


The application of golang framework extension in distributed systems
Introduction to the application of Go framework extensions in distributed systems: Extension type: Middleware: Perform custom operations in the request life cycle Provider: Provide support for services (such as databases, caches, message queues) Practical case: Create Middleware extension records request data creation provider extension manages Redis database and Kafka message queue interaction code example: middleware extension: func RequestLoggerMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { ... }) }Redis provider extension:
Application of Go framework extension in distributed systems
Introduction
Extensions are a powerful feature in the Go framework, which allow developers to extend the framework to meet specific needs or integrate additional functionality. In distributed systems, extensions are particularly useful because they allow you to add custom logic in different components of the system.
Extension types
There are two main types of extensions in the Go framework:
- Middleware: Yes An extension inserted during the request lifecycle that allows custom actions to be performed.
- Provider: Extensions used to provide services, such as database connections, caching, or message queues.
Practical case:
Suppose you need to deploy Go-based microservices in a distributed system that uses Redis cache and Kafka message queue. You can simplify the process by using extensions in the following ways:
Middleware Extension:
- Create a middleware extension to catch all incoming requests and log them Request data.
- In the middleware, you can access the request object and extract the required information.
Provider extension:
- Create a Redis provider extension to manage interaction with the Redis database.
- In providers, you can configure Redis connection information and provide convenience methods to perform common Redis operations.
- Create a similar provider extension for Kafka to manage message production and consumption.
Code Example:
// 中间件扩展 func RequestLoggerMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // 在此记录请求数据 fmt.Printf("Received request: %s %s\n", r.Method, r.URL) next.ServeHTTP(w, r) }) } // Redis 提供者扩展 type RedisProvider struct { client *redis.Client } func (p *RedisProvider) Get(key string) (string, error) { return p.client.Get(key).Result() } // Kafka 提供者扩展 type KafkaProvider struct { producer *kafka.Producer consumer *kafka.Consumer } func (p *KafkaProvider) Produce(topic string, key string, value string) error { return p.producer.Produce(&kafka.Message{ TopicPartition: kafka.TopicPartition{Topic: topic}, Key: []byte(key), Value: []byte(value), }) }
Conclusion
By using extensions, you can scale in a distributed system Go framework features. Middleware extensions allow you to enhance request handling, while provider extensions simplify interaction with external services. With this approach, you can create highly customizable and scalable distributed applications.
The above is the detailed content of The application of golang framework extension in distributed systems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


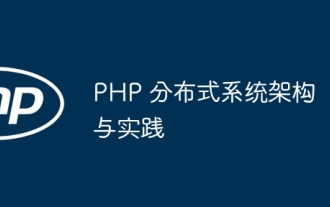
PHP distributed system architecture achieves scalability, performance, and fault tolerance by distributing different components across network-connected machines. The architecture includes application servers, message queues, databases, caches, and load balancers. The steps for migrating PHP applications to a distributed architecture include: Identifying service boundaries Selecting a message queue system Adopting a microservices framework Deployment to container management Service discovery
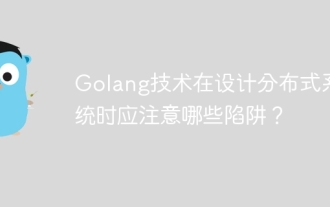
Pitfalls in Go Language When Designing Distributed Systems Go is a popular language used for developing distributed systems. However, there are some pitfalls to be aware of when using Go, which can undermine the robustness, performance, and correctness of your system. This article will explore some common pitfalls and provide practical examples on how to avoid them. 1. Overuse of concurrency Go is a concurrency language that encourages developers to use goroutines to increase parallelism. However, excessive use of concurrency can lead to system instability because too many goroutines compete for resources and cause context switching overhead. Practical case: Excessive use of concurrency leads to service response delays and resource competition, which manifests as high CPU utilization and high garbage collection overhead.
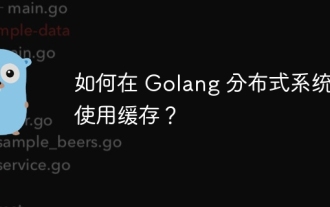
In the Go distributed system, caching can be implemented using the groupcache package. This package provides a general caching interface and supports multiple caching strategies, such as LRU, LFU, ARC and FIFO. Leveraging groupcache can significantly improve application performance, reduce backend load, and enhance system reliability. The specific implementation method is as follows: Import the necessary packages, set the cache pool size, define the cache pool, set the cache expiration time, set the number of concurrent value requests, and process the value request results.
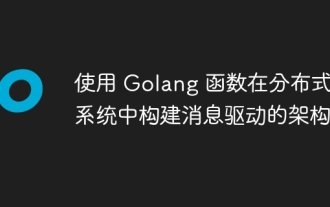
Building a message-driven architecture using Golang functions includes the following steps: creating an event source and generating events. Select a message queue for storing and forwarding events. Deploy a Go function as a subscriber to subscribe to and process events from the message queue.
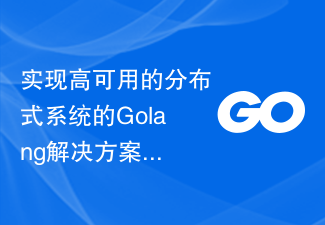
Golang is an efficient, concise, and safe programming language that can help developers implement highly available distributed systems. In this article, we will explore how Golang implements highly available distributed systems and provide some specific code examples. Challenges of Distributed Systems A distributed system is a system in which multiple participants collaborate. Participants in a distributed system may be different nodes distributed in multiple aspects such as geographical location, network, and organizational structure. When implementing a distributed system, there are many challenges that need to be addressed, such as:
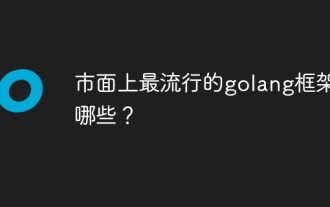
The most popular Go frameworks at present are: Gin: lightweight, high-performance web framework, simple and easy to use. Echo: A fast, highly customizable web framework that provides high-performance routing and middleware. GorillaMux: A fast and flexible multiplexer that provides advanced routing configuration options. Fiber: A performance-optimized, high-performance web framework that handles high concurrent requests. Martini: A modular web framework with object-oriented design that provides a rich feature set.
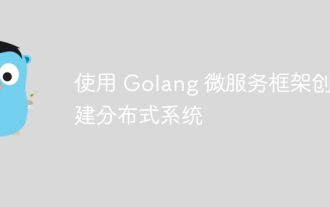
Create a distributed system using the Golang microservices framework: Install Golang, choose a microservices framework (such as Gin), create a Gin microservice, add endpoints to deploy the microservice, build and run the application, create an order and inventory microservice, use the endpoint to process orders and inventory Use messaging systems such as Kafka to connect microservices Use the sarama library to produce and consume order information
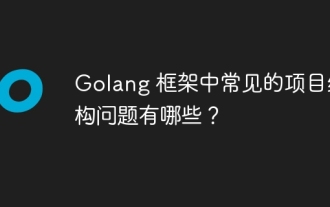
Common structural problems in Go projects include: Lack of layering: Solution: Adopt a vertical layered structure and use interfaces to achieve loose coupling. Excessive nesting: Solution: Reduce the nesting depth and use functions or structures to encapsulate complex logic. Lack of modularity: Solution: Break the code into manageable modules and use package and dependency management tools. Routing multi-level directories: Solution: Use a clear directory structure and avoid directories with too many dependencies. Lack of automated testing: Solution: Modularize test logic and use automated testing frameworks.
