Alternatives to the Go language framework include: Web framework: Echo, GinORM framework: Gorm, XORM Other frameworks: Viper (configuration file loading), Beego (full stack web framework)
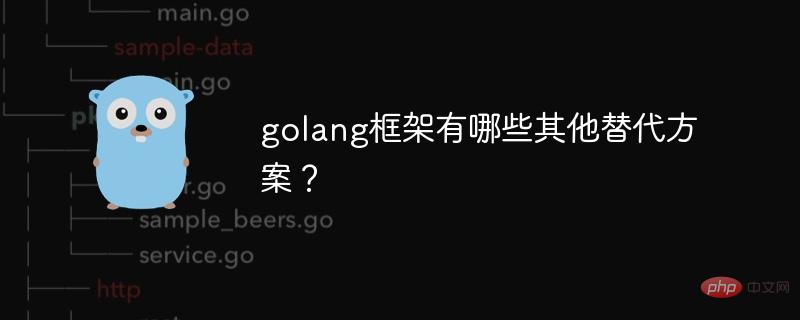
What are the alternatives to the Go language framework?
The Go language is known for its excellent concurrency, memory safety, and efficiency. Its standard library contains many popular frameworks for building various applications. However, there are many third-party frameworks that provide additional features and enhancements.
Web Framework
-
Echo: A lightweight, fast web framework with a focus on scalability and flexibility.
1 2 3 4 5 6 7 8 9 10 11 12 13 | package main
import (
"github.com/labstack/echo"
)
func main() {
e := echo .New()
e.GET( "/" , func(c echo .Context) error {
return c.String(200, "Hello, World!" )
})
e.Logger.Fatal(e.Start( ":8080" ))
}
|
Copy after login
Gin: A high-performance, highly customizable web framework known for its routing system and middleware support.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | package main
import (
"github.com/gin-gonic/gin"
)
func main() {
r := gin.Default()
r.GET( "/" , func(c *gin.Context) {
c.JSON(200, gin.H{
"message" : "Hello, World!" ,
})
})
r.Run()
}
|
Copy after login
ORM framework
##Gorm: A powerful ORM framework that provides various Support for multiple databases and advanced query capabilities.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | package main
import (
"fmt"
"github.com/jinzhu/gorm"
_ "github.com/jinzhu/gorm/dialects/postgres"
)
type User struct {
gorm.Model
Name string
Email string
}
func main() {
db, err := gorm.Open( "postgres" , "user=postgres password=mysecret dbname=mydatabase sslmode=disable" )
if err != nil {
panic(err)
}
defer db.Close()
db.AutoMigrate(&User{})
user := &User{Name: "John Doe" , Email: "johndoe@example.com" }
db.Create(user)
fmt.Println( "User created:" , user)
}
|
Copy after login
XORM: A lightweight, high-performance ORM framework with a powerful query builder and efficient database operations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | package main
import (
"fmt"
"github.com/go-xorm/xorm"
_ "github.com/go-xorm/xorm-sqlite3"
)
type User struct {
Id int64
Name string
Email string
}
func main() {
engine, err := xorm.NewEngine( "sqlite3" , "user.db" )
if err != nil {
panic(err)
}
engine.Sync2( new (User))
user := &User{Name: "Jane Doe" , Email: "janedoe@example.com" }
_, err = engine.Insert(user)
if err != nil {
panic(err)
}
fmt.Println( "User created:" , user)
}
|
Copy after login
Other frameworks
- ##Viper:
A simple configuration file loader that supports Various file formats and dynamic configuration updates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | <strong>package main
import (
"fmt"
"log"
"github.com/spf13/viper"
)
func main() {
viper.SetConfigName( "config" )
viper.SetConfigType( "yaml" )
viper.AddConfigPath( "." )
err := viper.ReadInConfig()
if err != nil {
log.Fatalf( "Error loading config file: %v" , err)
}
fmt.Println( "Port:" , viper.GetInt( "port" ))
fmt.Println( "Database Host:" , viper.GetString( "database.host" ))
}</strong>
|
Copy after login
- Beego:
A full-stack web framework that provides a complete set of features, including ORM, routing, templates, and form validation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <strong>package main
import (
"github.com/beego/beego/v2/core"
"github.com/beego/beego/v2/server/web"
)
func main() {
router := web.NewRouter()
router.GET( "/" , func(ctx *web.Context) {
ctx.WriteString( "Hello, World!" )
})
core.RunWebServer( "" , ":8080" , router)
}</strong>
|
Copy after login
These are just some of the many frameworks available for the Go language. Choosing the right framework for your project depends on your specific needs and preferences.
The above is the detailed content of What are the other alternatives to golang framework?. For more information, please follow other related articles on the PHP Chinese website!