


What are the advantages and disadvantages of message passing in C++ multi-threaded programming?
Message passing provides the following advantages in C++ multi-threaded programming: 1. Decoupled threads; 2. Synchronous communication; 3. Modularization. But it also has disadvantages: 1. Overhead; 2. Latency; 3. Complexity.
Advantages and Disadvantages of Message Passing in C++ Multithreaded Programming
Introduction
Message passing is a technology that allows communication between threads and is widely used in multi-threaded programming. This article will explore the advantages and disadvantages of message passing in C++ and provide practical examples to illustrate the concept.
Advantages
- Decoupling threads: Message passing decouples threads through message queues so that they do not have to interact directly. This simplifies the code and improves maintainability.
- Synchronous communication: Message passing ensures the synchronization of communication between threads and prevents data races and inconsistencies. By sending a message to a queue, the sending thread waits for the receiving thread to process the message.
- Modularization: Message passing allows the specific functions of threads to be modularized, thus facilitating code reuse and extension.
Disadvantages
- Overhead: Message passing involves the creation and management of message queues, which results in additional overhead and memory consumption.
- Delay: Due to the existence of the message queue, message delivery may introduce a certain degree of delay, especially when the message queue is busy.
- Complexity: The implementation of the message passing mechanism can be challenging and requires careful consideration of locks and synchronization mechanisms.
Practical case
// 创建消息队列 mqd_t queue = mq_open("/my_queue", O_CREAT | O_WRONLY); // 创建线程向队列发送消息 void* sender(void* arg) { while (true) { // 将消息写入队列 mq_send(queue, "Hello", 5, 0); // 休眠 1 秒 sleep(1); } return NULL; } // 创建线程从队列接收消息 void* receiver(void* arg) { char buffer[5]; while (true) { // 从队列读取消息 mq_receive(queue, buffer, 5, NULL); // 处理消息 printf("Received: %s\n", buffer); } return NULL; } int main() { // 创建两个线程 pthread_t sender_thread, receiver_thread; // 启动线程 pthread_create(&sender_thread, NULL, sender, NULL); pthread_create(&receiver_thread, NULL, receiver, NULL); // 等待线程结束 pthread_join(sender_thread, NULL); pthread_join(receiver_thread, NULL); // 关闭消息队列 mq_close(queue); mq_unlink("/my_queue"); return 0; }
In this example, two threads are created: one for sending messages to the message queue, and the other for sending messages from the queue Receive messages. This shows how to implement inter-thread communication using message passing.
The above is the detailed content of What are the advantages and disadvantages of message passing in C++ multi-threaded programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
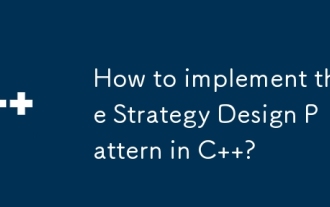
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
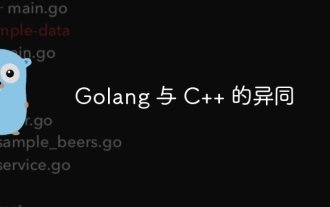
Golang and C++ are garbage collected and manual memory management programming languages respectively, with different syntax and type systems. Golang implements concurrent programming through Goroutine, and C++ implements it through threads. Golang memory management is simple, and C++ has stronger performance. In practical cases, Golang code is simpler and C++ has obvious performance advantages.
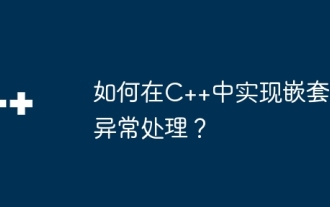
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
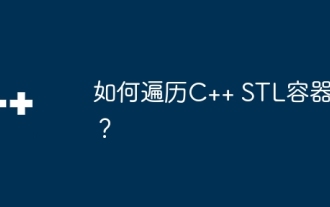
To iterate over an STL container, you can use the container's begin() and end() functions to get the iterator range: Vector: Use a for loop to iterate over the iterator range. Linked list: Use the next() member function to traverse the elements of the linked list. Mapping: Get the key-value iterator and use a for loop to traverse it.
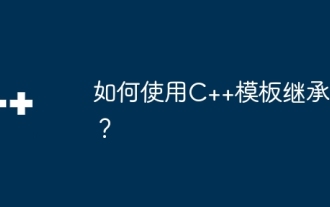
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
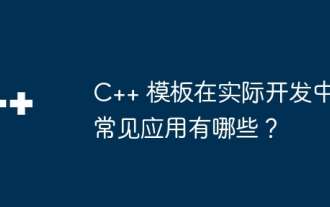
C++ templates are widely used in actual development, including container class templates, algorithm templates, generic function templates and metaprogramming templates. For example, a generic sorting algorithm can sort arrays of different types of data.
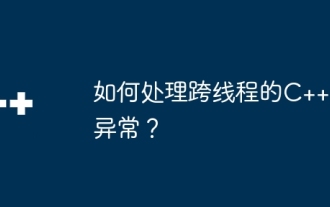
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
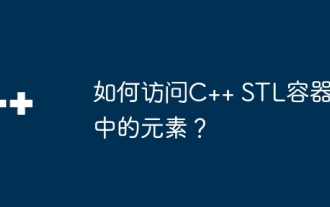
How to access elements in C++ STL container? There are several ways to do this: Traverse a container: Use an iterator Range-based for loop to access specific elements: Use an index (subscript operator []) Use a key (std::map or std::unordered_map)
