How to create and manage files using Golang?
Steps to create and manage files in Go language: Use the os.Create function to create files. Open the file using the os.Open function. Use the File object's WriteString method to write to the file. Use the io.ReadAll function to read files. Use the os.Remove function to delete files.
How to use Go language to create and manage files
Create files
Use os.Create
Function creates a new file:
package main import ( "fmt" "os" ) func main() { f, err := os.Create("test.txt") if err != nil { fmt.Println(err) return } fmt.Println("File created successfully") defer f.Close() }
Open a file
Open an existing file using the os.Open
function :
func main() { f, err := os.Open("test.txt") if err != nil { fmt.Println(err) return } fmt.Println("File opened successfully") defer f.Close() }
Write a file
Use the WriteString
method of the File object to write a file:
func main() { f, err := os.OpenFile("test.txt", os.O_WRONLY, 0644) if err != nil { fmt.Println(err) return } _, err = f.WriteString("Hello, world!") if err != nil { fmt.Println(err) return } fmt.Println("File written successfully") defer f.Close() }
Read a file
Use io.ReadAll
function to read files:
func main() { f, err := os.Open("test.txt") if err != nil { fmt.Println(err) return } data, err := io.ReadAll(f) if err != nil { fmt.Println(err) return } fmt.Println("File read successfully:", string(data)) defer f.Close() }
Delete files
Use os.Remove
Function to delete files:
func main() { err := os.Remove("test.txt") if err != nil { fmt.Println(err) return } fmt.Println("File deleted successfully") }
The above is the detailed content of How to create and manage files using Golang?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


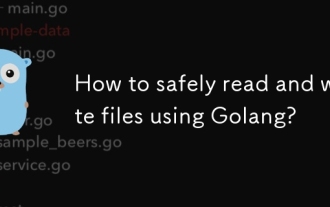
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
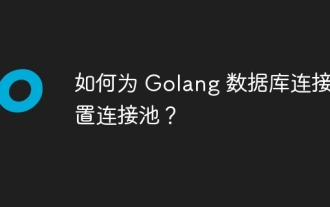
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
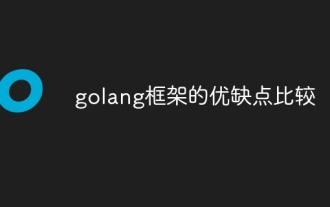
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.
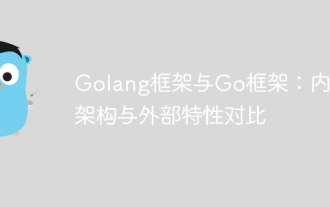
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
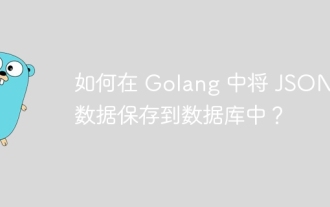
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
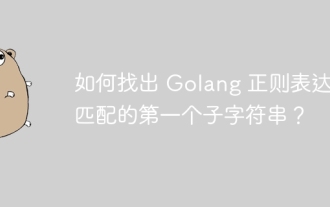
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
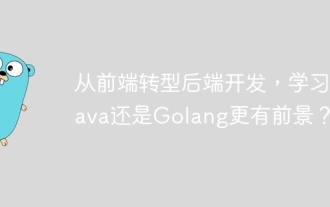
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
