How to choose the appropriate data structure in C++ class design?
When choosing a data structure in a C class design, the following points should be considered: Determine the data type Consider the data relationship Evaluate the access mode Weigh the performance and space cost
Guidelines for selecting data structures in C class design
Selecting the appropriate data structure in C class design is crucial because it affects performance, memory usage, and code maintenance. Here are some guidelines for choosing an appropriate data structure:
1. Determine the data type
Understanding the type of data you want to store is crucial to choosing an appropriate data structure. Common data types include integers, floating point numbers, strings, and objects.
2. Consider data relationships
Data relationships determine how the data structure is organized. For example, if the data is arranged in sequence, use a linear data structure (such as an array or linked list); if the data is tree-structured, use a tree-like data structure (such as a binary tree or a red-black tree).
3. Evaluate access patterns
Considering the pattern of accessing data is also important for selecting data structures. For example, if the data is frequently accessed in random order, a hash table is more suitable; if the data is only accessed sequentially, an array is more efficient.
4. Trade-off performance and space cost
Different data structures have different performance and space cost characteristics. For example, arrays are very efficient in accessing and inserting, but use more space; linked lists are very efficient in inserting, but access is slow.
Practical case:
Problem: Store a series of student scores. These scores need to be accessed and inserted quickly in ascending order.
Solution: Use a sorted array. Arrays provide fast access (O(1)) and enable fast insertion via binary search (O(log n)).
Code Example:
class Student { public: int score; ... // 其他属性 }; class StudentList { public: Student* arr; int size; // 在数组中查找给定分数的学生 int find(int score) { ... // 二分搜索实现 } // 将学生插入数组并按升序排序 void insert(Student student) { ... // 插入和排序算法实现 } };
By following these guidelines and weighing them against your specific requirements, you can improve performance by selecting appropriate data structures for your C classes , optimize memory usage and simplify code maintenance.
The above is the detailed content of How to choose the appropriate data structure in C++ class design?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


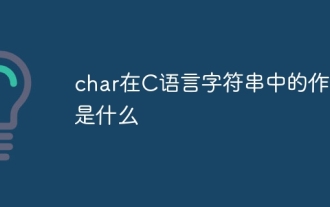
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
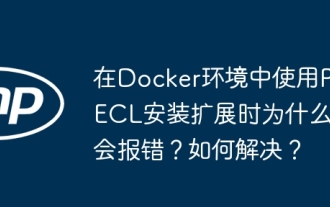
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
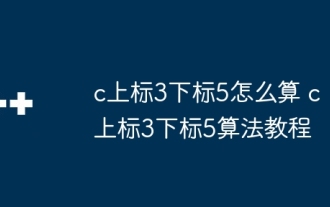
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
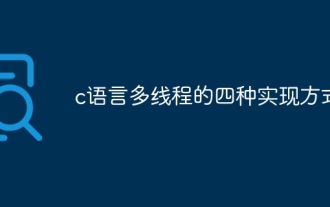
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
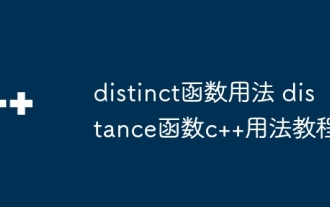
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
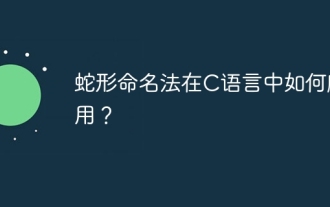
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
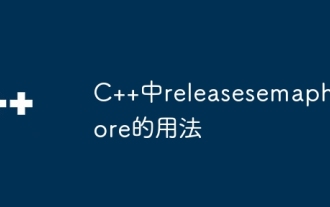
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
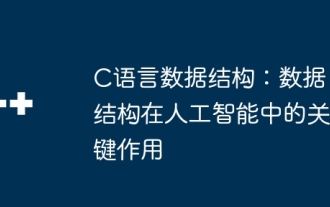
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
