How to read and write binary files using C++?
Methods to read and write binary files in C++: Write binary files: Use the std::ofstream class and set the output mode to std::ios::binary. Read binary files: Use the std::ifstream class and set the input mode to std::ios::binary.
How to read and write binary files in C++
A binary file is a special file type that stores non-text data, e.g. Images, Audio and Archives. There are two main operations when working with binary files in C++: reading and writing.
Writing to binary files
Use the std::ofstream
class to write to binary files. When opening the file, specify the output mode as binary mode (std::ios::binary
).
// 打开文件以进行二进制写入 std::ofstream outFile("binaryFile.bin", std::ios::binary); // 向文件写入二进制数据 outFile.write((char*) &data, sizeof(data)); // 关闭文件 outFile.close();
Reading binary files
Use the std::ifstream
class to read binary files. Likewise, specify binary mode when opening the file.
// 打开文件以进行二进制读取 std::ifstream inFile("binaryFile.bin", std::ios::binary); // 从文件读取二进制数据 inFile.read((char*) &data, sizeof(data)); // 关闭文件 inFile.close();
Practical case: reading and displaying images
The following code snippet demonstrates how to read an image file in C++ and display it in the console:
#include <iostream> #include <fstream> #include <vector> int main() { // 二进制图像文件 std::string fileName = "image.bmp"; // 打开图像文件以进行二进制读取 std::ifstream inFile(fileName, std::ios::binary); // 检查文件是否打开 if (!inFile.is_open()) { std::cerr << "无法打开文件 " << fileName << std::endl; return 1; } // 获取文件大小 inFile.seekg(0, std::ios::end); size_t fileSize = inFile.tellg(); inFile.seekg(0, std::ios::beg); // 读取图像数据 std::vector<unsigned char> imageData(fileSize); inFile.read((char*) &imageData[0], fileSize); // 关闭文件 inFile.close(); // 在控制台中显示图像数据 for (unsigned char pixel : imageData) { std::cout << (int)pixel << " "; } return 0; }
This will print the value of each pixel in the image file.
The above is the detailed content of How to read and write binary files using C++?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


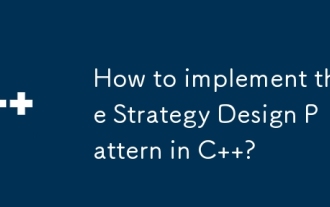
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
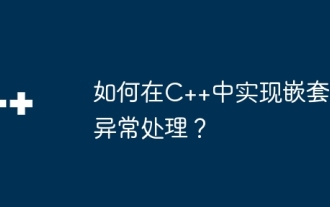
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
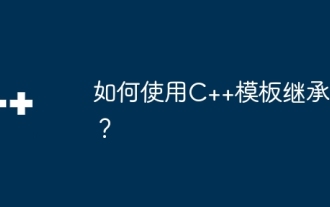
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
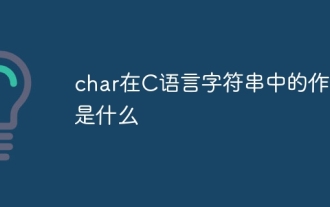
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
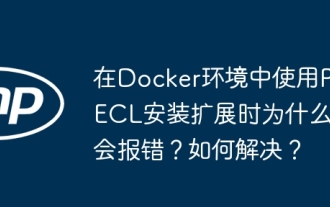
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
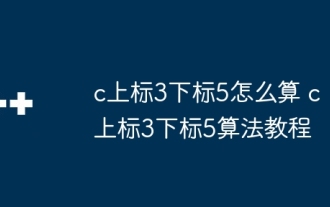
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
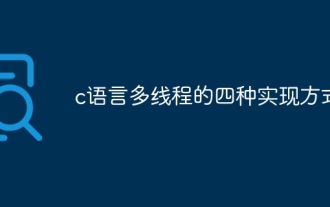
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
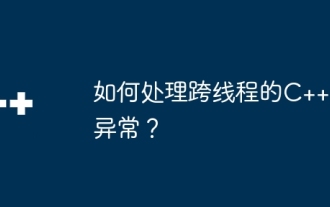
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
