Application of C++ in distributed computing for mobile applications
C Distributed computing in mobile applications improves performance and scalability. Key technology stacks include CUDA, MPI, and OpenMP. In the example, image processing tasks are decomposed and executed in parallel on multi-core CPUs or GPUs via CUDA.
C Distributed Computing in Mobile Applications
Introduction
Distribution Formula computing involves breaking down computing tasks into smaller parts and assigning them to multiple devices or cores for parallel execution. In mobile applications, distributed computing can significantly improve performance and scalability. C is ideal for implementing distributed computing in mobile applications due to its high performance and low overhead.
Technology stack
The following lists the key technology stacks required for distributed computing in C:
- CUDA (Compute Unified Device Architecture): For parallel computing on NVIDIA GPUs.
- MPI (Message Passing Interface): Used for communication and data exchange between different devices or nodes.
- OpenMP: Used to manage threads in shared memory parallel systems.
Practical Case
Consider a mobile image processing application that needs to process large amounts of image data. To improve performance, we can use distributed computing to break image processing tasks into smaller parts and then execute them in parallel on a multi-core CPU or GPU.
The following is a code example to implement this distributed computing scheme using C and CUDA:
// 头文件 #include <cuda.h> #include <cuda_runtime.h> // 设备函数 __global__ void processImage(unsigned char* imageData) { // 图像处理代码 } int main() { // 从设备分配内存 unsigned char* devImageData; cudaMalloc(&devImageData, sizeof(unsigned char) * width * height); // 将图像数据复制到设备 cudaMemcpy(devImageData, imageData, sizeof(unsigned char) * width * height, cudaMemcpyHostToDevice); // 调用设备函数 processImage<<<blocksPerGrid, threadsPerBlock>>>(devImageData); // 从设备复制回图像数据 cudaMemcpy(imageData, devImageData, sizeof(unsigned char) * width * height, cudaMemcpyDeviceToHost); // 释放设备内存 cudaFree(devImageData); return 0; }
Conclusion
Through this article, we introduced the role of C in Distributed computing in mobile applications, and provides practical examples of using CUDA. C enables mobile applications to significantly improve performance and scalability by distributing computing tasks across multiple devices or cores.
The above is the detailed content of Application of C++ in distributed computing for mobile applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
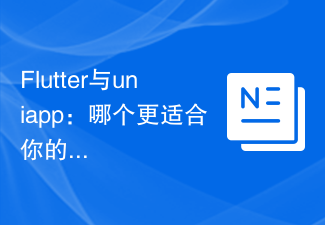
Today, mobile application development has become a key area of concern for more and more companies and individuals. For developers, it is crucial to choose a development framework that suits their needs. Among the many optional development frameworks, Flutter and uniapp are two that have attracted much attention. This article will compare the advantages and disadvantages of these two frameworks and help readers choose the mobile application development solution that best suits them. First, let’s understand these two frameworks. Flutter is a cross-platform mobile application development framework developed by Google, which uses Dart language
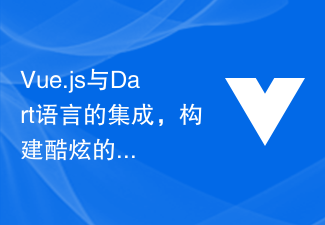
Integration of Vue.js and Dart language, practice and development skills for building cool mobile application UI interfaces Introduction: In mobile application development, the design and implementation of the user interface (UI) is a very important part. In order to achieve a cool mobile application interface, we can integrate Vue.js with the Dart language, and use the powerful data binding and componentization features of Vue.js and the rich mobile application development library of the Dart language to build Stunning mobile application UI interface. This article will show you how to

Mobile Hejiaqin APP is a comprehensive software that integrates family management, intelligent control, and family communication. It aims to create a comfortable, intelligent and harmonious home environment for users through intelligent and convenient operations. Through this application, users can easily control and manage various smart devices at home and enjoy the convenience brought by smart life. So what are the specific functions of the Mobile and Jiaqin App? Users who want to know more about it can follow this article to learn more about it! Tutorial on how to use the Mobile and Jiaqin app: What are the uses of the Mobile and Jiaqin app? Even if you don’t know IT, you can easily manage the network. 2. No matter how many smart products you have, one app is enough. 3. Even if you are thousands of miles away from home, you can still "go home" to watch it. See 4. Rich functions, enjoy smart life
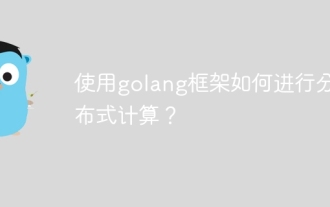
A step-by-step guide to implementing distributed computing with GoLang: Install a distributed computing framework (such as Celery or Luigi) Create a GoLang function that encapsulates task logic Define a task queue Submit a task to the queue Set up a task handler function
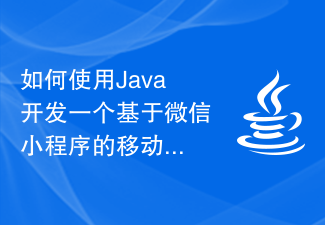
How to use Java to develop a mobile application based on WeChat Mini Program WeChat Mini Program has become a popular choice in the field of mobile application development today, and its convenience and user convenience are favored by developers. Java, as a programming language widely used in mobile application development, can also be used to develop mobile applications based on WeChat applets. This article will introduce how to use Java to develop a mobile application based on WeChat applet and provide specific code examples. 1. Introduction to WeChat Mini Program WeChat Mini Program is an open application platform.
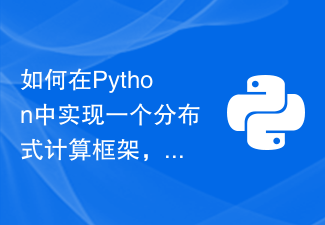
Title: Implementation of distributed computing framework and task scheduling and result collection mechanism in Python Abstract: Distributed computing is a method that effectively utilizes the resources of multiple computers to accelerate task processing. This article will introduce how to use Python to implement a simple distributed computing framework, including the mechanisms and strategies of task scheduling and result collection, and provide relevant code examples. Text: 1. Overview of distributed computing framework Distributed computing is a method that uses multiple computers to jointly process tasks to achieve the purpose of accelerating computing. In a distributed computing framework,
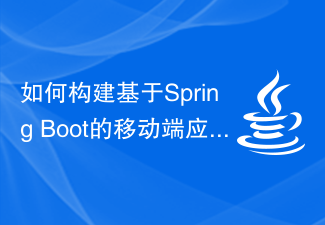
Mobile applications have become an integral part of people's daily lives. When developing mobile applications, it is very important to choose the right development framework. SpringBoot is a rapid development Java framework. Its lightweight and easy-to-use characteristics make it an excellent choice for building mobile applications. This article will introduce in detail how to build a mobile application based on SpringBoot. Environment setup Before starting development, we need to prepare the development environment. Here we choose to use IntelliJ
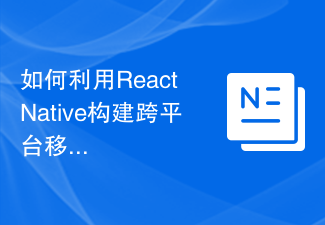
How to use ReactNative to build cross-platform mobile applications Introduction: With the booming mobile application market, developers need to quickly deploy applications to multiple platforms. ReactNative is a powerful tool that helps developers build cross-platform mobile applications using a single code base. This article will introduce the basic concepts of ReactNative and provide some specific code examples to help readers understand how to use ReactNative to build cross-platform mobile applications. 1. Reac
