


How to achieve distributed performance optimization in Golang technology performance optimization?
How to implement Golang distributed performance optimization? Concurrent programming: Use Goroutines to execute tasks in parallel. Distributed locks: Use mutex locks to prevent data inconsistency caused by concurrent operations. Distributed caching: Use Memcached to reduce access to slow storage. Message queue: Use Kafka to decouple task parallel processing. Database sharding: Horizontally split data into multiple servers to reduce the load on a single server.
Golang Technology Performance Optimization: Distributed Performance Optimization
Distributed systems are favored for their scalability and elasticity , but also brings a new set of performance challenges. Achieving distributed performance optimization in Golang technology is particularly important because it involves optimization of parallelism and distributed data management. This article will introduce several common techniques for achieving distributed performance optimization in Golang, and illustrate them with practical cases.
1. Concurrent programming
-
goroutine: Goroutine is a lightweight thread used to perform concurrent tasks in Golang . Using goroutine, tasks can be executed in parallel to improve performance.
func main() { var wg sync.WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go func(i int) { // 并发执行任务 defer wg.Done() }(i) } wg.Wait() }
Copy after login
2. Distributed lock
Mutex lock: In distributed system , a mechanism is needed to ensure exclusive access to shared resources. Distributed locks use mutex locks to achieve this, preventing concurrent operations from causing data inconsistency.
import ( "sync" "time" ) // 用于分布式锁的互斥锁 var mutex sync.Mutex func main() { // 获取锁 mutex.Lock() defer mutex.Unlock() // 对共享资源进行独占操作 }
Copy after login
3. Distributed cache
Memcached: Memcached is a distributed cache In-memory object cache system for storing frequently accessed data. By using Memcached, you can improve performance by reducing the number of accesses to the database or other slow backend storage.
import ( "github.com/bradfitz/gomemcache/memcache" ) func main() { // 创建 Memcached 客户端 client, err := memcache.New("localhost:11211") if err != nil { // 处理错误 } // 设置缓存项 err = client.Set(&memcache.Item{ Key: "key", Value: []byte("value"), }) if err != nil { // 处理错误 } // 获取缓存项 item, err := client.Get("key") if err != nil { // 处理错误 } // 使用缓存项 }
Copy after login
4. Message queue
Kafka: Kafka is a distributed message Queues for reliably transmitting large amounts of data. With Kafka, tasks can be decoupled into independent processes and processed in parallel, thereby improving performance.
import ( "github.com/Shopify/sarama" ) func main() { // 创建 Kafka 消费者 consumer, err := sarama.NewConsumer([]string{"localhost:9092"}, nil) if err != nil { // 处理错误 } // 消费消息 messages, err := consumer.Consume([]string{"topic"}, nil) if err != nil { // 处理错误 } for { msg := <-messages // 处理消息 } }</code> **5. 数据库分片**
Copy after loginHorizontal sharding: Horizontal sharding horizontally splits the data in the database table across multiple servers, thereby reducing the load on a single server. This is especially useful for processing large amounts of data.
CREATE TABLE users ( id INT NOT NULL AUTO_INCREMENT, name VARCHAR(255) NOT NULL, PRIMARY KEY (id) ) PARTITION BY HASH (id) PARTITIONS 4;
Copy after loginPractical case: Cache parallel query
In a mall system, the homepage will display basic information of multiple products. The traditional query method is to query product information one at a time from the database, which is inefficient. Using concurrent queries and caching can significantly improve performance.
func main() { // 从缓存中获取产品信息 products := getProductsFromCache() // 并发查询数据库获取缺失的产品信息 var wg sync.WaitGroup for _, p := range products { if p.Info == nil { wg.Add(1) go func(p *product) { defer wg.Done() // 从数据库查询产品信息 p.Info = getProductInfoFromDB(p.ID) // 更新缓存 setCache(p.ID, p.Info) }(p) } } wg.Wait()
Copy after login
The above is the detailed content of How to achieve distributed performance optimization in Golang technology performance optimization?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


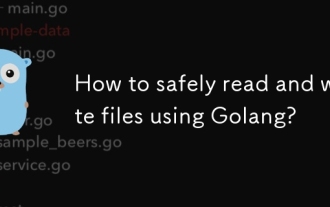
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
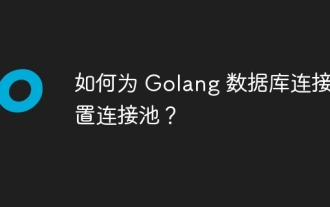
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
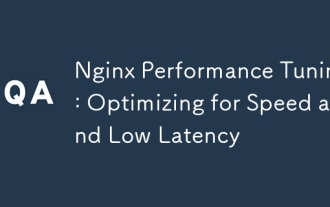
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
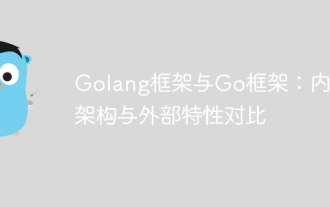
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
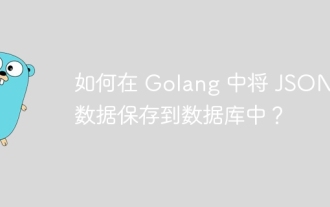
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
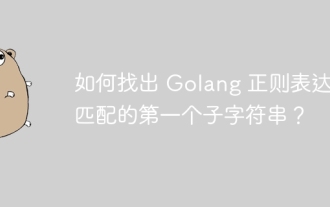
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
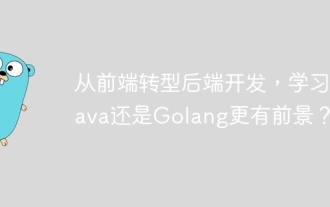
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
