


Deadlock prevention and detection mechanism in C++ multi-threaded programming
Multi-thread deadlock prevention mechanism includes: 1. Lock sequence; 2. Test and set up. Detection mechanisms include: 1. Timeout; 2. Deadlock detector. The article takes an example of a shared bank account and avoids deadlock through lock sequence. The transfer function first requests the lock of the transfer out account and then the transfer in account.
Deadlock prevention and detection mechanism in C++ multi-threaded programming
In a multi-threaded environment, deadlock is a common errors that may cause the program to stop responding. A deadlock occurs when multiple threads wait indefinitely for each other to release their locks, creating a waiting loop.
In order to avoid and detect deadlocks, C++ provides several mechanisms:
Prevention mechanism
- Lock order: Develop a strict request lock order for all shared mutable data to ensure that all threads always request locks in the same order.
-
Test and set: Use
std::atomic
provided by thestd::atomic
library to test and set variables to check whether the lock has been request and then set it up immediately.
Detection mechanism
- Timeout:Set a timeout for the lock request. If the lock is not obtained after the time, then Throw an exception or take other appropriate action.
- Deadlock Detector: Use third-party libraries such as Boost.Thread to monitor thread activity, detect deadlocks and take necessary actions.
Practical case:
Consider the following shared bank account example:
class BankAccount { private: std::mutex m_; int balance_; public: void deposit(int amount) { std::lock_guard<std::mutex> lock(m_); balance_ += amount; } bool withdraw(int amount) { std::lock_guard<std::mutex> lock(m_); if (balance_ >= amount) { balance_ -= amount; return true; } return false; } };
The way to avoid deadlock is to use lock order: request first deposit()
lock, and then request withdraw()
lock.
void transfer(BankAccount& from, BankAccount& to, int amount) { std::lock_guard<std::mutex> fromLock(from.m_); std::lock_guard<std::mutex> toLock(to.m_); if (from.withdraw(amount)) { to.deposit(amount); } }
Deadlock can be prevented by requesting locks in the order of transfers.
The above is the detailed content of Deadlock prevention and detection mechanism in C++ multi-threaded programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


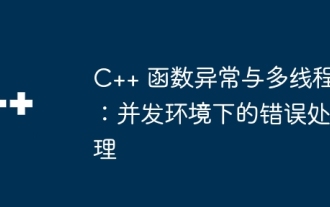
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
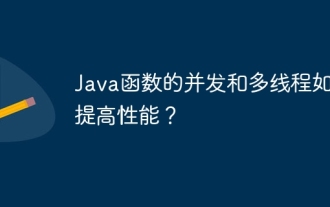
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
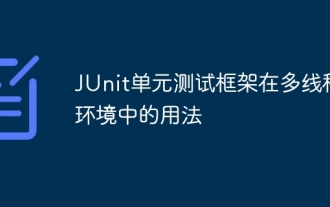
There are two common approaches when using JUnit in a multi-threaded environment: single-threaded testing and multi-threaded testing. Single-threaded tests run on the main thread to avoid concurrency issues, while multi-threaded tests run on worker threads and require a synchronized testing approach to ensure shared resources are not disturbed. Common use cases include testing multi-thread-safe methods, such as using ConcurrentHashMap to store key-value pairs, and concurrent threads to operate on the key-value pairs and verify their correctness, reflecting the application of JUnit in a multi-threaded environment.
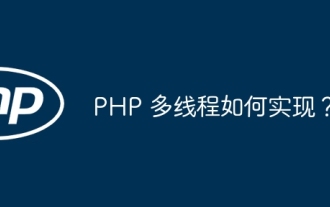
PHP multithreading refers to running multiple tasks simultaneously in one process, which is achieved by creating independently running threads. You can use the Pthreads extension in PHP to simulate multi-threading behavior. After installation, you can use the Thread class to create and start threads. For example, when processing a large amount of data, the data can be divided into multiple blocks and a corresponding number of threads can be created for simultaneous processing to improve efficiency.
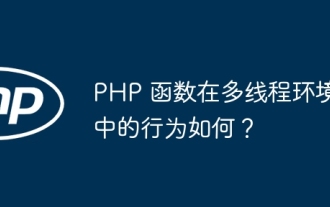
In a multi-threaded environment, the behavior of PHP functions depends on their type: Normal functions: thread-safe, can be executed concurrently. Functions that modify global variables: unsafe, need to use synchronization mechanism. File operation function: unsafe, need to use synchronization mechanism to coordinate access. Database operation function: Unsafe, database system mechanism needs to be used to prevent conflicts.
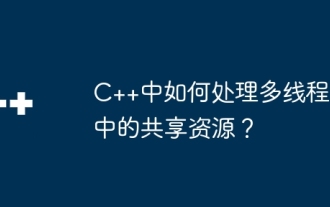
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
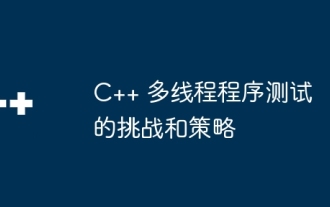
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks, and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools to find potential data races, such as valgrind. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
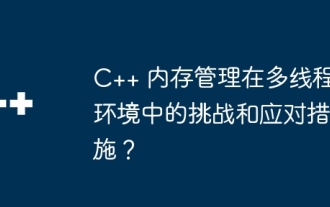
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
