


How to effectively handle error scenarios in C++ through exception handling?
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Taking file opening error handling as an example, when the program fails to open the file, it will throw an exception and print the error message and return the error code through the catch block to handle the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
Efficient handling of error scenarios in C++ through exception handling
Exception handling is a powerful mechanism that allows programs to handle errors gracefully and maintain code integrity sex. In C++, exceptions are handled through try-catch
blocks:
try { // 可能会引发异常的代码 } catch (exception& e) { // 处理异常 }
Common exception types
The C++ standard library defines multiple exception types:
runtime_error
: Runtime error, such as memory allocation failurelogic_error
: Logic error, such as invalid parameterinvalid_argument
: Invalid function parameterout_of_range
: Index or value out of bounds
Practical case: File opening error handling
Consider one Program, which attempts to open a file:
#include <fstream> #include <iostream> using namespace std; int main() { ifstream infile; try { infile.open("data.txt"); if (!infile.is_open()) throw runtime_error("无法打开文件!"); } catch (const runtime_error& e) { cerr << "错误:" << e.what() << endl; return -1; // 返回错误代码 } // 使用文件 infile.close(); return 0; }
When a program fails to open a file, it throws a runtime_error
exception and handles it via a catch
block. This block prints an error message and returns an error code. This allows the program to handle errors gracefully without unexpected termination.
Advantages
Exception handling provides the following advantages:
-
Centralized location for error handling: Confines error handling code to
catch
block to make it easier to maintain. - Error propagation: Exceptions can be passed to the calling function, allowing higher-level code to handle the error.
- Code Robustness: Exception handling helps write robust code that handles errors gracefully.
The above is the detailed content of How to effectively handle error scenarios in C++ through exception handling?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
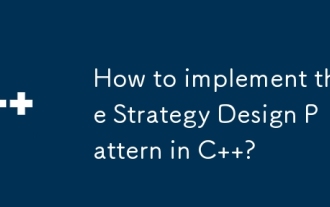
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
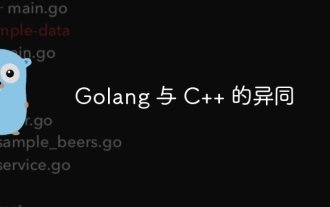
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
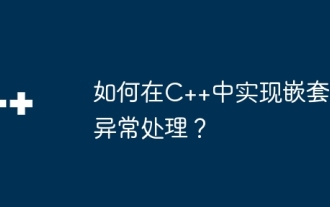
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
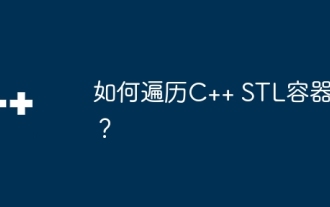
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
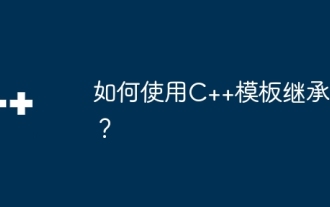
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
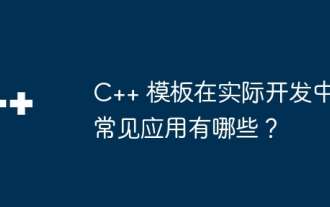
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
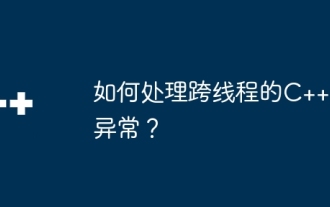
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
