What are the precautions for using the golang framework?
You need to pay attention to the following when using the Go framework: Dependency management: Use the go mod command to manage dependencies, update dependencies regularly, and consider using automated tools. Routing and middleware: Follow consistent routing conventions, use middleware for common tasks, and consider third-party routers for more advanced functionality. Data persistence: Choose the right database, use an ORM framework to simplify data manipulation, and adhere to data persistence best practices. Configuration management: Store configurations in separate files, use environment variables to override configurations, and consider using configuration file management tools. Error handling: Provide clear error messages, return correct HTTP status codes, and consider using error tracking tools. Security Considerations: Using Secure HTTP Markers
# Considerations and Best Practices for the Go Framework
The Go Framework is a powerful toolbox for building Complex and scalable web applications. However, when using the framework, it is necessary to know some considerations and best practices to ensure the high quality and reliability of the application.
Dependency Management
The Go framework depends on multiple third-party libraries and packages. It is crucial to ensure that these dependencies are up to date and compatible with the version of the application. The following best practices can be used:
- Use the
go mod
command to manage dependencies. - Run the
go mod tidy
command regularly to update dependencies. - Automate dependency management using a tool like GoReleaser.
Routing and Middleware
Routing and middleware are key components in the Go framework for controlling the flow of application requests. Here are considerations that shouldn't be ignored:
- Follow consistent routing conventions, such as using the
/api
prefix for API endpoints. - Use middleware for common tasks such as authentication, authorization, and logging.
- Consider using a third-party router such as gorilla/mux for more advanced functionality.
Data persistence
The Go framework supports the use of various databases for data persistence. Here are the best practices:
- Choose a database that matches your application's needs.
- Use an ORM (Object Relational Mapping) framework such as GORM to simplify data manipulation.
- Adhere to best practices for data persistence, such as using transactions and avoiding SQL injection.
Configuration Management
The Go framework uses configurations to customize the behavior of the application. The following are considerations that should not be ignored:
- Store configuration in a separate file or package.
- Use environment variables or command line parameters to override the default configuration.
- Consider using a profile management tool such as Viper to simplify handling of profiles.
Error handling
Error handling is crucial in applications built with the Go framework. Here are the best practices:
- Use clear and concise error messages.
- Return the correct HTTP status code to indicate errors.
- Consider using a third-party bug tracking tool such as Sentry or Logrus.
Security Considerations
The Go framework provides built-in security features, but it is also important to implement additional security measures. Here are some considerations:
- Use secure HTTP headers (such as
X-XSS-Protection
) to prevent cross-site scripting attacks. - Enable CSRF protection to prevent cross-site request forgery.
- Regularly update the framework and dependencies to patch security vulnerabilities.
Practical case
Use GORM to manage database data
Use GORM ORM framework to manage database data:
package main import ( "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" ) type User struct { ID uint `gorm:"primaryKey"` Name string `gorm:"size:255"` Email string `gorm:"unique"` Password string `gorm:"size:255"` } func main() { db, err := gorm.Open(mysql.Open("user:password@tcp(127.0.0.1:3306)/database"), &gorm.Config{}) if err != nil { panic(err) } user := User{Name: "John Doe", Email: "john.doe@example.com", Password: "secret"} db.Create(&user) fmt.Println("User created:", user) }
The above is the detailed content of What are the precautions for using the golang framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
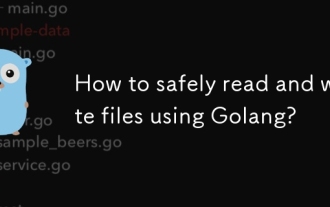
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
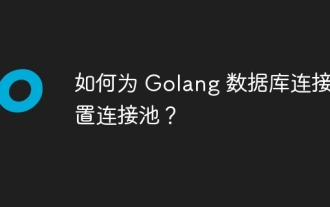
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
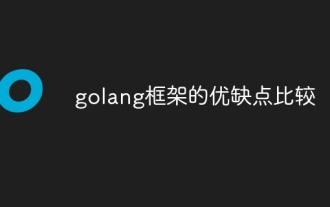
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
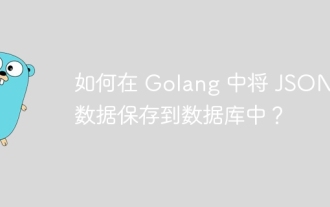
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
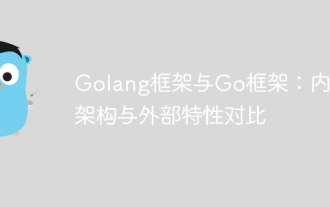
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
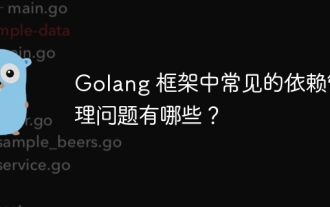
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
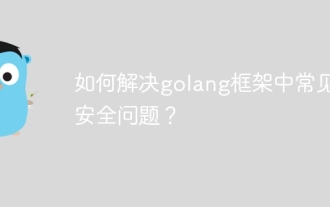
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
