Profiling and performance analysis of Golang functions
Question: How to optimize performance in Go language? Profiling: Use built-in tools to generate code execution information (CPU, memory, etc.). Analyze profiling results: Use the pprof tool to visually analyze the profiling file and find the performance bottleneck function. Benchmarking: Compare the performance of different implementations and understand the optimization effect. Practical case: Find server bottlenecks through profiling analysis and optimize loops to improve performance. Recommended tools: In addition to built-in tools, there are also third-party tools such as go-torch, pprof, go-perf, etc. to assist in performance optimization.
Profiling and performance analysis of Go functions
The profiling and performance analysis tools in the Go language are very powerful and can help you easily find out the problems in the Go code. Performance bottleneck. This article will introduce the use of profiling and performance analysis in the Go language.
Profiling
The Go language has a built-in profiling tool that can generate various information when the code is executed, including:
func main() { f := func() { // 占用 CPU 时间的代码 } // 开始 profiling prof := pprof.StartCPUProfile(os.Stderr) defer prof.Stop() // 运行函数 f() }
You can use the following command to generate a CPU profiling file:
go run main.go > prof.out
Analyze profiling results
You can use the pprof
tool to analyze the profiling file:
pprof -web prof.out
This will open an interactive interface in the browser , display profiling results. You can drill down to the function level to see which functions are taking up the most time.
Benchmarking
In addition to profiling, the Go language also provides benchmarking tools for comparing the performance of different implementations.
func BenchmarkMyFunction(b *testing.B) { for i := 0; i < b.N; i++ { f() } }
You can run the benchmark using the following command:
go test -v -bench=.
Practical case
In the following example, we create a simple Go server that contains a performance bottleneck. Using the profiling tool, we can easily find out where the bottleneck is:
func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } func handler(w http.ResponseWriter, r *http.Request) { for i := 0; i < 10000000; i++ { // 占用 CPU 时间的代码 } w.Write([]byte("Hello, world!")) }
Using the pprof
tool to analyze the profiling file, we find that the loops in the handler
function occupy most of the time. We can improve server performance by optimizing loops.
Performance Tool Recommendation
In addition to the built-in tools, there are many third-party tools that can help you profile and perform performance analysis of Go code, such as:
- [go -torch](https://github.com/uber-go/go-torch)
- [pprof](https://github.com/google/pprof)
- [go -perf](https://github.com/maruel/go-perf)
The above is the detailed content of Profiling and performance analysis of Golang functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


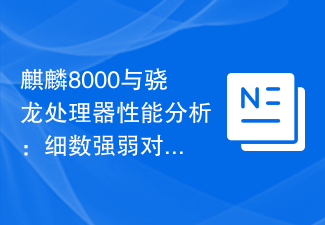
Kirin 8000 and Snapdragon processor performance analysis: detailed comparison of strengths and weaknesses. With the popularity of smartphones and their increasing functionality, processors, as the core components of mobile phones, have also attracted much attention. One of the most common and excellent processor brands currently on the market is Huawei's Kirin series and Qualcomm's Snapdragon series. This article will focus on the performance analysis of Kirin 8000 and Snapdragon processors, and explore the comparison of the strengths and weaknesses of the two in various aspects. First, let’s take a look at the Kirin 8000 processor. As Huawei’s latest flagship processor, Kirin 8000
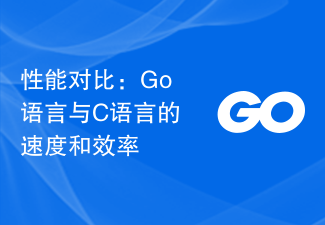
Performance comparison: speed and efficiency of Go language and C language In the field of computer programming, performance has always been an important indicator that developers pay attention to. When choosing a programming language, developers usually focus on its speed and efficiency. Go language and C language, as two popular programming languages, are widely used for system-level programming and high-performance applications. This article will compare the performance of Go language and C language in terms of speed and efficiency, and demonstrate the differences between them through specific code examples. First, let's take a look at the overview of Go language and C language. Go language is developed by G
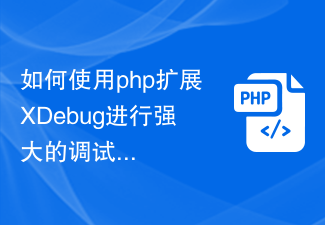
How to use the PHP extension Xdebug for powerful debugging and performance analysis Introduction: In the process of developing PHP applications, debugging and performance analysis are essential links. Xdebug is a powerful debugging tool commonly used by PHP developers. It provides a series of advanced functions, such as breakpoint debugging, variable tracking, performance analysis, etc. This article will introduce how to use Xdebug for powerful debugging and performance analysis, as well as some practical tips and precautions. 1. Install Xdebug and start using Xdebu
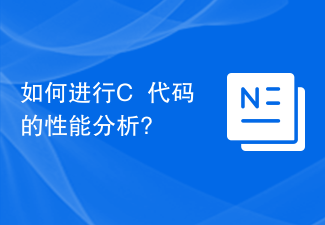
How to perform performance analysis of C++ code? Performance is an important consideration when developing C++ programs. Optimizing the performance of your code can improve the speed and efficiency of your program. However, to optimize your code, you first need to understand where its performance bottlenecks are. To find the performance bottleneck, you first need to perform code performance analysis. This article will introduce some commonly used C++ code performance analysis tools and techniques to help developers find performance bottlenecks in the code for optimization. Profiling tool using Profiling tool
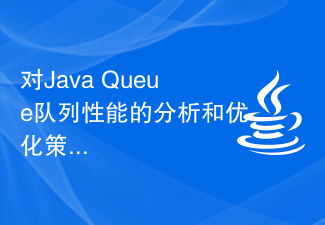
Performance Analysis and Optimization Strategy of JavaQueue Queue Summary: Queue (Queue) is one of the commonly used data structures in Java and is widely used in various scenarios. This article will discuss the performance issues of JavaQueue queues from two aspects: performance analysis and optimization strategies, and give specific code examples. Introduction Queue is a first-in-first-out (FIFO) data structure that can be used to implement producer-consumer mode, thread pool task queue and other scenarios. Java provides a variety of queue implementations, such as Arr
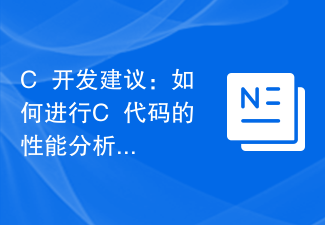
As a C++ developer, performance optimization is one of our inevitable tasks. In order to improve the execution efficiency and response speed of the code, we need to understand the performance analysis methods of C++ code in order to better debug and optimize the code. In this article, we will introduce you to some commonly used C++ code performance analysis tools and techniques. Compilation options The C++ compiler provides some compilation options that can be used to optimize the execution efficiency of the code. Among them, the most commonly used option is -O, which tells the compiler to optimize the code. Normally, we would set
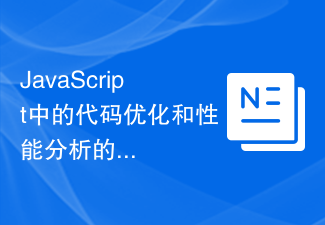
With the rapid development of Internet technology, JavaScript, as a widely used front-end language, is receiving more and more attention. However, when processing large amounts of data or complex logic, JavaScript performance will be affected. In order to solve this problem, we need to master some code optimization and performance analysis tools and techniques. This article will introduce you to some commonly used JavaScript code optimization and performance analysis tools and techniques. 1. Code optimization to avoid global variables: global variables will occupy more
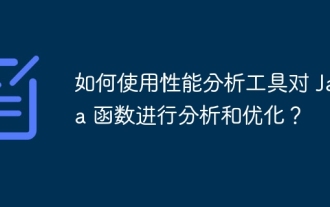
Java performance analysis tools can be used to analyze and optimize the performance of Java functions. Choose performance analysis tools: JVisualVM, VisualVM, JavaFlightRecorder (JFR), etc. Configure performance analysis tools: set sampling rate, enable events. Execute the function and collect data: Execute the function after enabling the profiling tool. Analyze performance data: identify bottleneck indicators such as CPU usage, memory usage, execution time, hot spots, etc. Optimize functions: Use optimization algorithms, refactor code, use caching and other technologies to improve efficiency.
