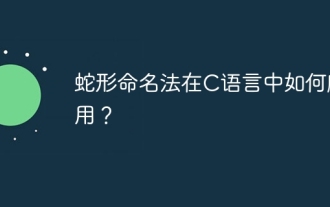
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
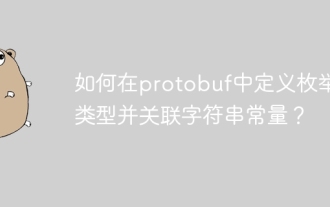
Issues of defining string constant enumeration in protobuf When using protobuf, you often encounter situations where you need to associate the enum type with string constants...
