Practical cases of golang framework in game development
Practical cases of Go framework in game development: Technology stack: Go v1.18, Gin framework, MongoDB architecture: Web server (processing HTTP requests), game server (processing game logic and communication), MongoDB database (storing players Data) Web server: Use Gin routing to handle player creation and acquisition requests Game server: Handle game logic and player communication, use UNIX sockets for network communication Database: Use MongoDB to store player data, provide functions for creating and obtaining player information Practical cases Functions: Create players, obtain players, update player status, handle player interactions Conclusion: The Go framework provides efficient
Practical cases of the Go framework in game development
Go has received widespread attention in the field of game development due to its excellent concurrency and high-performance features. The following is a practical case of using the Go framework to build a multi-player game server:
Technology Stack
- Go v1.18
- Gin Web Framework
- MongoDB database
Architecture
The game server architecture is as follows:
+----------------+ | Web 服务器 | +----------------+ | v +----------------+ | 游戏服务器 | +----------------+ | v +----------------+ | MongoDB 数据库 | +----------------+
Code snippet
Web Server
Gin routing for handling HTTP requests:
func main() { router := gin.Default() router.POST("/create-player", createPlayer) router.GET("/get-player", getPlayer) router.Run() } func createPlayer(c *gin.Context) { // 处理创建玩家请求 }func getPlayer(c *gin.Context) { // 处理获取玩家请求 }
Game Server
Game Server Handle game logic and player communication:
func main() { // 创建游戏世界 world := game.NewWorld() // 创建网络服务器 server := net.ListenUnix("unix", &net.UnixAddr{Name: "/tmp/game.sock", Net: "unix"}) for { conn, err := server.Accept() if err != nil { log.Fatal(err) } go handleConn(conn, world) } } func handleConn(conn net.Conn, world *game.World) { // 处理玩家连接并传递消息 }
Database
Use MongoDB to store player data:
type Player struct { ID string `bson:"_id"` Name string Level int } func createPlayer(player *Player) error { _, err := collection.InsertOne(ctx, player) return err } func getPlayer(id string) (*Player, error) { var player Player err := collection.FindOne(ctx, bson.M{"_id": id}).Decode(&player) return &player, err }
Practical case
This game server provides the following functions:
- Create and obtain players
- Update player status in the game
- Handle interactions between players
Conclusion
The Go framework can simplify the game development process by providing efficient and scalable solutions. This practical example shows how to build a multiplayer game server using Go, which can be used as a starting point for building more complex games.
The above is the detailed content of Practical cases of golang framework in game development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


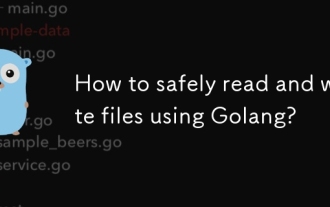
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
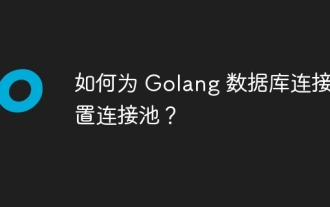
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
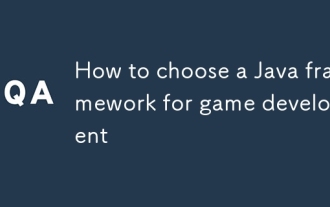
When choosing a Java framework in game development, you should consider the specific needs of your project. Available Java game frameworks include: LibGDX: suitable for cross-platform 2D/3D games. JMonkeyEngine: used to build complex 3D games. Slick2D: Suitable for lightweight 2D games. AndEngine: A 2D game engine developed specifically for Android. Kryonet: Provides network connection capabilities. For 2DRPG games, for example, LibGDX is ideal because of its cross-platform support, lightweight design, and active community.
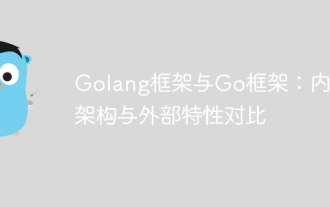
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
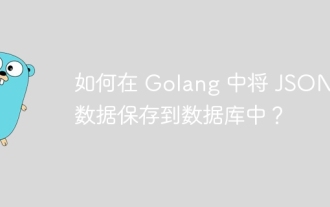
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
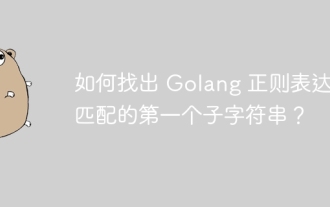
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
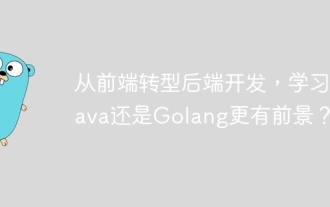
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
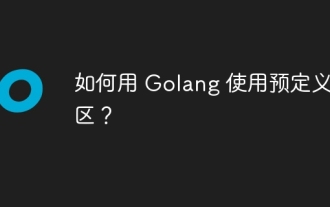
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
